
Fork to see if I can get working
Dependencies: BufferedSerial OneWire WinbondSPIFlash libxDot-dev-mbed5-deprecated
Fork of xDotBridge_update_test20180823 by
testLRRPins.cpp
00001 #include "mbed.h" 00002 #include <string> 00003 #include "../../config.h" 00004 00005 #ifdef __TEST_LRR__ 00006 DigitalOut gpio0(GPIO0); 00007 DigitalOut gpio1(GPIO1); 00008 DigitalOut gpio2(GPIO2); 00009 DigitalOut gpio3(GPIO3); 00010 DigitalOut wake_DOUT(WAKE); 00011 DigitalOut i2cOut1(I2C1_SCL); 00012 DigitalOut i2cOut2(I2C1_SDA); 00013 00014 DigitalOut uartCts(UART1_CTS); 00015 DigitalOut uartRts(UART1_RTS); 00016 DigitalOut uartTx(UART1_TX); 00017 DigitalOut uartRx(UART1_RX); 00018 00019 DigitalOut mosi(SPI_MOSI); 00020 DigitalOut sck(SPI_SCK); 00021 00022 DigitalOut nss(SPI_NSS, 1); // if low then miso on the flash becomes output 00023 DigitalInOut miso(SPI_MISO, PIN_INPUT, PullNone, 0); 00024 00025 Serial pc(USBTX, USBRX); // Externally defined 00026 00027 const static std::string PIN_NAMES [] = { 00028 "Pin 2: UART_TX ", // Idx 0 00029 "Pin 3: UART_RX ", // Idx 1 00030 "Pin 4: MISO ", // Idx 2 00031 "Pin 6: SCL ", // Idx 3 00032 "Pin 7: SDA ", // Idx 4 00033 "Pin 11: MOSI ", // Idx 5 00034 "Pin 12: UART_CTS", // Idx 6 00035 "Pin 13: WAKE ", // Idx 7 00036 "Pin 15: GPIO2 ", // Idx 8 00037 "Pin 16: UART_RTS", // Idx 9 00038 "Pin 17: NSS ", // Idx 10 00039 "Pin 18: SCK ", // Idx 11 00040 "Pin 19: GPIO1 ", // Idx 12 00041 "Pin 20: GPIO3 " // Idx 13 00042 }; 00043 00044 const uint8_t config0PinIdx[] = {0,1, 3,4,5,6,7,8,9,10,11,12,13}; 00045 const uint8_t config1PinIdx[] = {0,1,2,3,4,5,6,7,8,9, 11,12,13}; 00046 00047 class MenuManager 00048 { 00049 private: 00050 uint8_t mCurrSel; // Current selection 00051 bool validInput(uint8_t in) { 00052 return in <= 1; // Either 0, 1 00053 } 00054 public: 00055 MenuManager() { 00056 mCurrSel = 0; 00057 } 00058 uint8_t getCurrentSel() { 00059 return mCurrSel; 00060 } 00061 void applyInput(uint8_t in) { 00062 if (validInput(in)) { 00063 mCurrSel = in; 00064 } 00065 } 00066 void printMenu() { 00067 pc.printf("===============================================\r\n"); 00068 pc.printf("= LRR I/O Tester =\r\n"); 00069 pc.printf("===============================================\r\n"); 00070 pc.printf("= Option 0: MISO Disabled, NSS Enabled =\r\n"); 00071 pc.printf("= Option 1: MISO Enabled, NSS Disabled = \r\n"); 00072 pc.printf("= Current Selection is %d =\r\n", mCurrSel); 00073 pc.printf("===============================================\r\n"); 00074 pc.printf("= Details: =\r\n"); 00075 if (mCurrSel == 0) { 00076 pc.printf("= The following pins are toggling: =\r\n"); 00077 pc.printf("= Pin #: Name =\r\n"); 00078 for (unsigned int i=0; i < sizeof(config0PinIdx); i++) { 00079 pc.printf("= %s =\r\n", PIN_NAMES[i].c_str()); 00080 } 00081 } 00082 else if (mCurrSel == 1) { 00083 pc.printf("= The following pins are toggling: =\r\n"); 00084 pc.printf("= Pin #: Name =\r\n"); 00085 for (unsigned int i=0; i < sizeof(config1PinIdx); i++) { 00086 pc.printf("= %s =\r\n", PIN_NAMES[i].c_str()); 00087 } 00088 } 00089 pc.printf("===============================================\r\n"); 00090 } 00091 }; 00092 00093 /** 00094 * Checks that in idle state all the IOs are pulled up. 00095 */ 00096 int main () 00097 { 00098 pc.baud(115200); 00099 00100 MenuManager menuMgr; 00101 menuMgr.printMenu(); 00102 00103 while (true) { 00104 gpio0 = !gpio0; 00105 gpio1 = !gpio1; 00106 gpio2 = !gpio2; 00107 gpio3 = !gpio3; 00108 wake_DOUT = !wake_DOUT; 00109 i2cOut1 = !i2cOut1; 00110 i2cOut2 = !i2cOut2; 00111 00112 uartCts = !uartCts; 00113 uartRts = !uartRts; 00114 uartTx = !uartTx; 00115 uartRx = !uartRx; 00116 00117 mosi = !mosi; 00118 sck = !sck; 00119 00120 if (menuMgr.getCurrentSel() == 0) { 00121 miso.input(); 00122 nss = !nss; 00123 } 00124 else if (menuMgr.getCurrentSel() == 1) { 00125 nss = 1; // Disable flash 00126 miso.output(); 00127 miso = !miso; 00128 } 00129 00130 if (pc.readable()) { 00131 char menuInput = pc.getc(); 00132 menuInput -= '0'; // Convert to raw interger value 00133 menuMgr.applyInput(menuInput); 00134 menuMgr.printMenu(); 00135 } 00136 else { 00137 pc.printf("*"); 00138 } 00139 wait(1.0); 00140 } 00141 return 0; 00142 } 00143 #endif
Generated on Fri Jul 15 2022 14:36:45 by
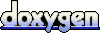