
ch5_mbed_dust_sensor
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Serial pc(USBTX, USBRX); 00004 InterruptIn probe(p19); 00005 DigitalOut led(LED1); 00006 00007 Timer timer; 00008 Ticker ticker; 00009 00010 uint32_t low_time = 0; 00011 00012 void down(); 00013 void up(); 00014 00015 void tick() 00016 { 00017 pc.printf("%d us, %f %%\r\n", low_time, low_time / (30.0 * 1000000)); 00018 low_time = 0; 00019 } 00020 00021 void down() 00022 { 00023 probe.rise(&up); 00024 led = 0; 00025 timer.start(); 00026 } 00027 00028 void up() 00029 { 00030 led = 1; 00031 timer.stop(); 00032 probe.fall(&down); 00033 00034 low_time += timer.read_us(); 00035 timer.reset(); 00036 } 00037 00038 int main() 00039 { 00040 pc.baud(115200); 00041 pc.printf("Dust Sensor test\r\n"); 00042 probe.fall(&down); 00043 ticker.attach(tick, 30); 00044 }
Generated on Mon Jul 18 2022 17:09:45 by
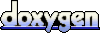