
Fork to see if I can get working
Dependencies: BufferedSerial OneWire WinbondSPIFlash libxDot-dev-mbed5-deprecated
Fork of xDotBridge_update_test20180823 by
DS2408.h
00001 /** 00002 * Library for DS2408 on top of OneWire library 00003 */ 00004 00005 #ifndef XDOT_DS2408_H 00006 #define XDOT_DS2408_H 00007 00008 #include "../config.h" 00009 #include "OneWire.h" 00010 00011 const uint8_t DS2408_NRETRIES = 5; 00012 00013 /** 00014 * @class DS2408 00015 * @brief This class abstracts communicating with the DS2408 port expander. 00016 * The OneWire library is used for physical layer communication and this class 00017 * simply makes it easy to communicate with this one chip. 00018 */ 00019 class DS2408 00020 { 00021 public: 00022 enum DS2408RegAddr { 00023 pioLogicStateReg = 0x88, 00024 pioOutputLatchStateReg = 0x89, 00025 pioActivityStateReg = 0x8A, 00026 condSearchChSelMaskReg = 0x8B, 00027 condSearchChPolMaskReg = 0x8C, 00028 ctrlStatusReg = 0x8D 00029 }; 00030 00031 /** 00032 * @brief DS2408 constructor 00033 * 00034 * @details Just initialized internal variables and disables test mode 00035 * 00036 * On Entry: 00037 * @param[in] owMaster - reference to 1-wire master 00038 * @param[in] romAddr - 64-bit address of ROM 00039 * 00040 * On Exit: 00041 * 00042 * @return 00043 */ 00044 DS2408(OneWire *owMaster, uint8_t romAddr[8]); 00045 00046 /** 00047 * @brief registerRead() 00048 * 00049 * @details reads state of pio 00050 * 00051 * 00052 * On Exit: 00053 * @param[in] addr - per datasheet valid registers are from 0x88 to 0x8D. 00054 * Note value will be automatically promoted to 16-bit value. 00055 * @param[out] val - register value 00056 * 00057 * @return Result of operation zero for success 00058 */ 00059 CmdResult registerRead(uint8_t addr, uint8_t &val); 00060 00061 CmdResult registerReadReliable(uint8_t addr, uint8_t &val); 00062 00063 /** 00064 * @brief pioLogicRead() 00065 * 00066 * @details Uses regisrterRead to get logic values 00067 * 00068 * 00069 * On Exit: 00070 * @param[out] val - lsb represents the state of the pio 00071 * 00072 * @return Result of operation zero for success 00073 */ 00074 CmdResult pioLogicRead(uint8_t &val); 00075 00076 /** 00077 * @brief pioLogicReliableRead() 00078 * 00079 * @details Uses regisrterRead to get logic values. Then reads again to check for bit errors. 00080 * 00081 * 00082 * On Exit: 00083 * @param[out] val - lsb represents the state of the pio 00084 * 00085 * @return Result of operation zero for success 00086 */ 00087 CmdResult pioLogicReliableRead(uint8_t &val); 00088 // TODO implement other register based functions 00089 00090 /** 00091 * @brief pioLogicReliableWrite() 00092 * 00093 * @details writes to pio. Note 0 means active pull down and 1 high-Z to 00094 * allow PIO to float high. This will automatically retry for DS2408_NRETRIES 00095 * times. 00096 * 00097 * On Entry: 00098 * @param[in] val - Value for IO. 00099 * 00100 * @return CmdResult - result of operation 00101 */ 00102 CmdResult pioLogicReliableWrite(uint8_t val); 00103 00104 /** 00105 * @brief pioLogicWrite() 00106 * 00107 * @details writes to pio. Note 0 means active pull down and 1 high-Z to 00108 * allow PIO to float high. 00109 * 00110 * On Entry: 00111 * @param[in] val - Value for IO. 00112 * 00113 * @return CmdResult - result of operation 00114 */ 00115 CmdResult pioLogicWrite(uint8_t val); 00116 00117 private: 00118 OneWire *mMaster; 00119 uint8_t mRomAddr[8]; 00120 00121 /** 00122 * @brief disableTestMode() 00123 * 00124 * @details Per datasheet if slew power on has a specific slew rate the 00125 * chip may enter a test mode. See page 38 of application information. 00126 * 00127 * @return CmdResult - result of operation 00128 */ 00129 CmdResult disableTestMode(); 00130 00131 }; 00132 00133 #endif
Generated on Fri Jul 15 2022 14:36:45 by
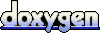