
Fork to see if I can get working
Dependencies: BufferedSerial OneWire WinbondSPIFlash libxDot-dev-mbed5-deprecated
Fork of xDotBridge_update_test20180823 by
UserInterface.cpp
00001 /* 00002 * UserInterface.cpp 00003 * 00004 */ 00005 00006 #include "UserInterface.h" 00007 00008 uint32_t HoldTimeSetting::rotVal2Msec(uint8_t in) { 00009 switch(in) { 00010 case 1: 00011 return 10e3; // 10 sec 00012 break; 00013 case 2: 00014 return 20e3; // 20 sec 00015 break; 00016 case 3: 00017 return 30e3; // 30 sec 00018 break; 00019 case 4: 00020 return 40e3; // 40 sec 00021 break; 00022 case 5: 00023 return 50e3; // 50 sec 00024 break; 00025 case 6: 00026 return 60e3; // 60 sec 00027 break; 00028 case 7: 00029 return 2*60e3; // 2 mins 00030 break; 00031 case 8: 00032 return 5*60e3; // 5 mins 00033 break; 00034 case 9: 00035 return 10*60e3; // 10 mins 00036 break; 00037 case 0: 00038 return 500; // 0.5 sec 00039 break; 00040 default: // Match case 0 00041 return 500; // 0.5 sec 00042 } 00043 } 00044 00045 PairBtnState PairBtnInterp::read(BaseboardIO *bbio) 00046 { 00047 const uint8_t holdCnt2ms = 100; // 100 ms per 0.1 sec count 00048 uint8_t holdCnt = 0; 00049 for (holdCnt=0; holdCnt < 90; holdCnt++) { 00050 if (bbio->isPairBtn() == false){ // Button released 00051 break; 00052 } 00053 // TODO Sleep here rather than just wait 00054 wait(0.1); 00055 } 00056 if (ShortPressStartTime <= holdCnt*holdCnt2ms 00057 && holdCnt*holdCnt2ms < ShortPressStopTime) { 00058 return pairBtnShortPress; 00059 } 00060 else if (MediumPressStartTime <= holdCnt*holdCnt2ms 00061 && holdCnt*holdCnt2ms < MediumPressStopTime) { 00062 return pairBtnMediumPress; 00063 } 00064 else { 00065 return pairBtnLongPress; 00066 } 00067 } 00068 00069 LedPatterns::LedPatterns(BaseboardIO *bbio) 00070 { 00071 mBbio = bbio; 00072 } 00073 00074 void LedPatterns::turnOn() 00075 { 00076 mBbio->ledOn(); 00077 } 00078 00079 void LedPatterns::turnOff() 00080 { 00081 mBbio->ledOff(); 00082 } 00083 00084 void LedPatterns::singleBlink() 00085 { 00086 mBbio->ledOn(); 00087 wait(0.5); 00088 mBbio->ledOff(); 00089 } 00090 00091 void LedPatterns::tripleBlink() 00092 { 00093 singleBlink(); 00094 wait(0.5); 00095 singleBlink(); 00096 wait(0.5); 00097 singleBlink(); 00098 } 00099 00100 void LedPatterns::tenBlinks() 00101 { 00102 for (uint8_t i=0; i<10; i++) { 00103 singleBlink(); 00104 wait(0.5); 00105 } 00106 }
Generated on Fri Jul 15 2022 14:36:45 by
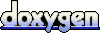