
websocket client for gyro and 3-Axio Accelerometer
Dependencies: ITG3200 MMA7660FC WebSocketClient WiflyInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "Websocket.h" 00004 #include "ITG3200.h" 00005 #include "MMA7660FC.h" 00006 00007 /* wifly interface: 00008 * - p9 and p10 are for the serial communication 00009 * - p19 is for the reset pin 00010 * - p26 is for the connection status 00011 * - "mbed" is the ssid of the network 00012 * - "password" is the password 00013 * - WPA is the security 00014 */ 00015 WiflyInterface wifly(p13, p14, p19, p26, "WWNet", "mmmmmmmm", WPA); 00016 DigitalOut led1(LED1); 00017 DigitalOut led2(LED1); 00018 //gyrc 00019 ITG3200 gyro(p9, p10, 0x68); 00020 //3Axis 00021 #define ADDR_MMA7660 0x98 // I2C SLAVE ADDR MMA7660FC 00022 MMA7660FC Acc(p28, p27, ADDR_MMA7660); //sda, scl, Addr 00023 float G_VALUE[64] = {0, 0.047, 0.094, 0.141, 0.188, 0.234, 0.281, 0.328, 0.375, 0.422, 0.469, 0.516, 0.563, 0.609, 0.656, 0.703, 0.750, 0.797, 0.844, 0.891, 0.938, 0.984, 1.031, 1.078, 1.125, 1.172, 1.219, 1.266, 1.313, 1.359, 1.406, 1.453, -1.500, -1.453, -1.406, -1.359, -1.313, -1.266, -1.219, -1.172, -1.125, -1.078, -1.031, -0.984, -0.938, -0.891, -0.844, -0.797, -0.750, -0.703, -0.656, -0.609, -0.563, -0.516, -0.469, -0.422, -0.375, -0.328, -0.281, -0.234, -0.188, -0.141, -0.094, -0.047}; 00024 00025 00026 int main() { 00027 wifly.init(); //Use DHCP 00028 while (!wifly.connect()); 00029 led1=1; 00030 Websocket ws("ws://192.168.21.104:8080"); 00031 while (!ws.connect()); 00032 led1=2; 00033 00034 00035 //gyro 00036 int gyro_x = 0, gyro_y = 0, gyro_z = 0, temp = 0; 00037 //Set highest bandwidth. 00038 gyro.setLpBandwidth(LPFBW_42HZ); 00039 //3Axio 00040 Acc.init(); // Initialization 00041 00042 while (1) { 00043 char data[256]; 00044 wait(0.1f); 00045 00046 //gyro 00047 gyro_x = gyro.getGyroX(); 00048 gyro_y = gyro.getGyroY(); 00049 gyro_z = gyro.getGyroZ(); 00050 temp = gyro.getTemperature(); 00051 00052 //3-Axio 00053 float x=0, y=0, z=0; 00054 float ax=0, ay=0, az=0; 00055 00056 Acc.read_Tilt(&x, &y, &z); // Read the acceleration 00057 00058 ax = G_VALUE[Acc.read_x()]; 00059 ay = G_VALUE[Acc.read_y()]; 00060 az = G_VALUE[Acc.read_z()]; 00061 00062 00063 00064 //websocket send data 00065 sprintf( data , "{ \"x\": %d, \"y\": %d, \"z\": %d , \"ax\": %f, \"ay\": %f, \"az\": %f\n}", gyro_x, gyro_y, gyro_z ,ax,ay,az); 00066 ws.send(data); 00067 00068 } 00069 } 00070
Generated on Wed Jul 27 2022 12:15:52 by
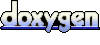