
Fork to see if I can get working
Dependencies: BufferedSerial OneWire WinbondSPIFlash libxDot-dev-mbed5-deprecated
Fork of xDotBridge_update_test20180823 by
RadioEvent.h
00001 #ifndef __RADIO_EVENT_H__ 00002 #define __RADIO_EVENT_H__ 00003 00004 #include "dot_util.h" 00005 #include "mDotEvent.h" 00006 00007 class RadioEvent : public mDotEvent 00008 { 00009 00010 public: 00011 RadioEvent() {} 00012 00013 virtual ~RadioEvent() {} 00014 00015 /*! 00016 * MAC layer event callback prototype. 00017 * 00018 * \param [IN] flags Bit field indicating the MAC events occurred 00019 * \param [IN] info Details about MAC events occurred 00020 */ 00021 virtual void MacEvent(LoRaMacEventFlags* flags, LoRaMacEventInfo* info) { 00022 00023 if (mts::MTSLog::getLogLevel() == mts::MTSLog::TRACE_LEVEL) { 00024 std::string msg = "OK"; 00025 switch (info->Status) { 00026 case LORAMAC_EVENT_INFO_STATUS_ERROR: 00027 msg = "ERROR"; 00028 break; 00029 case LORAMAC_EVENT_INFO_STATUS_TX_TIMEOUT: 00030 msg = "TX_TIMEOUT"; 00031 break; 00032 case LORAMAC_EVENT_INFO_STATUS_RX_TIMEOUT: 00033 msg = "RX_TIMEOUT"; 00034 break; 00035 case LORAMAC_EVENT_INFO_STATUS_RX_ERROR: 00036 msg = "RX_ERROR"; 00037 break; 00038 case LORAMAC_EVENT_INFO_STATUS_JOIN_FAIL: 00039 msg = "JOIN_FAIL"; 00040 break; 00041 case LORAMAC_EVENT_INFO_STATUS_DOWNLINK_FAIL: 00042 msg = "DOWNLINK_FAIL"; 00043 break; 00044 case LORAMAC_EVENT_INFO_STATUS_ADDRESS_FAIL: 00045 msg = "ADDRESS_FAIL"; 00046 break; 00047 case LORAMAC_EVENT_INFO_STATUS_MIC_FAIL: 00048 msg = "MIC_FAIL"; 00049 break; 00050 default: 00051 break; 00052 } 00053 logTrace("Event: %s", msg.c_str()); 00054 00055 logTrace("Flags Tx: %d Rx: %d RxData: %d RxSlot: %d LinkCheck: %d JoinAccept: %d", 00056 flags->Bits.Tx, flags->Bits.Rx, flags->Bits.RxData, flags->Bits.RxSlot, flags->Bits.LinkCheck, flags->Bits.JoinAccept); 00057 logTrace("Info: Status: %d ACK: %d Retries: %d TxDR: %d RxPort: %d RxSize: %d RSSI: %d SNR: %d Energy: %d Margin: %d Gateways: %d", 00058 info->Status, info->TxAckReceived, info->TxNbRetries, info->TxDatarate, info->RxPort, info->RxBufferSize, 00059 info->RxRssi, info->RxSnr, info->Energy, info->DemodMargin, info->NbGateways); 00060 } 00061 00062 if (flags->Bits.Rx) { 00063 00064 logDebug("Rx %d bytes", info->RxBufferSize); 00065 if (info->RxBufferSize > 0) { 00066 // print RX data as hexadecimal 00067 //printf("Rx data: %s\r\n", mts::Text::bin2hexString(info->RxBuffer, info->RxBufferSize).c_str()); 00068 00069 // print RX data as string 00070 std::string rx((const char*)info->RxBuffer, info->RxBufferSize); 00071 mtsLogInfo("Rx data: %s\r\n", rx.c_str()); 00072 } 00073 } 00074 } 00075 }; 00076 00077 #endif 00078
Generated on Fri Jul 15 2022 14:36:45 by
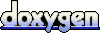