Current transducer. For the electronic measurement of currents: DC, AC, pulsed..., with galvanic separation between the primary circuit and the secondary circuit.
LEM_HAIS.h
00001 /** 00002 * @brief LEM_HAIS.h 00003 * @details Current transducer. For the electronic measurement of currents: 00004 * DC, AC, pulsed..., with galvanic separation between the primary 00005 * circuit and the secondary circuit. 00006 * Header file. 00007 * 00008 * 00009 * @return NA 00010 * 00011 * @author Manuel Caballero 00012 * @date 19/September/2017 00013 * @version 19/September/2017 The ORIGIN 00014 * @pre NaN. 00015 * @warning NaN 00016 * @pre This code belongs to Nimbus Centre ( http://www.nimbus.cit.ie ). 00017 */ 00018 #ifndef LEM_HAIS_H 00019 #define LEM_HAIS_H 00020 00021 #include "mbed.h" 00022 00023 00024 /** 00025 Example: 00026 00027 #include "mbed.h" 00028 #include "LEM_HAIS.h" 00029 00030 00031 LEM_HAIS myCurrentTransducer ( p20 ); 00032 Serial pc ( USBTX, USBRX ); 00033 00034 Ticker newReading; 00035 DigitalOut myled1 ( LED1 ); 00036 DigitalOut myled2 ( LED2 ); 00037 AnalogIn myVref ( p19 ); 00038 AnalogIn myINPUT ( p20 ); 00039 00040 00041 LEM_HAIS::LEM_HAIS_parameters_t myParameters; 00042 LEM_HAIS::LEM_HAIS_voltage_t myVoltages; 00043 00044 00045 void readDATA ( void ) 00046 { 00047 LEM_HAIS::LEM_HAIS_current_t myCurrent; 00048 00049 myled2 = 1; 00050 00051 myVoltages = myCurrentTransducer.LEM_HAIS_GetVoltage (); 00052 myCurrent = myCurrentTransducer.LEM_HAIS_CalculateCurrent ( myVoltages, myParameters, LEM_HAIS::FILTER_DISABLED ); 00053 00054 pc.printf( "IP: %0.5f A\r\n", myCurrent.Current ); 00055 00056 myled2 = 0; 00057 } 00058 00059 00060 int main() 00061 { 00062 uint32_t i = 0; 00063 00064 // CONFIGURATION. The parameters of the system 00065 // myParameters.lem_hais_reference_voltage = 2.5; // ( uncomment ) Mandatory if calibration is NOT used! 00066 myParameters.voltage_divider = 2.0; // Resistor ( both with the same value ) divider at the Sensor ( LEM-HAIS ) Vout 00067 myParameters.adc_reference_voltage = 3.3; // ADC microcontroller ~ 3.3V 00068 myParameters.lem_hais_ipm = 150.0; // HAIS 150-P 00069 00070 pc.baud ( 115200 ); 00071 00072 00073 // [ OPTIONAL ] CALIBRATION. It calculates the offset to calibrate the future measurements. 00074 // It reads the Vref from the device. 00075 myled1 = 1; 00076 for ( i = 0; i < 10; i++ ) { 00077 myParameters.lem_hais_reference_voltage += myVref.read(); 00078 wait ( 0.25 ); 00079 } 00080 00081 myParameters.lem_hais_reference_voltage /= 10.0; 00082 myParameters.lem_hais_reference_voltage *= myParameters.adc_reference_voltage; 00083 00084 // It reads OUPUT from the device. NOTE: This MUST be done at 0A current!!! 00085 myParameters.lem_hais_offset_voltage = myCurrentTransducer.LEM_HAIS_SetAutoOffset ( myParameters ); 00086 myled1 = 0; 00087 00088 pc.printf( "Vref: %0.5f V Voff: %0.5f V\r\n", myParameters.lem_hais_reference_voltage, myParameters.lem_hais_offset_voltage ); 00089 // CALIBRATION ends here 00090 00091 00092 newReading.attach( &readDATA, 1.5 ); // the address of the function to be attached ( readDATA ) and the interval ( 1.5s ) 00093 00094 00095 // Let the callbacks take care of everything 00096 while(1) { 00097 sleep(); 00098 } 00099 } 00100 00101 */ 00102 00103 00104 /*! 00105 Library for the LEM_HAIS Current transducer. 00106 */ 00107 class LEM_HAIS 00108 { 00109 public: 00110 #define CALIBRATION_AVERAGE 10 // Change it if you wish to calculate the offset with less measurements 00111 #define SAMPLE_DATA 1000 // 1 sample every 1ms -> 1000 total samples in total ( = 1s ) 00112 00113 typedef enum { 00114 FILTER_ENABLED = 1, 00115 FILTER_DISABLED = 0 00116 } LEM_HAIS_filter_status_t; 00117 00118 00119 00120 00121 #ifndef LEM_HAIS_DATA_STRUCT_H 00122 #define LEM_HAIS_DATA_STRUCT_H 00123 typedef struct { 00124 float Current; 00125 } LEM_HAIS_current_t; 00126 00127 typedef struct { 00128 float OUTPUT_Voltage[SAMPLE_DATA]; 00129 } LEM_HAIS_voltage_t; 00130 00131 typedef struct { 00132 float lem_hais_offset_voltage; 00133 float lem_hais_reference_voltage; 00134 float voltage_divider; 00135 float adc_reference_voltage; 00136 float lem_hais_ipm; 00137 } LEM_HAIS_parameters_t; 00138 #endif 00139 00140 00141 00142 00143 /** Create an LEM_HAIS object connected to the specified pins. 00144 * 00145 * @param OUTPUT Vout from the device 00146 */ 00147 LEM_HAIS ( PinName OUTPUT ); 00148 00149 /** Delete LEM_HAIS object. 00150 */ 00151 ~LEM_HAIS(); 00152 00153 /** It gets the voltage. 00154 */ 00155 LEM_HAIS_voltage_t LEM_HAIS_GetVoltage ( void ); 00156 00157 /** It calculates the offset automatically ( at 0A current ). 00158 */ 00159 float LEM_HAIS_SetAutoOffset ( LEM_HAIS_parameters_t myParameters ); 00160 00161 /** It calculates the current. 00162 */ 00163 LEM_HAIS_current_t LEM_HAIS_CalculateCurrent ( LEM_HAIS_voltage_t myVoltages, LEM_HAIS_parameters_t myParameters, LEM_HAIS_filter_status_t myFilter = FILTER_DISABLED ); 00164 00165 00166 00167 private: 00168 AnalogIn _OUTPUT; 00169 }; 00170 00171 #endif
Generated on Mon Jul 18 2022 17:34:09 by
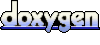