Modification of Mbed-dev library for LQFP48 package microcontrollers: STM32F103C8 (STM32F103C8T6) and STM32F103CB (STM32F103CBT6) (Bluepill boards, Maple mini etc. )
Fork of mbed-STM32F103C8_org by
flash_api.h
00001 /** \addtogroup hal */ 00002 /** @{*/ 00003 00004 /* mbed Microcontroller Library 00005 * Copyright (c) 2017 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 #ifndef MBED_FLASH_API_H 00020 #define MBED_FLASH_API_H 00021 00022 #include "device.h" 00023 #include <stdint.h> 00024 00025 #if DEVICE_FLASH 00026 00027 #define MBED_FLASH_INVALID_SIZE 0xFFFFFFFF 00028 00029 typedef struct flash_s flash_t; 00030 00031 #if TARGET_FLASH_CMSIS_ALGO 00032 #include "flash_data.h" 00033 #endif 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif 00038 00039 /** 00040 * \defgroup flash_hal Flash HAL API 00041 * @{ 00042 */ 00043 00044 /** Initialize the flash peripheral and the flash_t object 00045 * 00046 * @param obj The flash object 00047 * @return 0 for success, -1 for error 00048 */ 00049 int32_t flash_init(flash_t *obj); 00050 00051 /** Uninitialize the flash peripheral and the flash_t object 00052 * 00053 * @param obj The flash object 00054 * @return 0 for success, -1 for error 00055 */ 00056 int32_t flash_free(flash_t *obj); 00057 00058 /** Erase one sector starting at defined address 00059 * 00060 * The address should be at sector boundary. This function does not do any check for address alignments 00061 * @param obj The flash object 00062 * @param address The sector starting address 00063 * @return 0 for success, -1 for error 00064 */ 00065 int32_t flash_erase_sector(flash_t *obj, uint32_t address); 00066 00067 /** Program one page starting at defined address 00068 * 00069 * The page should be at page boundary, should not cross multiple sectors. 00070 * This function does not do any check for address alignments or if size is aligned to a page size. 00071 * @param obj The flash object 00072 * @param address The sector starting address 00073 * @param data The data buffer to be programmed 00074 * @param size The number of bytes to program 00075 * @return 0 for success, -1 for error 00076 */ 00077 int32_t flash_program_page(flash_t *obj, uint32_t address, const uint8_t *data, uint32_t size); 00078 00079 /** Get sector size 00080 * 00081 * @param obj The flash object 00082 * @param address The sector starting address 00083 * @return The size of a sector 00084 */ 00085 uint32_t flash_get_sector_size(const flash_t *obj, uint32_t address); 00086 00087 /** Get page size 00088 * 00089 * @param obj The flash object 00090 * @param address The page starting address 00091 * @return The size of a page 00092 */ 00093 uint32_t flash_get_page_size(const flash_t *obj); 00094 00095 /** Get start address for the flash region 00096 * 00097 * @param obj The flash object 00098 * @return The start address for the flash region 00099 */ 00100 uint32_t flash_get_start_address(const flash_t *obj); 00101 00102 /** Get the flash region size 00103 * 00104 * @param obj The flash object 00105 * @return The flash region size 00106 */ 00107 uint32_t flash_get_size(const flash_t *obj); 00108 00109 /**@}*/ 00110 00111 #ifdef __cplusplus 00112 } 00113 #endif 00114 00115 #endif 00116 00117 #endif 00118 00119 /** @}*/
Generated on Tue Jul 12 2022 16:30:32 by
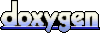