Current transducer. For the electronic measurement of currents: DC, AC, pulsed..., with galvanic separation between the primary circuit and the secondary circuit.
LEM_HAIS.cpp
00001 /** 00002 * @brief LEM_HAIS.h 00003 * @details Current transducer. For the electronic measurement of currents: 00004 * DC, AC, pulsed..., with galvanic separation between the primary 00005 * circuit and the secondary circuit. 00006 * Function file. 00007 * 00008 * 00009 * @return NA 00010 * 00011 * @author Manuel Caballero 00012 * @date 19/September/2017 00013 * @version 19/September/2017 The ORIGIN 00014 * @pre NaN. 00015 * @warning NaN 00016 * @pre This code belongs to Nimbus Centre ( http://www.nimbus.cit.ie ). 00017 */ 00018 00019 #include "LEM_HAIS.h" 00020 00021 LEM_HAIS::LEM_HAIS ( PinName OUTPUT ) 00022 : _OUTPUT ( OUTPUT ) 00023 { 00024 } 00025 00026 LEM_HAIS::~LEM_HAIS() 00027 { 00028 } 00029 00030 00031 00032 /** 00033 * @brief LEM_HAIS_GetVoltage ( void ) 00034 * 00035 * @details It performs a new voltage measurement. 00036 * 00037 * @param[in] NaN. 00038 * 00039 * @param[out] myAuxVoltage: All the samples. 00040 * 00041 * 00042 * @return The actual voltage. 00043 * 00044 * 00045 * @author Manuel Caballero 00046 * @date 19/September/2017 00047 * @version 27/September/2017 RMS calculations included. 00048 * 19/September/2017 The ORIGIN 00049 * @pre If SAMPLE_DATA = 1000, this funcion will last for 1000 * 1ms 00050 * = 1 second. 00051 * @warning NaN. 00052 */ 00053 LEM_HAIS::LEM_HAIS_voltage_t LEM_HAIS::LEM_HAIS_GetVoltage ( void ) 00054 { 00055 uint32_t i = 0; 00056 00057 LEM_HAIS_voltage_t myAuxVoltage; 00058 00059 00060 for ( i = 0; i < SAMPLE_DATA; i++ ) 00061 { 00062 myAuxVoltage.OUTPUT_Voltage[i] = _OUTPUT.read(); 00063 00064 wait_ms ( 1 ); 00065 } 00066 00067 00068 return myAuxVoltage; 00069 } 00070 00071 00072 00073 /** 00074 * @brief LEM_HAIS_CalculateCurrent ( Vector_LEM_HAIS_voltage_t , LEM_HAIS_parameters_t , LEM_HAIS_filter_status_t ) 00075 * 00076 * @details It calculates the actual current. 00077 * 00078 * @param[in] myVoltages: Both voltages, OUTPUT and Vref voltages. 00079 * @param[in] myParameters: Parameters are necessary to calculate the 00080 * result. 00081 * @param[in] myFilter: If a low pass filter is enabled/disabled. 00082 * 00083 * @param[out] NaN. 00084 * 00085 * 00086 * @return The calculated current. 00087 * 00088 * 00089 * @author Manuel Caballero 00090 * @date 19/September/2017 00091 * @version 10/October/2017 New parameters are sent to this function to 00092 * process the data in the right way. 00093 * 19/September/2017 The ORIGIN 00094 * @pre LEM_HAIS_GetVoltage function MUST be called first. 00095 * @warning NaN. 00096 */ 00097 LEM_HAIS::LEM_HAIS_current_t LEM_HAIS::LEM_HAIS_CalculateCurrent ( LEM_HAIS_voltage_t myVoltages, LEM_HAIS_parameters_t myParameters, LEM_HAIS_filter_status_t myFilter ) 00098 { 00099 LEM_HAIS_current_t myAuxCurrent; 00100 00101 float myAuxSQI = 0; 00102 float myAuxVol = 0; 00103 float myI_filtered = 0; 00104 float myI_Previousfiltered = 0; 00105 uint32_t i = 0; 00106 00107 00108 00109 // Check if we want to use a low pass filter 00110 if ( myFilter == FILTER_ENABLED ) 00111 { 00112 myI_Previousfiltered = myParameters.voltage_divider * myParameters.adc_reference_voltage * myVoltages.OUTPUT_Voltage[0]; 00113 i = 1; 00114 } 00115 else 00116 i = 0; 00117 00118 00119 // Calculate the RMS current 00120 for ( ; i < SAMPLE_DATA; i++ ) 00121 { 00122 myAuxVol = myParameters.voltage_divider * myParameters.adc_reference_voltage * myVoltages.OUTPUT_Voltage[i]; 00123 00124 if ( myFilter == FILTER_ENABLED ) 00125 { 00126 myI_filtered = ( ( 1 - 0.5 ) * myAuxVol ) + ( 0.5 * myI_Previousfiltered ); 00127 00128 myI_Previousfiltered = myI_filtered; 00129 00130 myAuxSQI = ( 8.0 / 5.0 ) * ( ( myI_filtered + myParameters.lem_hais_offset_voltage ) - myParameters.lem_hais_reference_voltage ) * myParameters.lem_hais_ipm; 00131 } 00132 else 00133 myAuxSQI = ( 8.0 / 5.0 ) * ( ( myAuxVol + myParameters.lem_hais_offset_voltage ) - myParameters.lem_hais_reference_voltage ) * myParameters.lem_hais_ipm; 00134 00135 00136 myAuxSQI *= myAuxSQI; 00137 00138 00139 myAuxCurrent.Current += myAuxSQI; 00140 } 00141 00142 00143 if ( myFilter == FILTER_ENABLED ) 00144 i = 1; 00145 else 00146 i = 0; 00147 00148 00149 myAuxCurrent.Current /= ( float )( SAMPLE_DATA - i ); 00150 myAuxCurrent.Current = sqrt( myAuxCurrent.Current ); 00151 00152 00153 00154 return myAuxCurrent; 00155 } 00156 00157 00158 00159 /** 00160 * @brief LEM_HAIS_SetAutoOffset ( LEM_HAIS_parameters_t ) 00161 * 00162 * @details It calculates the offset automatically ( at 0A current ). 00163 * 00164 * @param[in] myParameters: voltage_divider, adc_reference_voltage. These 00165 * parameters are necessary to calculate the 00166 * result. 00167 * 00168 * @param[out] NaN. 00169 * 00170 * 00171 * @return The actual offset volatge. 00172 * 00173 * 00174 * @author Manuel Caballero 00175 * @date 19/September/2017 00176 * @version 10/October/2017 New parameters are sent to this function to 00177 * process the data in the right way. 00178 * 19/September/2017 The ORIGIN 00179 * @pre If CALIBRATION_AVERAGE = 10, this function will last for 00180 * 10 * 0.25 = 2.5 seconds. 00181 * @warning This test has to be perfomed at 0A ( no current through 00182 * the sensor at all ). 00183 */ 00184 float LEM_HAIS::LEM_HAIS_SetAutoOffset ( LEM_HAIS_parameters_t myParameters ) 00185 { 00186 uint32_t i = 0; 00187 float myVoltage = 0; 00188 00189 00190 // Calculate the average, get a new measurement every 0.25s 00191 for ( i = 0; i < CALIBRATION_AVERAGE; i++ ){ 00192 myVoltage += _OUTPUT.read(); 00193 wait ( 0.25 ); 00194 } 00195 00196 myVoltage /= ( float )CALIBRATION_AVERAGE; 00197 00198 00199 // Store the offset 00200 myParameters.lem_hais_offset_voltage = myParameters.lem_hais_reference_voltage - ( myParameters.voltage_divider * myVoltage * myParameters.adc_reference_voltage ); 00201 00202 00203 00204 return myParameters.lem_hais_offset_voltage; 00205 }
Generated on Mon Jul 18 2022 17:34:09 by
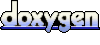