Modification of Mbed-dev library for LQFP48 package microcontrollers: STM32F103C8 (STM32F103C8T6) and STM32F103CB (STM32F103CBT6) (Bluepill boards, Maple mini etc. )
Fork of mbed-STM32F103C8_org by
FileLike.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_FILELIKE_H 00017 #define MBED_FILELIKE_H 00018 00019 #include "platform/mbed_toolchain.h" 00020 #include "drivers/FileBase.h" 00021 00022 namespace mbed { 00023 /** \addtogroup drivers */ 00024 /** @{*/ 00025 00026 00027 /* Class FileLike 00028 * A file-like object is one that can be opened with fopen by 00029 * fopen("/name", mode). 00030 * 00031 * @Note Synchronization level: Set by subclass 00032 */ 00033 class FileLike : public FileBase { 00034 public: 00035 /** Constructor FileLike 00036 * 00037 * @param name The name to use to open the file. 00038 */ 00039 FileLike(const char *name = NULL) : FileBase(name, FilePathType) {} 00040 virtual ~FileLike() {} 00041 00042 /** Read the contents of a file into a buffer 00043 * 00044 * @param buffer The buffer to read in to 00045 * @param size The number of bytes to read 00046 * @return The number of bytes read, 0 at end of file, negative error on failure 00047 */ 00048 virtual ssize_t read(void *buffer, size_t len) = 0; 00049 00050 /** Write the contents of a buffer to a file 00051 * 00052 * @param buffer The buffer to write from 00053 * @param size The number of bytes to write 00054 * @return The number of bytes written, negative error on failure 00055 */ 00056 virtual ssize_t write(const void *buffer, size_t len) = 0; 00057 00058 /** Close a file 00059 * 00060 * @return 0 on success, negative error code on failure 00061 */ 00062 virtual int close() = 0; 00063 00064 /** Flush any buffers associated with the file 00065 * 00066 * @return 0 on success, negative error code on failure 00067 */ 00068 virtual int sync() = 0; 00069 00070 /** Check if the file in an interactive terminal device 00071 * 00072 * @return True if the file is a terminal 00073 */ 00074 virtual int isatty() = 0; 00075 00076 /** Move the file position to a given offset from from a given location 00077 * 00078 * @param offset The offset from whence to move to 00079 * @param whence The start of where to seek 00080 * SEEK_SET to start from beginning of file, 00081 * SEEK_CUR to start from current position in file, 00082 * SEEK_END to start from end of file 00083 * @return The new offset of the file 00084 */ 00085 virtual off_t seek(off_t offset, int whence = SEEK_SET) = 0; 00086 00087 /** Get the file position of the file 00088 * 00089 * @return The current offset in the file 00090 */ 00091 virtual off_t tell() = 0; 00092 00093 /** Rewind the file position to the beginning of the file 00094 * 00095 * @note This is equivalent to file_seek(file, 0, FS_SEEK_SET) 00096 */ 00097 virtual void rewind() = 0; 00098 00099 /** Get the size of the file 00100 * 00101 * @return Size of the file in bytes 00102 */ 00103 virtual size_t size() = 0; 00104 00105 /** Move the file position to a given offset from a given location. 00106 * 00107 * @param offset The offset from whence to move to 00108 * @param whence SEEK_SET for the start of the file, SEEK_CUR for the 00109 * current file position, or SEEK_END for the end of the file. 00110 * 00111 * @returns 00112 * new file position on success, 00113 * -1 on failure or unsupported 00114 */ 00115 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by FileLike::seek") 00116 virtual off_t lseek(off_t offset, int whence) { return seek(offset, whence); } 00117 00118 /** Flush any buffers associated with the FileHandle, ensuring it 00119 * is up to date on disk 00120 * 00121 * @returns 00122 * 0 on success or un-needed, 00123 * -1 on error 00124 */ 00125 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by FileLike::sync") 00126 virtual int fsync() { return sync(); } 00127 00128 /** Find the length of the file 00129 * 00130 * @returns 00131 * Length of the file 00132 */ 00133 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by FileLike::size") 00134 virtual off_t flen() { return size(); } 00135 00136 protected: 00137 /** Acquire exclusive access to this object. 00138 */ 00139 virtual void lock() { 00140 // Stub 00141 } 00142 00143 /** Release exclusive access to this object. 00144 */ 00145 virtual void unlock() { 00146 // Stub 00147 } 00148 }; 00149 00150 00151 /** @}*/ 00152 } // namespace mbed 00153 00154 #endif
Generated on Tue Jul 12 2022 16:30:32 by
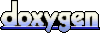