
Component Test's Software to work with "Universal Controller Box" - Software is an interpreter or "compiler" for programs to be done with a .txt file and read off of the SD Card
Dependencies: BridgeDriver FrontPanelButtons MCP23017 SDFileSystem TextLCD mbed
Initialization.hpp
00001 #ifndef INITIALIZATION_HPP 00002 #define INITIALIZATION_HPP 00003 00004 #include "mbed.h" 00005 #include "LocalPinNames.h" 00006 #include "BridgeDriver.h" 00007 #include "FrontPanelButtons.h" 00008 #include "TextLCD.h" 00009 #include "SDFileSystem.h" 00010 #include <string> 00011 #include <vector> 00012 00013 00014 /**********************************************************************************************************************************/ 00015 /**********************************************************************************************************************************/ 00016 /************************** <MUST MODIFY> *************************/ 00017 /**********************************************************************************************************************************/ 00018 /**********************************************************************************************************************************/ 00019 00020 enum DeviceType{MOTOR, VOLTAGE_DRIVER, PIN_IN, TIMER_DEVICE, CAN_DEVICE}; //ADD DEVICE NAME 00021 static const enum DeviceType Device_Map[] = {MOTOR, VOLTAGE_DRIVER, PIN_IN, TIMER_DEVICE, CAN_DEVICE}; //AND HERE ***NOTE TO KEEP SAME ORDER 00022 00023 /************************** <MUST MODIFY> *************************/ 00024 /**********************************************************************************************************************************/ 00025 /**********************************************************************************************************************************/ 00026 00027 00028 00029 /******************************************************************************/ 00030 /*** <Global Initializations> ***/ 00031 /******************************************************************************/ 00032 00033 //Initializations 00034 extern Timer timer; // general purpose timer 00035 extern I2C i2c; // I2C bus (SDA, SCL) 00036 extern BridgeDriver bridges; // Bridge 00037 extern TextLCD_I2C lcd; // LCD 00038 extern SDFileSystem sd; // the pinout on the mbed LPC1768 00039 00040 extern DigitalIn killSw; 00041 00042 //extern Ticker errorWatcher; 00043 00044 extern const int MAX_LINE_LENGTH; 00045 extern int DummyMode; 00046 extern FILE *selectedFile; 00047 extern int errorFLAG; 00048 00049 /******************************************************************************/ 00050 /*** <Line Data Struct Initializations> ***/ 00051 /******************************************************************************/ 00052 00053 struct LineData{ 00054 00055 int lineNumber; //current line number in the program txt file that is running 00056 string word[50]; //array of words from the line of text, assuming no more than 50 words will be in any given line 00057 //in this initialization there are 15 string (pointers) of size MAX_LINE_LENGTH each 00058 int numWords; //Number of words in the given line 00059 int lineAddress; //current line address in the SD Card 00060 }; 00061 00062 /******************************************************************************/ 00063 /*** <Function Initializations> ***/ 00064 /******************************************************************************/ 00065 00066 void fullInit(); //Perform and call any needed initializations 00067 00068 void initLCD(void); //Initialize the LCD 00069 00070 void ErrorOut(string, int); //Outputs error message, line number, and formatting to LCD 00071 00072 int cyclePrograms(vector<string>, int, int, int); 00073 00074 void resetLineData(LineData &); //reset and all variables of the Line Data Struct 00075 00076 int interpretCommand(LineData &); 00077 00078 int loopCommand(LineData &); 00079 00080 /******************************************************************************/ 00081 /*** <GoTo Label Initializations> ***/ 00082 /******************************************************************************/ 00083 00084 struct GoToLabel{ 00085 00086 string name; //name of the GoTo Label 00087 int lineNumber; //line number of the GoTo Label 00088 int lineAddress; //line address of the GoTo Label 00089 }; 00090 00091 extern vector<GoToLabel> GoToLabels; 00092 00093 00094 /******************************************************************************/ 00095 /*** <Cycle Struct Initializations> ***/ 00096 /******************************************************************************/ 00097 00098 struct CycleWatch{ 00099 00100 int numCycles; //number of cycles to go to 00101 int counter; //number the tracks the current cycle count 00102 float totalCycleTime; //tracks the total amount of time for the cycle, in order to calculate the average cycle time 00103 int startAddress; //starting address to seek back to on loop 00104 int startLineNumber; //starting line number to reset to 00105 }; 00106 00107 /******************************************************************************/ 00108 /*** <Parent Device Class Initializations> ***/ 00109 /******************************************************************************/ 00110 00111 extern const string DeviceNames[]; 00112 extern int numDevices; 00113 extern int currNumDevices; 00114 00115 00116 class Device{ 00117 00118 public: 00119 string name; 00120 int errorFlag; 00121 enum DeviceType type; 00122 static Device* newDevice(int, string, LineData); 00123 virtual int interpret(LineData&) = 0; 00124 virtual int off() = 0; 00125 virtual int pause() = 0; 00126 virtual int resume() = 0; 00127 }; 00128 00129 extern vector<Device*> devices; //Initialize vector of devices 00130 00131 00132 /******************************************************************************/ 00133 /*** <Error Monitor Initializations> ***/ 00134 /******************************************************************************/ 00135 /* 00136 struct ErrorCondition{ 00137 00138 LineData errorToWatch; 00139 LineData errorFix; 00140 int hasFix; 00141 }; 00142 00143 //extern vector<ErrorCondition> errorMonitors; //Initialize vector of errors to monitor 00144 extern ErrorCondition errorMonitors[15]; 00145 extern int numErrorMonitors;*/ 00146 //void ErrorMonitor(); //Monitors the conditions to watch for erroring, and pauses system if any of the conditions turn out to be true 00147 00148 #endif
Generated on Sun Jul 24 2022 01:49:11 by
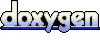