
Fork to see if I can get working
Dependencies: BufferedSerial OneWire WinbondSPIFlash libxDot-dev-mbed5-deprecated
Fork of xDotBridge_update_test20180823 by
MyLog.h
00001 #ifndef MYLOG_H 00002 #define MYLOG_H 00003 00004 #include "MTSLog.h" 00005 00006 //inline const char* className(const std::string& prettyFunction) 00007 //{ 00008 // size_t colons = prettyFunction.find_last_of("::"); 00009 // if (colons == std::string::npos) 00010 // return ""; 00011 // size_t begin = prettyFunction.substr(0,colons).rfind(" ") + 1; 00012 // size_t end = colons - begin; 00013 // 00014 // return prettyFunction.substr(begin,end).c_str(); 00015 //} 00016 // 00017 //#define __CLASSNAME__ className(__PRETTY_FUNCTION__) 00018 00019 00020 #ifdef MTS_DEBUG 00021 #define logFatal(format, ...) \ 00022 mts::MTSLog::printMessage(mts::MTSLog::FATAL_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::FATAL_LABEL, ##__VA_ARGS__) 00023 #define logError(format, ...) \ 00024 mts::MTSLog::printMessage(mts::MTSLog::ERROR_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::ERROR_LABEL, ##__VA_ARGS__) 00025 #define logWarning(format, ...) \ 00026 mts::MTSLog::printMessage(mts::MTSLog::WARNING_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::WARNING_LABEL, ##__VA_ARGS__) 00027 #define logInfo(format, ...) \ 00028 mts::MTSLog::printMessage(mts::MTSLog::INFO_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::INFO_LABEL, ##__VA_ARGS__) 00029 #define logDebug(format, ...) \ 00030 mts::MTSLog::printMessage(mts::MTSLog::DEBUG_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::DEBUG_LABEL, ##__VA_ARGS__) 00031 #define logTrace(format, ...) \ 00032 mts::MTSLog::printMessage(mts::MTSLog::TRACE_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::TRACE_LABEL, ##__VA_ARGS__) 00033 #else 00034 #define myLogFatal(format, ...) \ 00035 MyLog::printMessage(MyLog::FATAL_LEVEL, "[%s] " format "\r\n", MyLog::FATAL_LABEL, ##__VA_ARGS__) 00036 #define myLogError(format, ...) \ 00037 MyLog::printMessage(MyLog::ERROR_LEVEL, "[%s] " format "\r\n", MyLog::ERROR_LABEL, ##__VA_ARGS__) 00038 #define myLogWarning(format, ...) \ 00039 MyLog::printMessage(MyLog::WARNING_LEVEL, "[%s] " format "\r\n", MyLog::WARNING_LABEL, ##__VA_ARGS__) 00040 #define myLogInfo(format, ...) \ 00041 MyLog::printMessage(MyLog::INFO_LEVEL, "[%s] " format "\r\n", MyLog::INFO_LABEL, ##__VA_ARGS__) 00042 #define myLogDebug(format, ...) \ 00043 MyLog::printMessage(MyLog::DEBUG_LEVEL, "[%s] " format "\r\n", MyLog::DEBUG_LABEL, ##__VA_ARGS__) 00044 #define myLogTrace(format, ...) \ 00045 MyLog::printMessage(MyLog::TRACE_LEVEL, "[%s] " format "\r\n", MyLog::TRACE_LABEL, ##__VA_ARGS__) 00046 #endif 00047 00048 class MyLog 00049 { 00050 public: 00051 00052 /** Enum of log levels. 00053 */ 00054 enum logLevel { 00055 NONE_LEVEL = 0, 00056 FATAL_LEVEL = 1, 00057 ERROR_LEVEL = 2, 00058 WARNING_LEVEL = 3, 00059 INFO_LEVEL = 4, 00060 DEBUG_LEVEL = 5, 00061 TRACE_LEVEL = 6 00062 }; 00063 00064 /** Print log message. 00065 */ 00066 static void printMessage(int level, const char* format, ...); 00067 00068 /** Determine if the given level is currently printable. 00069 */ 00070 static bool printable(int level); 00071 00072 /** Set log level 00073 * Messages with lower priority than the current level will not be printed. 00074 * If the level is set to NONE, no messages will print. 00075 */ 00076 static void setLogLevel(int level); 00077 00078 /** Get the current log level. 00079 */ 00080 static int getLogLevel(); 00081 00082 /** Get string representation of the current log level. 00083 */ 00084 static const char* getLogLevelString(); 00085 00086 static const char* NONE_LABEL; 00087 static const char* FATAL_LABEL; 00088 static const char* ERROR_LABEL; 00089 static const char* WARNING_LABEL; 00090 static const char* INFO_LABEL; 00091 static const char* DEBUG_LABEL; 00092 static const char* TRACE_LABEL; 00093 00094 private: 00095 00096 /** Constructor 00097 */ 00098 MyLog(); 00099 00100 static int currentLevel; 00101 00102 }; 00103 00104 #endif
Generated on Fri Jul 15 2022 14:36:45 by
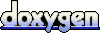