Modification of Mbed-dev library for LQFP48 package microcontrollers: STM32F103C8 (STM32F103C8T6) and STM32F103CB (STM32F103CBT6) (Bluepill boards, Maple mini etc. )
Fork of mbed-STM32F103C8_org by
FlashIAP.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef MBED_FLASHIAP_H 00023 #define MBED_FLASHIAP_H 00024 00025 #ifdef DEVICE_FLASH 00026 00027 #include "flash_api.h" 00028 #include "platform/SingletonPtr.h" 00029 #include "platform/PlatformMutex.h" 00030 00031 namespace mbed { 00032 00033 /** \addtogroup drivers */ 00034 /** @{*/ 00035 00036 /** Flash IAP driver. It invokes flash HAL functions. 00037 * 00038 * Note Synchronization level: Thread safe 00039 */ 00040 class FlashIAP { 00041 public: 00042 FlashIAP(); 00043 ~FlashIAP(); 00044 00045 /** Initialize a flash IAP device 00046 * 00047 * Should be called once per lifetime of the object. 00048 * @return 0 on success or a negative error code on failure 00049 */ 00050 int init(); 00051 00052 /** Deinitialize a flash IAP device 00053 * 00054 * @return 0 on success or a negative error code on failure 00055 */ 00056 int deinit(); 00057 00058 /** Read data from a flash device. 00059 * 00060 * This method invokes memcpy - reads number of bytes from the address 00061 * 00062 * @param buffer Buffer to write to 00063 * @param addr Flash address to begin reading from 00064 * @param size Size to read in bytes 00065 * @return 0 on success, negative error code on failure 00066 */ 00067 int read(void *buffer, uint32_t addr, uint32_t size); 00068 00069 /** Program data to pages 00070 * 00071 * The sectors must have been erased prior to being programmed 00072 * 00073 * @param buffer Buffer of data to be written 00074 * @param addr Address of a page to begin writing to, must be a multiple of program and sector sizes 00075 * @param size Size to write in bytes, must be a multiple of program and sector sizes 00076 * @return 0 on success, negative error code on failure 00077 */ 00078 int program(const void *buffer, uint32_t addr, uint32_t size); 00079 00080 /** Erase sectors 00081 * 00082 * The state of an erased sector is undefined until it has been programmed 00083 * 00084 * @param addr Address of a sector to begin erasing, must be a multiple of the sector size 00085 * @param size Size to erase in bytes, must be a multiple of the sector size 00086 * @return 0 on success, negative error code on failure 00087 */ 00088 int erase(uint32_t addr, uint32_t size); 00089 00090 /** Get the sector size at the defined address 00091 * 00092 * Sector size might differ at address ranges. 00093 * An example <0-0x1000, sector size=1024; 0x10000-0x20000, size=2048> 00094 * 00095 * @param addr Address of or inside the sector to query 00096 * @return Size of a sector in bytes or MBED_FLASH_INVALID_SIZE if not mapped 00097 */ 00098 uint32_t get_sector_size(uint32_t addr) const; 00099 00100 /** Get the flash start address 00101 * 00102 * @return Flash start address 00103 */ 00104 uint32_t get_flash_start() const; 00105 00106 /** Get the flash size 00107 * 00108 * @return Flash size 00109 */ 00110 uint32_t get_flash_size() const; 00111 00112 /** Get the program page size 00113 * 00114 * @return Size of a program page in bytes 00115 */ 00116 uint32_t get_page_size() const; 00117 00118 private: 00119 00120 /** Check if address and size are aligned to a sector 00121 * 00122 * @param addr Address of block to check for alignment 00123 * @param size Size of block to check for alignment 00124 * @return true if the block is sector aligned, false otherwise 00125 */ 00126 bool is_aligned_to_sector(uint32_t addr, uint32_t size); 00127 00128 flash_t _flash; 00129 static SingletonPtr<PlatformMutex> _mutex; 00130 }; 00131 00132 } /* namespace mbed */ 00133 00134 #endif /* DEVICE_FLASH */ 00135 00136 #endif /* MBED_FLASHIAP_H */ 00137 00138 /** @}*/
Generated on Tue Jul 12 2022 16:30:32 by
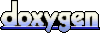