Modification of Mbed-dev library for LQFP48 package microcontrollers: STM32F103C8 (STM32F103C8T6) and STM32F103CB (STM32F103CBT6) (Bluepill boards, Maple mini etc. )
Fork of mbed-STM32F103C8_org by
emac_api.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2016 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_EMAC_API_H 00018 #define MBED_EMAC_API_H 00019 00020 #if DEVICE_EMAC 00021 00022 #include <stdbool.h> 00023 #include "emac_stack_mem.h" 00024 00025 typedef struct emac_interface emac_interface_t; 00026 00027 /** 00028 * EmacInterface 00029 * 00030 * This interface should be used to abstract low level access to networking hardware 00031 */ 00032 00033 /** 00034 * Callback to be register with Emac interface and to be called fore received packets 00035 * 00036 * @param data Arbitrary user data (IP stack) 00037 * @param buf Received data 00038 */ 00039 typedef void (*emac_link_input_fn)(void *data, emac_stack_mem_chain_t *buf); 00040 00041 /** 00042 * Callback to be register with Emac interface and to be called for link status changes 00043 * 00044 * @param data Arbitrary user data (IP stack) 00045 * @param up Link status 00046 */ 00047 typedef void (*emac_link_state_change_fn)(void *data, bool up); 00048 00049 /** 00050 * Return maximum transmission unit 00051 * 00052 * @param emac Emac interface 00053 * @return MTU in bytes 00054 */ 00055 typedef uint32_t (*emac_get_mtu_size_fn)(emac_interface_t *emac); 00056 00057 /** 00058 * Return interface name 00059 * 00060 * @param emac Emac interface 00061 * @param name Pointer to where the name should be written 00062 * @param size Maximum number of character to copy 00063 */ 00064 typedef void (*emac_get_ifname_fn)(emac_interface_t *emac, char *name, uint8_t size); 00065 00066 /** 00067 * Returns size of the underlying interface HW address size 00068 * 00069 * @param emac Emac interface 00070 * @return HW address size in bytes 00071 */ 00072 typedef uint8_t (*emac_get_hwaddr_size_fn)(emac_interface_t *emac); 00073 00074 /** 00075 * Return interface hw address 00076 * 00077 * Copies HW address to provided memory, @param addr has to be of correct size see @a get_hwaddr_size 00078 * 00079 * @param emac Emac interface 00080 * @param addr HW address for underlying interface 00081 */ 00082 typedef void (*emac_get_hwaddr_fn)(emac_interface_t *emac, uint8_t *addr); 00083 00084 /** 00085 * Set HW address for interface 00086 * 00087 * Provided address has to be of correct size, see @a get_hwaddr_size 00088 * 00089 * @param emac Emac interface 00090 * @param addr Address to be set 00091 */ 00092 typedef void (*emac_set_hwaddr_fn)(emac_interface_t *emac, uint8_t *addr); 00093 00094 /** 00095 * Sends the packet over the link 00096 * 00097 * That can not be called from an interrupt context. 00098 * 00099 * @param emac Emac interface 00100 * @param buf Packet to be send 00101 * @return True if the packet was send successfully, False otherwise 00102 */ 00103 typedef bool (*emac_link_out_fn)(emac_interface_t *emac, emac_stack_mem_t *buf); 00104 00105 /** 00106 * Initializes the HW 00107 * 00108 * @return True on success, False in case of an error. 00109 */ 00110 typedef bool (*emac_power_up_fn)(emac_interface_t *emac); 00111 00112 /** 00113 * Deinitializes the HW 00114 * 00115 * @param emac Emac interface 00116 */ 00117 typedef void (*emac_power_down_fn)(emac_interface_t *emac); 00118 00119 /** 00120 * Sets a callback that needs to be called for packets received for that interface 00121 * 00122 * @param emac Emac interface 00123 * @param input_cb Function to be register as a callback 00124 * @param data Arbitrary user data to be passed to the callback 00125 */ 00126 typedef void (*emac_set_link_input_cb_fn)(emac_interface_t *emac, emac_link_input_fn input_cb, void *data); 00127 00128 /** 00129 * Sets a callback that needs to be called on link status changes for given interface 00130 * 00131 * @param emac Emac interface 00132 * @param state_cb Function to be register as a callback 00133 * @param data Arbitrary user data to be passed to the callback 00134 */ 00135 typedef void (*emac_set_link_state_cb_fn)(emac_interface_t *emac, emac_link_state_change_fn state_cb, void *data); 00136 00137 typedef struct emac_interface_ops { 00138 emac_get_mtu_size_fn get_mtu_size; 00139 emac_get_ifname_fn get_ifname; 00140 emac_get_hwaddr_size_fn get_hwaddr_size; 00141 emac_get_hwaddr_fn get_hwaddr; 00142 emac_set_hwaddr_fn set_hwaddr; 00143 emac_link_out_fn link_out; 00144 emac_power_up_fn power_up; 00145 emac_power_down_fn power_down; 00146 emac_set_link_input_cb_fn set_link_input_cb; 00147 emac_set_link_state_cb_fn set_link_state_cb; 00148 } emac_interface_ops_t; 00149 00150 typedef struct emac_interface { 00151 const emac_interface_ops_t ops; 00152 void *hw; 00153 } emac_interface_t; 00154 00155 #else 00156 00157 typedef void *emac_interface_t; 00158 00159 #endif /* DEVICE_EMAC */ 00160 #endif /* MBED_EMAC_API_H */
Generated on Tue Jul 12 2022 16:30:31 by
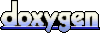