A version of LWIP, provided for backwards compatibility.
Dependents: AA_DemoBoard DemoBoard HelloServerDemo DemoBoard_RangeIndicator ... more
tcpconnection.h
00001 #ifndef TCPCONNECTION_H 00002 #define TCPCONNECTION_H 00003 00004 #include "tcpitem.h" 00005 00006 #include "arch/cc.h" 00007 #include "lwip/err.h" 00008 //#include "lwip/tcp.h" 00009 00010 namespace mbed { 00011 00012 class NetServer; 00013 class TCPListener; 00014 00015 class TCPConnection : public TCPItem { 00016 public: 00017 TCPConnection(struct ip_addr, u16_t); 00018 TCPConnection(TCPListener *, struct tcp_pcb *); 00019 00020 virtual ~TCPConnection(); 00021 00022 void connect(); 00023 00024 err_t write(void *, u32_t len); 00025 void recved(u32_t len); 00026 u16_t sndbuf() { return tcp_sndbuf(_pcb); } 00027 00028 void set_poll_timer(const u32_t &time) { 00029 if(_pcb) { 00030 _pcb->polltmr = time; 00031 } 00032 } 00033 00034 u32_t get_poll_interval() const { 00035 return (_pcb)? _pcb->pollinterval: 0; 00036 } 00037 00038 void set_poll_interval(const u32_t &value) { 00039 if(_pcb) { 00040 _pcb->pollinterval = value; 00041 } 00042 } 00043 00044 u32_t get_poll_timer() const { 00045 return (_pcb)? _pcb->polltmr: 0; 00046 } 00047 00048 00049 /** 00050 * Function to be called when more send buffer space is available. 00051 * @param space the amount of bytes available 00052 * @return ERR_OK: try to send some data by calling tcp_output 00053 */ 00054 virtual err_t sent(u16_t space) = 0; 00055 00056 /** 00057 * Function to be called when (in-sequence) data has arrived. 00058 * @param p the packet buffer which arrived 00059 * @param err an error argument (TODO: that is current always ERR_OK?) 00060 * @return ERR_OK: try to send some data by calling tcp_output 00061 */ 00062 virtual err_t recv(struct pbuf *p, err_t err) = 0; 00063 00064 /** 00065 * Function to be called when a connection has been set up. 00066 * @param pcb the tcp_pcb that now is connected 00067 * @param err an error argument (TODO: that is current always ERR_OK?) 00068 * @return value is currently ignored 00069 */ 00070 virtual err_t connected(err_t err); 00071 00072 /** 00073 * Function which is called periodically. 00074 * The period can be adjusted in multiples of the TCP slow timer interval 00075 * by changing tcp_pcb.polltmr. 00076 * @return ERR_OK: try to send some data by calling tcp_output 00077 */ 00078 virtual err_t poll() = 0; 00079 00080 /** 00081 * Function to be called whenever a fatal error occurs. 00082 * There is no pcb parameter since most of the times, the pcb is 00083 * already deallocated (or there is no pcb) when this function is called. 00084 * @param err an indication why the error callback is called: 00085 * ERR_ABRT: aborted through tcp_abort or by a TCP timer 00086 * ERR_RST: the connection was reset by the remote host 00087 */ 00088 virtual void err(err_t) = 0; 00089 00090 protected: 00091 TCPListener *_parent; 00092 struct ip_addr _ipaddr; 00093 u16_t _port; 00094 friend class NetServer; 00095 }; 00096 00097 }; 00098 00099 #endif /* TCPCONNECTION_H */
Generated on Tue Jul 12 2022 16:06:26 by
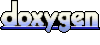