
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
PinIN.cpp
00001 #include "PinIN.hpp" 00002 //#include "mbed.h" 00003 //#include "LocalPinNames.h" 00004 //#include "BridgeDriver.h" 00005 00006 //Constructor 00007 PinIN::PinIN(LineData lineData){ 00008 this->errorFlag = 0; 00009 00010 //Order of Line: Command, Local_Name, PIN_IN, pinName, pinMode 00011 if (lineData.numWords != 5) 00012 this->errorFlag = 1; 00013 00014 string _pinName = lineData.word[3]; // Local Pin 00015 string _pinMode = lineData.word[4]; // Pin Mode to Select 00016 00017 if(_pinName.compare("DIO0") == 0) 00018 this->pinName = DIO0; 00019 else if(_pinName.compare("DIO1") == 0) 00020 this->pinName = DIO1; 00021 else if(_pinName.compare("DIO2") == 0) 00022 this->pinName = DIO2; 00023 else if(_pinName.compare("DIO3") == 0) 00024 this->pinName = DIO3; 00025 else if(_pinName.compare("DIO4") == 0) 00026 this->pinName = DIO4; 00027 else if(_pinName.compare("DIO5") == 0) 00028 this->pinName = DIO5; 00029 else if(_pinName.compare("DIO6") == 0) 00030 this->pinName = DIO6; 00031 else if(_pinName.compare("DIO7") == 0) 00032 this->pinName = DIO7; 00033 else if(_pinName.compare("DIO8") == 0) 00034 this->pinName = DIO8; 00035 else if(_pinName.compare("DIO9") == 0) 00036 this->pinName = DIO9; 00037 else if(_pinName.compare("DIO10") == 0) 00038 this->pinName = DIO10; 00039 else if(_pinName.compare("DIO11") == 0) 00040 this->pinName = DIO11; 00041 00042 else if(_pinName.compare("AI0") == 0) 00043 this->pinName = AI0; 00044 else if(_pinName.compare("AI1") == 0) 00045 this->pinName = AI1; 00046 else if(_pinName.compare("AI2") == 0) 00047 this->pinName = AI2; 00048 else if(_pinName.compare("AI3") == 0) 00049 this->pinName = AI3; 00050 else if(_pinName.compare("AI4") == 0) 00051 this->pinName = AI4; 00052 else if(_pinName.compare("AI5") == 0) 00053 this->pinName = AI5; 00054 00055 //Pin Name not recognized 00056 else 00057 this->errorFlag = 1; 00058 00059 if(_pinMode.compare("PU") == 0) 00060 this->pinMode = PullUp; 00061 else if(_pinMode.compare("PD") == 0) 00062 this->pinMode = PullDown; 00063 else if(_pinMode.compare("PN") == 0) 00064 this->pinMode = PullNone; 00065 else if(_pinMode.compare("OD") == 0) 00066 this->pinMode = OpenDrain; 00067 00068 //Pin Mode not recognized 00069 else 00070 this->errorFlag = 1; 00071 } 00072 00073 00074 //A line consists of [ __(Local_Name)__ __(function)__ __(parameter1)__ __(parameter2)__ __(parameter3)__ ... and so on] 00075 int PinIN::interpret(LineData &lineData){ 00076 00077 //Initialize the Pin with appropriate values 00078 DigitalIn signal(pinName, pinMode); 00079 00080 //Order of Line: Local_Name, Function_Name, Param1, Param2, Param3,....... 00081 string func = lineData.word[1]; 00082 00083 /******************************************************************************/ 00084 /*** <Func: wait> ***/ 00085 /******************************************************************************/ 00086 if (func.compare("wait") == 0){ 00087 00088 if (lineData.numWords != 3){ 00089 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00090 return -1; 00091 } 00092 00093 //Initialize and Convert Parameters 00094 string pinValue = lineData.word[2]; 00095 int pinValue_Value = 0; 00096 00097 int numValuesFound = sscanf(pinValue.c_str(), "%d", &pinValue_Value); 00098 if (numValuesFound < 1){ 00099 ErrorOut("Parameter Unknown, pin value can't be converted", lineData.lineNumber); 00100 return -1; 00101 } 00102 00103 if(pinValue_Value < 0 && pinValue_Value > 1){ 00104 ErrorOut("Pin Value must be either 0 or 1", lineData.lineNumber); 00105 return -1; 00106 } 00107 00108 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00109 if (DummyMode) 00110 return 0; //Function operated successfully but doesn't return a value 00111 00112 //Wait on the signal to turn to the specified value 00113 while(signal.read() != pinValue_Value); 00114 } 00115 00116 00117 /******************************************************************************/ 00118 /*** <Func: val> ***/ 00119 /******************************************************************************/ 00120 // returns 1 if the check value is equal to the current check value of the Pin 00121 else if (func.compare("val") == 0){ 00122 00123 if (lineData.numWords != 3){ 00124 ErrorOut("Incorrect number of parameters", lineData.lineNumber); 00125 return -1; 00126 } 00127 00128 //Initialize and Convert Parameters 00129 string checkValue = lineData.word[2]; 00130 int checkValue_Value = 0; 00131 int numValuesFound = sscanf(checkValue.c_str(), "%d", &checkValue_Value); 00132 if (numValuesFound < 1){ 00133 ErrorOut("Parameter Unknown, pin value can't be converted", lineData.lineNumber); 00134 return -1; 00135 } 00136 00137 if(checkValue_Value < 0 && checkValue_Value > 1){ 00138 ErrorOut("Pin Value must be either 0 or 1", lineData.lineNumber); 00139 return -1; 00140 } 00141 00142 //All syntax checking done by this point, if Dummy then return success in order to check the code, no need to perform functionality 00143 if (DummyMode) 00144 return 0; //Function operated successfully but doesn't return a value 00145 00146 //Return one if value meets the wanted pin value, return 0 if it doesn't 00147 if(signal.read() == checkValue_Value){ 00148 //Debounce check to ensure the value is still correct, if incorrect after debounce time, return 0, since it's a false truth 00149 if (signal.read() == checkValue_Value){ 00150 Timer debounceTimer; 00151 debounceTimer.reset(); 00152 debounceTimer.start(); 00153 while (debounceTimer.read_ms() < 40); 00154 if (signal.read() == checkValue_Value) 00155 return 1; 00156 else 00157 return 0; 00158 } 00159 } 00160 else 00161 return 0; 00162 } 00163 00164 else { 00165 ErrorOut("Unknown Command for PinIn Class", lineData.lineNumber); 00166 return -1; 00167 } 00168 00169 return 0; //Return success as 0 since no condition had to be met 00170 }
Generated on Sun Jul 24 2022 01:49:11 by
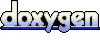