A version of LWIP, provided for backwards compatibility.
Dependents: AA_DemoBoard DemoBoard HelloServerDemo DemoBoard_RangeIndicator ... more
dhcp.c
00001 /** 00002 * @file 00003 * Dynamic Host Configuration Protocol client 00004 * 00005 */ 00006 00007 /* 00008 * 00009 * Copyright (c) 2001-2004 Leon Woestenberg <leon.woestenberg@gmx.net> 00010 * Copyright (c) 2001-2004 Axon Digital Design B.V., The Netherlands. 00011 * All rights reserved. 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. The name of the author may not be used to endorse or promote products 00022 * derived from this software without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00025 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00026 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00027 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00028 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00029 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00030 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00031 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00032 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00033 * OF SUCH DAMAGE. 00034 * 00035 * This file is a contribution to the lwIP TCP/IP stack. 00036 * The Swedish Institute of Computer Science and Adam Dunkels 00037 * are specifically granted permission to redistribute this 00038 * source code. 00039 * 00040 * Author: Leon Woestenberg <leon.woestenberg@gmx.net> 00041 * 00042 * This is a DHCP client for the lwIP TCP/IP stack. It aims to conform 00043 * with RFC 2131 and RFC 2132. 00044 * 00045 * TODO: 00046 * - Proper parsing of DHCP messages exploiting file/sname field overloading. 00047 * - Add JavaDoc style documentation (API, internals). 00048 * - Support for interfaces other than Ethernet (SLIP, PPP, ...) 00049 * 00050 * Please coordinate changes and requests with Leon Woestenberg 00051 * <leon.woestenberg@gmx.net> 00052 * 00053 * Integration with your code: 00054 * 00055 * In lwip/dhcp.h 00056 * #define DHCP_COARSE_TIMER_SECS (recommended 60 which is a minute) 00057 * #define DHCP_FINE_TIMER_MSECS (recommended 500 which equals TCP coarse timer) 00058 * 00059 * Then have your application call dhcp_coarse_tmr() and 00060 * dhcp_fine_tmr() on the defined intervals. 00061 * 00062 * dhcp_start(struct netif *netif); 00063 * starts a DHCP client instance which configures the interface by 00064 * obtaining an IP address lease and maintaining it. 00065 * 00066 * Use dhcp_release(netif) to end the lease and use dhcp_stop(netif) 00067 * to remove the DHCP client. 00068 * 00069 */ 00070 00071 #include "lwip/opt.h" 00072 00073 #if LWIP_DHCP /* don't build if not configured for use in lwipopts.h */ 00074 00075 #include "lwip/stats.h" 00076 #include "lwip/mem.h" 00077 #include "lwip/udp.h" 00078 #include "lwip/ip_addr.h" 00079 #include "lwip/netif.h" 00080 #include "lwip/inet.h" 00081 #include "lwip/sys.h" 00082 #include "lwip/dhcp.h" 00083 #include "lwip/autoip.h" 00084 #include "lwip/dns.h" 00085 #include "netif/etharp.h" 00086 00087 #include <string.h> 00088 00089 /** global transaction identifier, must be 00090 * unique for each DHCP request. We simply increment, starting 00091 * with this value (easy to match with a packet analyzer) */ 00092 static u32_t xid = 0xABCD0000; 00093 00094 /* DHCP client state machine functions */ 00095 static void dhcp_handle_ack(struct netif *netif); 00096 static void dhcp_handle_nak(struct netif *netif); 00097 static void dhcp_handle_offer(struct netif *netif); 00098 00099 static err_t dhcp_discover(struct netif *netif); 00100 static err_t dhcp_select(struct netif *netif); 00101 static void dhcp_check(struct netif *netif); 00102 static void dhcp_bind(struct netif *netif); 00103 #if DHCP_DOES_ARP_CHECK 00104 static err_t dhcp_decline(struct netif *netif); 00105 #endif /* DHCP_DOES_ARP_CHECK */ 00106 static err_t dhcp_rebind(struct netif *netif); 00107 static void dhcp_set_state(struct dhcp *dhcp, u8_t new_state); 00108 00109 /* receive, unfold, parse and free incoming messages */ 00110 static void dhcp_recv(void *arg, struct udp_pcb *pcb, struct pbuf *p, struct ip_addr *addr, u16_t port); 00111 static err_t dhcp_unfold_reply(struct dhcp *dhcp); 00112 static u8_t *dhcp_get_option_ptr(struct dhcp *dhcp, u8_t option_type); 00113 static u8_t dhcp_get_option_byte(u8_t *ptr); 00114 #if 0 00115 static u16_t dhcp_get_option_short(u8_t *ptr); 00116 #endif 00117 static u32_t dhcp_get_option_long(u8_t *ptr); 00118 static void dhcp_free_reply(struct dhcp *dhcp); 00119 00120 /* set the DHCP timers */ 00121 static void dhcp_timeout(struct netif *netif); 00122 static void dhcp_t1_timeout(struct netif *netif); 00123 static void dhcp_t2_timeout(struct netif *netif); 00124 00125 /* build outgoing messages */ 00126 /* create a DHCP request, fill in common headers */ 00127 static err_t dhcp_create_request(struct netif *netif); 00128 /* free a DHCP request */ 00129 static void dhcp_delete_request(struct netif *netif); 00130 /* add a DHCP option (type, then length in bytes) */ 00131 static void dhcp_option(struct dhcp *dhcp, u8_t option_type, u8_t option_len); 00132 /* add option values */ 00133 static void dhcp_option_byte(struct dhcp *dhcp, u8_t value); 00134 static void dhcp_option_short(struct dhcp *dhcp, u16_t value); 00135 static void dhcp_option_long(struct dhcp *dhcp, u32_t value); 00136 /* always add the DHCP options trailer to end and pad */ 00137 static void dhcp_option_trailer(struct dhcp *dhcp); 00138 00139 /** 00140 * Back-off the DHCP client (because of a received NAK response). 00141 * 00142 * Back-off the DHCP client because of a received NAK. Receiving a 00143 * NAK means the client asked for something non-sensible, for 00144 * example when it tries to renew a lease obtained on another network. 00145 * 00146 * We clear any existing set IP address and restart DHCP negotiation 00147 * afresh (as per RFC2131 3.2.3). 00148 * 00149 * @param netif the netif under DHCP control 00150 */ 00151 static void 00152 dhcp_handle_nak(struct netif *netif) 00153 { 00154 struct dhcp *dhcp = netif->dhcp; 00155 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_handle_nak(netif=%p) %c%c%"U16_F"\n", 00156 (void*)netif, netif->name[0], netif->name[1], (u16_t)netif->num)); 00157 /* Set the interface down since the address must no longer be used, as per RFC2131 */ 00158 netif_set_down(netif); 00159 /* remove IP address from interface */ 00160 netif_set_ipaddr(netif, IP_ADDR_ANY); 00161 netif_set_gw(netif, IP_ADDR_ANY); 00162 netif_set_netmask(netif, IP_ADDR_ANY); 00163 /* Change to a defined state */ 00164 dhcp_set_state(dhcp, DHCP_BACKING_OFF); 00165 /* We can immediately restart discovery */ 00166 dhcp_discover(netif); 00167 } 00168 00169 /** 00170 * Checks if the offered IP address is already in use. 00171 * 00172 * It does so by sending an ARP request for the offered address and 00173 * entering CHECKING state. If no ARP reply is received within a small 00174 * interval, the address is assumed to be free for use by us. 00175 * 00176 * @param netif the netif under DHCP control 00177 */ 00178 static void 00179 dhcp_check(struct netif *netif) 00180 { 00181 struct dhcp *dhcp = netif->dhcp; 00182 err_t result; 00183 u16_t msecs; 00184 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_check(netif=%p) %c%c\n", (void *)netif, (s16_t)netif->name[0], 00185 (s16_t)netif->name[1])); 00186 /* create an ARP query for the offered IP address, expecting that no host 00187 responds, as the IP address should not be in use. */ 00188 result = etharp_query(netif, &dhcp->offered_ip_addr, NULL); 00189 if (result != ERR_OK) { 00190 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_check: could not perform ARP query\n")); 00191 } 00192 dhcp->tries++; 00193 msecs = 500; 00194 dhcp->request_timeout = (msecs + DHCP_FINE_TIMER_MSECS - 1) / DHCP_FINE_TIMER_MSECS; 00195 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_check(): set request timeout %"U16_F" msecs\n", msecs)); 00196 dhcp_set_state(dhcp, DHCP_CHECKING); 00197 } 00198 00199 /** 00200 * Remember the configuration offered by a DHCP server. 00201 * 00202 * @param netif the netif under DHCP control 00203 */ 00204 static void 00205 dhcp_handle_offer(struct netif *netif) 00206 { 00207 struct dhcp *dhcp = netif->dhcp; 00208 /* obtain the server address */ 00209 u8_t *option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_SERVER_ID); 00210 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_handle_offer(netif=%p) %c%c%"U16_F"\n", 00211 (void*)netif, netif->name[0], netif->name[1], (u16_t)netif->num)); 00212 if (option_ptr != NULL) { 00213 dhcp->server_ip_addr.addr = htonl(dhcp_get_option_long(&option_ptr[2])); 00214 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_STATE, ("dhcp_handle_offer(): server 0x%08"X32_F"\n", dhcp->server_ip_addr.addr)); 00215 /* remember offered address */ 00216 ip_addr_set(&dhcp->offered_ip_addr, (struct ip_addr *)&dhcp->msg_in->yiaddr); 00217 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_STATE, ("dhcp_handle_offer(): offer for 0x%08"X32_F"\n", dhcp->offered_ip_addr.addr)); 00218 00219 dhcp_select(netif); 00220 } 00221 } 00222 00223 /** 00224 * Select a DHCP server offer out of all offers. 00225 * 00226 * Simply select the first offer received. 00227 * 00228 * @param netif the netif under DHCP control 00229 * @return lwIP specific error (see error.h) 00230 */ 00231 static err_t 00232 dhcp_select(struct netif *netif) 00233 { 00234 struct dhcp *dhcp = netif->dhcp; 00235 err_t result; 00236 u16_t msecs; 00237 #if LWIP_NETIF_HOSTNAME 00238 const char *p; 00239 #endif /* LWIP_NETIF_HOSTNAME */ 00240 00241 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_select(netif=%p) %c%c%"U16_F"\n", (void*)netif, netif->name[0], netif->name[1], (u16_t)netif->num)); 00242 00243 /* create and initialize the DHCP message header */ 00244 result = dhcp_create_request(netif); 00245 if (result == ERR_OK) { 00246 dhcp_option(dhcp, DHCP_OPTION_MESSAGE_TYPE, DHCP_OPTION_MESSAGE_TYPE_LEN); 00247 dhcp_option_byte(dhcp, DHCP_REQUEST); 00248 00249 dhcp_option(dhcp, DHCP_OPTION_MAX_MSG_SIZE, DHCP_OPTION_MAX_MSG_SIZE_LEN); 00250 dhcp_option_short(dhcp, 576); 00251 00252 /* MUST request the offered IP address */ 00253 dhcp_option(dhcp, DHCP_OPTION_REQUESTED_IP, 4); 00254 dhcp_option_long(dhcp, ntohl(dhcp->offered_ip_addr.addr)); 00255 00256 dhcp_option(dhcp, DHCP_OPTION_SERVER_ID, 4); 00257 dhcp_option_long(dhcp, ntohl(dhcp->server_ip_addr.addr)); 00258 00259 dhcp_option(dhcp, DHCP_OPTION_PARAMETER_REQUEST_LIST, 4/*num options*/); 00260 dhcp_option_byte(dhcp, DHCP_OPTION_SUBNET_MASK); 00261 dhcp_option_byte(dhcp, DHCP_OPTION_ROUTER); 00262 dhcp_option_byte(dhcp, DHCP_OPTION_BROADCAST); 00263 dhcp_option_byte(dhcp, DHCP_OPTION_DNS_SERVER); 00264 00265 #if LWIP_NETIF_HOSTNAME 00266 p = (const char*)netif->hostname; 00267 if (p!=NULL) { 00268 dhcp_option(dhcp, DHCP_OPTION_HOSTNAME, strlen(p)); 00269 while (*p) { 00270 dhcp_option_byte(dhcp, *p++); 00271 } 00272 } 00273 #endif /* LWIP_NETIF_HOSTNAME */ 00274 00275 dhcp_option_trailer(dhcp); 00276 /* shrink the pbuf to the actual content length */ 00277 pbuf_realloc(dhcp->p_out, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN + dhcp->options_out_len); 00278 00279 /* TODO: we really should bind to a specific local interface here 00280 but we cannot specify an unconfigured netif as it is addressless */ 00281 /* send broadcast to any DHCP server */ 00282 udp_sendto_if(dhcp->pcb, dhcp->p_out, IP_ADDR_BROADCAST, DHCP_SERVER_PORT, netif); 00283 /* reconnect to any (or to server here?!) */ 00284 udp_connect(dhcp->pcb, IP_ADDR_ANY, DHCP_SERVER_PORT); 00285 dhcp_delete_request(netif); 00286 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_select: REQUESTING\n")); 00287 dhcp_set_state(dhcp, DHCP_REQUESTING); 00288 } else { 00289 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_select: could not allocate DHCP request\n")); 00290 } 00291 dhcp->tries++; 00292 msecs = dhcp->tries < 4 ? dhcp->tries * 1000 : 4 * 1000; 00293 dhcp->request_timeout = (msecs + DHCP_FINE_TIMER_MSECS - 1) / DHCP_FINE_TIMER_MSECS; 00294 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_STATE, ("dhcp_select(): set request timeout %"U16_F" msecs\n", msecs)); 00295 return result; 00296 } 00297 00298 /** 00299 * The DHCP timer that checks for lease renewal/rebind timeouts. 00300 * 00301 */ 00302 void 00303 dhcp_coarse_tmr() 00304 { 00305 struct netif *netif = netif_list; 00306 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_coarse_tmr()\n")); 00307 /* iterate through all network interfaces */ 00308 while (netif != NULL) { 00309 /* only act on DHCP configured interfaces */ 00310 if (netif->dhcp != NULL) { 00311 /* timer is active (non zero), and triggers (zeroes) now? */ 00312 if (netif->dhcp->t2_timeout-- == 1) { 00313 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_coarse_tmr(): t2 timeout\n")); 00314 /* this clients' rebind timeout triggered */ 00315 dhcp_t2_timeout(netif); 00316 /* timer is active (non zero), and triggers (zeroes) now */ 00317 } else if (netif->dhcp->t1_timeout-- == 1) { 00318 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_coarse_tmr(): t1 timeout\n")); 00319 /* this clients' renewal timeout triggered */ 00320 dhcp_t1_timeout(netif); 00321 } 00322 } 00323 /* proceed to next netif */ 00324 netif = netif->next; 00325 } 00326 } 00327 00328 /** 00329 * DHCP transaction timeout handling 00330 * 00331 * A DHCP server is expected to respond within a short period of time. 00332 * This timer checks whether an outstanding DHCP request is timed out. 00333 * 00334 */ 00335 void 00336 dhcp_fine_tmr() 00337 { 00338 struct netif *netif = netif_list; 00339 /* loop through netif's */ 00340 while (netif != NULL) { 00341 /* only act on DHCP configured interfaces */ 00342 if (netif->dhcp != NULL) { 00343 /* timer is active (non zero), and is about to trigger now */ 00344 if (netif->dhcp->request_timeout > 1) { 00345 netif->dhcp->request_timeout--; 00346 } 00347 else if (netif->dhcp->request_timeout == 1) { 00348 netif->dhcp->request_timeout--; 00349 /* { netif->dhcp->request_timeout == 0 } */ 00350 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_fine_tmr(): request timeout\n")); 00351 /* this clients' request timeout triggered */ 00352 dhcp_timeout(netif); 00353 } 00354 } 00355 /* proceed to next network interface */ 00356 netif = netif->next; 00357 } 00358 } 00359 00360 /** 00361 * A DHCP negotiation transaction, or ARP request, has timed out. 00362 * 00363 * The timer that was started with the DHCP or ARP request has 00364 * timed out, indicating no response was received in time. 00365 * 00366 * @param netif the netif under DHCP control 00367 */ 00368 static void 00369 dhcp_timeout(struct netif *netif) 00370 { 00371 struct dhcp *dhcp = netif->dhcp; 00372 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_timeout()\n")); 00373 /* back-off period has passed, or server selection timed out */ 00374 if ((dhcp->state == DHCP_BACKING_OFF) || (dhcp->state == DHCP_SELECTING)) { 00375 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_timeout(): restarting discovery\n")); 00376 dhcp_discover(netif); 00377 /* receiving the requested lease timed out */ 00378 } else if (dhcp->state == DHCP_REQUESTING) { 00379 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_timeout(): REQUESTING, DHCP request timed out\n")); 00380 if (dhcp->tries <= 5) { 00381 dhcp_select(netif); 00382 } else { 00383 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_timeout(): REQUESTING, releasing, restarting\n")); 00384 dhcp_release(netif); 00385 dhcp_discover(netif); 00386 } 00387 /* received no ARP reply for the offered address (which is good) */ 00388 } else if (dhcp->state == DHCP_CHECKING) { 00389 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_timeout(): CHECKING, ARP request timed out\n")); 00390 if (dhcp->tries <= 1) { 00391 dhcp_check(netif); 00392 /* no ARP replies on the offered address, 00393 looks like the IP address is indeed free */ 00394 } else { 00395 /* bind the interface to the offered address */ 00396 dhcp_bind(netif); 00397 } 00398 } 00399 /* did not get response to renew request? */ 00400 else if (dhcp->state == DHCP_RENEWING) { 00401 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_timeout(): RENEWING, DHCP request timed out\n")); 00402 /* just retry renewal */ 00403 /* note that the rebind timer will eventually time-out if renew does not work */ 00404 dhcp_renew(netif); 00405 /* did not get response to rebind request? */ 00406 } else if (dhcp->state == DHCP_REBINDING) { 00407 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_timeout(): REBINDING, DHCP request timed out\n")); 00408 if (dhcp->tries <= 8) { 00409 dhcp_rebind(netif); 00410 } else { 00411 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_timeout(): RELEASING, DISCOVERING\n")); 00412 dhcp_release(netif); 00413 dhcp_discover(netif); 00414 } 00415 } 00416 } 00417 00418 /** 00419 * The renewal period has timed out. 00420 * 00421 * @param netif the netif under DHCP control 00422 */ 00423 static void 00424 dhcp_t1_timeout(struct netif *netif) 00425 { 00426 struct dhcp *dhcp = netif->dhcp; 00427 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_STATE, ("dhcp_t1_timeout()\n")); 00428 if ((dhcp->state == DHCP_REQUESTING) || (dhcp->state == DHCP_BOUND) || (dhcp->state == DHCP_RENEWING)) { 00429 /* just retry to renew - note that the rebind timer (t2) will 00430 * eventually time-out if renew tries fail. */ 00431 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_t1_timeout(): must renew\n")); 00432 dhcp_renew(netif); 00433 } 00434 } 00435 00436 /** 00437 * The rebind period has timed out. 00438 * 00439 * @param netif the netif under DHCP control 00440 */ 00441 static void 00442 dhcp_t2_timeout(struct netif *netif) 00443 { 00444 struct dhcp *dhcp = netif->dhcp; 00445 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_t2_timeout()\n")); 00446 if ((dhcp->state == DHCP_REQUESTING) || (dhcp->state == DHCP_BOUND) || (dhcp->state == DHCP_RENEWING)) { 00447 /* just retry to rebind */ 00448 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_t2_timeout(): must rebind\n")); 00449 dhcp_rebind(netif); 00450 } 00451 } 00452 00453 /** 00454 * Handle a DHCP ACK packet 00455 * 00456 * @param netif the netif under DHCP control 00457 */ 00458 static void 00459 dhcp_handle_ack(struct netif *netif) 00460 { 00461 struct dhcp *dhcp = netif->dhcp; 00462 u8_t *option_ptr; 00463 /* clear options we might not get from the ACK */ 00464 dhcp->offered_sn_mask.addr = 0; 00465 dhcp->offered_gw_addr.addr = 0; 00466 dhcp->offered_bc_addr.addr = 0; 00467 00468 /* lease time given? */ 00469 option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_LEASE_TIME); 00470 if (option_ptr != NULL) { 00471 /* remember offered lease time */ 00472 dhcp->offered_t0_lease = dhcp_get_option_long(option_ptr + 2); 00473 } 00474 /* renewal period given? */ 00475 option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_T1); 00476 if (option_ptr != NULL) { 00477 /* remember given renewal period */ 00478 dhcp->offered_t1_renew = dhcp_get_option_long(option_ptr + 2); 00479 } else { 00480 /* calculate safe periods for renewal */ 00481 dhcp->offered_t1_renew = dhcp->offered_t0_lease / 2; 00482 } 00483 00484 /* renewal period given? */ 00485 option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_T2); 00486 if (option_ptr != NULL) { 00487 /* remember given rebind period */ 00488 dhcp->offered_t2_rebind = dhcp_get_option_long(option_ptr + 2); 00489 } else { 00490 /* calculate safe periods for rebinding */ 00491 dhcp->offered_t2_rebind = dhcp->offered_t0_lease; 00492 } 00493 00494 /* (y)our internet address */ 00495 ip_addr_set(&dhcp->offered_ip_addr, &dhcp->msg_in->yiaddr); 00496 00497 /** 00498 * Patch #1308 00499 * TODO: we must check if the file field is not overloaded by DHCP options! 00500 */ 00501 #if 0 00502 /* boot server address */ 00503 ip_addr_set(&dhcp->offered_si_addr, &dhcp->msg_in->siaddr); 00504 /* boot file name */ 00505 if (dhcp->msg_in->file[0]) { 00506 dhcp->boot_file_name = mem_malloc(strlen(dhcp->msg_in->file) + 1); 00507 strcpy(dhcp->boot_file_name, dhcp->msg_in->file); 00508 } 00509 #endif 00510 00511 /* subnet mask */ 00512 option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_SUBNET_MASK); 00513 /* subnet mask given? */ 00514 if (option_ptr != NULL) { 00515 dhcp->offered_sn_mask.addr = htonl(dhcp_get_option_long(&option_ptr[2])); 00516 } 00517 00518 /* gateway router */ 00519 option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_ROUTER); 00520 if (option_ptr != NULL) { 00521 dhcp->offered_gw_addr.addr = htonl(dhcp_get_option_long(&option_ptr[2])); 00522 } 00523 00524 /* broadcast address */ 00525 option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_BROADCAST); 00526 if (option_ptr != NULL) { 00527 dhcp->offered_bc_addr.addr = htonl(dhcp_get_option_long(&option_ptr[2])); 00528 } 00529 00530 /* DNS servers */ 00531 option_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_DNS_SERVER); 00532 if (option_ptr != NULL) { 00533 u8_t n; 00534 dhcp->dns_count = dhcp_get_option_byte(&option_ptr[1]) / (u32_t)sizeof(struct ip_addr); 00535 /* limit to at most DHCP_MAX_DNS DNS servers */ 00536 if (dhcp->dns_count > DHCP_MAX_DNS) 00537 dhcp->dns_count = DHCP_MAX_DNS; 00538 for (n = 0; n < dhcp->dns_count; n++) { 00539 dhcp->offered_dns_addr[n].addr = htonl(dhcp_get_option_long(&option_ptr[2 + n * 4])); 00540 #if LWIP_DNS 00541 dns_setserver( n, (struct ip_addr *)(&(dhcp->offered_dns_addr[n].addr))); 00542 #endif /* LWIP_DNS */ 00543 } 00544 #if LWIP_DNS 00545 dns_setserver( n, (struct ip_addr *)(&ip_addr_any)); 00546 #endif /* LWIP_DNS */ 00547 } 00548 } 00549 00550 /** 00551 * Start DHCP negotiation for a network interface. 00552 * 00553 * If no DHCP client instance was attached to this interface, 00554 * a new client is created first. If a DHCP client instance 00555 * was already present, it restarts negotiation. 00556 * 00557 * @param netif The lwIP network interface 00558 * @return lwIP error code 00559 * - ERR_OK - No error 00560 * - ERR_MEM - Out of memory 00561 */ 00562 err_t 00563 dhcp_start(struct netif *netif) 00564 { 00565 struct dhcp *dhcp; 00566 err_t result = ERR_OK; 00567 00568 LWIP_ERROR("netif != NULL", (netif != NULL), return ERR_ARG;); 00569 dhcp = netif->dhcp; 00570 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_start(netif=%p) %c%c%"U16_F"\n", (void*)netif, netif->name[0], netif->name[1], (u16_t)netif->num)); 00571 netif->flags &= ~NETIF_FLAG_DHCP; 00572 00573 /* no DHCP client attached yet? */ 00574 if (dhcp == NULL) { 00575 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_start(): starting new DHCP client\n")); 00576 dhcp = (struct dhcp *)mem_malloc(sizeof(struct dhcp)); // static_cast<struct dhcp *>(x) 00577 if (dhcp == NULL) { 00578 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_start(): could not allocate dhcp\n")); 00579 return ERR_MEM; 00580 } 00581 /* store this dhcp client in the netif */ 00582 netif->dhcp = dhcp; 00583 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_start(): allocated dhcp")); 00584 /* already has DHCP client attached */ 00585 } else { 00586 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 3, ("dhcp_start(): restarting DHCP configuration\n")); 00587 } 00588 00589 /* clear data structure */ 00590 memset(dhcp, 0, sizeof(struct dhcp)); 00591 /* allocate UDP PCB */ 00592 dhcp->pcb = udp_new(); 00593 if (dhcp->pcb == NULL) { 00594 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_start(): could not obtain pcb\n")); 00595 mem_free((void *)dhcp); 00596 netif->dhcp = dhcp = NULL; 00597 return ERR_MEM; 00598 } 00599 /* set up local and remote port for the pcb */ 00600 udp_bind(dhcp->pcb, IP_ADDR_ANY, DHCP_CLIENT_PORT); 00601 udp_connect(dhcp->pcb, IP_ADDR_ANY, DHCP_SERVER_PORT); 00602 /* set up the recv callback and argument */ 00603 udp_recv(dhcp->pcb, dhcp_recv, netif); 00604 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_start(): starting DHCP configuration\n")); 00605 /* (re)start the DHCP negotiation */ 00606 result = dhcp_discover(netif); 00607 if (result != ERR_OK) { 00608 /* free resources allocated above */ 00609 dhcp_stop(netif); 00610 return ERR_MEM; 00611 } 00612 netif->flags |= NETIF_FLAG_DHCP; 00613 return result; 00614 } 00615 00616 /** 00617 * Inform a DHCP server of our manual configuration. 00618 * 00619 * This informs DHCP servers of our fixed IP address configuration 00620 * by sending an INFORM message. It does not involve DHCP address 00621 * configuration, it is just here to be nice to the network. 00622 * 00623 * @param netif The lwIP network interface 00624 */ 00625 void 00626 dhcp_inform(struct netif *netif) 00627 { 00628 struct dhcp *dhcp, *old_dhcp = netif->dhcp; 00629 err_t result = ERR_OK; 00630 dhcp = (struct dhcp *)mem_malloc(sizeof(struct dhcp)); // static_cast<struct dhcp *>(x) 00631 if (dhcp == NULL) { 00632 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_inform(): could not allocate dhcp\n")); 00633 return; 00634 } 00635 netif->dhcp = dhcp; 00636 memset(dhcp, 0, sizeof(struct dhcp)); 00637 00638 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_inform(): allocated dhcp\n")); 00639 dhcp->pcb = udp_new(); 00640 if (dhcp->pcb == NULL) { 00641 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_inform(): could not obtain pcb")); 00642 mem_free((void *)dhcp); 00643 return; 00644 } 00645 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_inform(): created new udp pcb\n")); 00646 /* create and initialize the DHCP message header */ 00647 result = dhcp_create_request(netif); 00648 if (result == ERR_OK) { 00649 00650 dhcp_option(dhcp, DHCP_OPTION_MESSAGE_TYPE, DHCP_OPTION_MESSAGE_TYPE_LEN); 00651 dhcp_option_byte(dhcp, DHCP_INFORM); 00652 00653 dhcp_option(dhcp, DHCP_OPTION_MAX_MSG_SIZE, DHCP_OPTION_MAX_MSG_SIZE_LEN); 00654 /* TODO: use netif->mtu ?! */ 00655 dhcp_option_short(dhcp, 576); 00656 00657 dhcp_option_trailer(dhcp); 00658 00659 pbuf_realloc(dhcp->p_out, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN + dhcp->options_out_len); 00660 00661 udp_bind(dhcp->pcb, IP_ADDR_ANY, DHCP_CLIENT_PORT); 00662 udp_connect(dhcp->pcb, IP_ADDR_BROADCAST, DHCP_SERVER_PORT); 00663 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_inform: INFORMING\n")); 00664 udp_sendto_if(dhcp->pcb, dhcp->p_out, IP_ADDR_BROADCAST, DHCP_SERVER_PORT, netif); 00665 udp_connect(dhcp->pcb, IP_ADDR_ANY, DHCP_SERVER_PORT); 00666 dhcp_delete_request(netif); 00667 } else { 00668 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_inform: could not allocate DHCP request\n")); 00669 } 00670 00671 if (dhcp != NULL) { 00672 if (dhcp->pcb != NULL) { 00673 udp_remove(dhcp->pcb); 00674 } 00675 dhcp->pcb = NULL; 00676 mem_free((void *)dhcp); 00677 netif->dhcp = old_dhcp; 00678 } 00679 } 00680 00681 #if DHCP_DOES_ARP_CHECK 00682 /** 00683 * Match an ARP reply with the offered IP address. 00684 * 00685 * @param netif the network interface on which the reply was received 00686 * @param addr The IP address we received a reply from 00687 */ 00688 void dhcp_arp_reply(struct netif *netif, struct ip_addr *addr) 00689 { 00690 LWIP_ERROR("netif != NULL", (netif != NULL), return;); 00691 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_arp_reply()\n")); 00692 /* is a DHCP client doing an ARP check? */ 00693 if ((netif->dhcp != NULL) && (netif->dhcp->state == DHCP_CHECKING)) { 00694 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_arp_reply(): CHECKING, arp reply for 0x%08"X32_F"\n", addr->addr)); 00695 /* did a host respond with the address we 00696 were offered by the DHCP server? */ 00697 if (ip_addr_cmp(addr, &netif->dhcp->offered_ip_addr)) { 00698 /* we will not accept the offered address */ 00699 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 1, ("dhcp_arp_reply(): arp reply matched with offered address, declining\n")); 00700 dhcp_decline(netif); 00701 } 00702 } 00703 } 00704 00705 /** 00706 * Decline an offered lease. 00707 * 00708 * Tell the DHCP server we do not accept the offered address. 00709 * One reason to decline the lease is when we find out the address 00710 * is already in use by another host (through ARP). 00711 * 00712 * @param netif the netif under DHCP control 00713 */ 00714 static err_t 00715 dhcp_decline(struct netif *netif) 00716 { 00717 struct dhcp *dhcp = netif->dhcp; 00718 err_t result = ERR_OK; 00719 u16_t msecs; 00720 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_decline()\n")); 00721 dhcp_set_state(dhcp, DHCP_BACKING_OFF); 00722 /* create and initialize the DHCP message header */ 00723 result = dhcp_create_request(netif); 00724 if (result == ERR_OK) { 00725 dhcp_option(dhcp, DHCP_OPTION_MESSAGE_TYPE, DHCP_OPTION_MESSAGE_TYPE_LEN); 00726 dhcp_option_byte(dhcp, DHCP_DECLINE); 00727 00728 dhcp_option(dhcp, DHCP_OPTION_MAX_MSG_SIZE, DHCP_OPTION_MAX_MSG_SIZE_LEN); 00729 dhcp_option_short(dhcp, 576); 00730 00731 dhcp_option(dhcp, DHCP_OPTION_REQUESTED_IP, 4); 00732 dhcp_option_long(dhcp, ntohl(dhcp->offered_ip_addr.addr)); 00733 00734 dhcp_option_trailer(dhcp); 00735 /* resize pbuf to reflect true size of options */ 00736 pbuf_realloc(dhcp->p_out, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN + dhcp->options_out_len); 00737 00738 /* @todo: should we really connect here? we are performing sendto() */ 00739 udp_connect(dhcp->pcb, IP_ADDR_ANY, DHCP_SERVER_PORT); 00740 /* per section 4.4.4, broadcast DECLINE messages */ 00741 udp_sendto_if(dhcp->pcb, dhcp->p_out, IP_ADDR_BROADCAST, DHCP_SERVER_PORT, netif); 00742 dhcp_delete_request(netif); 00743 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_decline: BACKING OFF\n")); 00744 } else { 00745 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_decline: could not allocate DHCP request\n")); 00746 } 00747 dhcp->tries++; 00748 msecs = 10*1000; 00749 dhcp->request_timeout = (msecs + DHCP_FINE_TIMER_MSECS - 1) / DHCP_FINE_TIMER_MSECS; 00750 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_decline(): set request timeout %"U16_F" msecs\n", msecs)); 00751 return result; 00752 } 00753 #endif 00754 00755 00756 /** 00757 * Start the DHCP process, discover a DHCP server. 00758 * 00759 * @param netif the netif under DHCP control 00760 */ 00761 static err_t 00762 dhcp_discover(struct netif *netif) 00763 { 00764 struct dhcp *dhcp = netif->dhcp; 00765 err_t result = ERR_OK; 00766 u16_t msecs; 00767 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_discover()\n")); 00768 ip_addr_set(&dhcp->offered_ip_addr, IP_ADDR_ANY); 00769 /* create and initialize the DHCP message header */ 00770 result = dhcp_create_request(netif); 00771 if (result == ERR_OK) { 00772 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_discover: making request\n")); 00773 dhcp_option(dhcp, DHCP_OPTION_MESSAGE_TYPE, DHCP_OPTION_MESSAGE_TYPE_LEN); 00774 dhcp_option_byte(dhcp, DHCP_DISCOVER); 00775 00776 dhcp_option(dhcp, DHCP_OPTION_MAX_MSG_SIZE, DHCP_OPTION_MAX_MSG_SIZE_LEN); 00777 dhcp_option_short(dhcp, 576); 00778 00779 dhcp_option(dhcp, DHCP_OPTION_PARAMETER_REQUEST_LIST, 4/*num options*/); 00780 dhcp_option_byte(dhcp, DHCP_OPTION_SUBNET_MASK); 00781 dhcp_option_byte(dhcp, DHCP_OPTION_ROUTER); 00782 dhcp_option_byte(dhcp, DHCP_OPTION_BROADCAST); 00783 dhcp_option_byte(dhcp, DHCP_OPTION_DNS_SERVER); 00784 00785 dhcp_option_trailer(dhcp); 00786 00787 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_discover: realloc()ing\n")); 00788 pbuf_realloc(dhcp->p_out, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN + dhcp->options_out_len); 00789 00790 udp_connect(dhcp->pcb, IP_ADDR_ANY, DHCP_SERVER_PORT); 00791 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_discover: sendto(DISCOVER, IP_ADDR_BROADCAST, DHCP_SERVER_PORT)\n")); 00792 udp_sendto_if(dhcp->pcb, dhcp->p_out, IP_ADDR_BROADCAST, DHCP_SERVER_PORT, netif); 00793 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_discover: deleting()ing\n")); 00794 dhcp_delete_request(netif); 00795 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_discover: SELECTING\n")); 00796 dhcp_set_state(dhcp, DHCP_SELECTING); 00797 } else { 00798 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_discover: could not allocate DHCP request\n")); 00799 } 00800 dhcp->tries++; 00801 #if LWIP_DHCP_AUTOIP_COOP 00802 /* that means we waited 57 seconds */ 00803 if(dhcp->tries >= 9 && dhcp->autoip_coop_state == DHCP_AUTOIP_COOP_STATE_OFF) { 00804 dhcp->autoip_coop_state = DHCP_AUTOIP_COOP_STATE_ON; 00805 autoip_start(netif); 00806 } 00807 #endif /* LWIP_DHCP_AUTOIP_COOP */ 00808 msecs = dhcp->tries < 4 ? (dhcp->tries + 1) * 1000 : 10 * 1000; 00809 dhcp->request_timeout = (msecs + DHCP_FINE_TIMER_MSECS - 1) / DHCP_FINE_TIMER_MSECS; 00810 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_discover(): set request timeout %"U16_F" msecs\n", msecs)); 00811 return result; 00812 } 00813 00814 00815 /** 00816 * Bind the interface to the offered IP address. 00817 * 00818 * @param netif network interface to bind to the offered address 00819 */ 00820 static void 00821 dhcp_bind(struct netif *netif) 00822 { 00823 u32_t timeout; 00824 struct dhcp *dhcp; 00825 struct ip_addr sn_mask, gw_addr; 00826 LWIP_ERROR("dhcp_bind: netif != NULL", (netif != NULL), return;); 00827 dhcp = netif->dhcp; 00828 LWIP_ERROR("dhcp_bind: dhcp != NULL", (dhcp != NULL), return;); 00829 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_bind(netif=%p) %c%c%"U16_F"\n", (void*)netif, netif->name[0], netif->name[1], (u16_t)netif->num)); 00830 00831 /* temporary DHCP lease? */ 00832 if (dhcp->offered_t1_renew != 0xffffffffUL) { 00833 /* set renewal period timer */ 00834 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_bind(): t1 renewal timer %"U32_F" secs\n", dhcp->offered_t1_renew)); 00835 timeout = (dhcp->offered_t1_renew + DHCP_COARSE_TIMER_SECS / 2) / DHCP_COARSE_TIMER_SECS; 00836 if(timeout > 0xffff) { 00837 timeout = 0xffff; 00838 } 00839 dhcp->t1_timeout = (u16_t)timeout; 00840 if (dhcp->t1_timeout == 0) { 00841 dhcp->t1_timeout = 1; 00842 } 00843 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_bind(): set request timeout %"U32_F" msecs\n", dhcp->offered_t1_renew*1000)); 00844 } 00845 /* set renewal period timer */ 00846 if (dhcp->offered_t2_rebind != 0xffffffffUL) { 00847 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_bind(): t2 rebind timer %"U32_F" secs\n", dhcp->offered_t2_rebind)); 00848 timeout = (dhcp->offered_t2_rebind + DHCP_COARSE_TIMER_SECS / 2) / DHCP_COARSE_TIMER_SECS; 00849 if(timeout > 0xffff) { 00850 timeout = 0xffff; 00851 } 00852 dhcp->t2_timeout = (u16_t)timeout; 00853 if (dhcp->t2_timeout == 0) { 00854 dhcp->t2_timeout = 1; 00855 } 00856 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_bind(): set request timeout %"U32_F" msecs\n", dhcp->offered_t2_rebind*1000)); 00857 } 00858 /* copy offered network mask */ 00859 ip_addr_set(&sn_mask, &dhcp->offered_sn_mask); 00860 00861 /* subnet mask not given? */ 00862 /* TODO: this is not a valid check. what if the network mask is 0? */ 00863 if (sn_mask.addr == 0) { 00864 /* choose a safe subnet mask given the network class */ 00865 u8_t first_octet = ip4_addr1(&sn_mask); 00866 if (first_octet <= 127) { 00867 sn_mask.addr = htonl(0xff000000); 00868 } else if (first_octet >= 192) { 00869 sn_mask.addr = htonl(0xffffff00); 00870 } else { 00871 sn_mask.addr = htonl(0xffff0000); 00872 } 00873 } 00874 00875 ip_addr_set(&gw_addr, &dhcp->offered_gw_addr); 00876 /* gateway address not given? */ 00877 if (gw_addr.addr == 0) { 00878 /* copy network address */ 00879 gw_addr.addr = (dhcp->offered_ip_addr.addr & sn_mask.addr); 00880 /* use first host address on network as gateway */ 00881 gw_addr.addr |= htonl(0x00000001); 00882 } 00883 00884 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_STATE, ("dhcp_bind(): IP: 0x%08"X32_F"\n", dhcp->offered_ip_addr.addr)); 00885 netif_set_ipaddr(netif, &dhcp->offered_ip_addr); 00886 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_STATE, ("dhcp_bind(): SN: 0x%08"X32_F"\n", sn_mask.addr)); 00887 netif_set_netmask(netif, &sn_mask); 00888 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_STATE, ("dhcp_bind(): GW: 0x%08"X32_F"\n", gw_addr.addr)); 00889 netif_set_gw(netif, &gw_addr); 00890 /* bring the interface up */ 00891 netif_set_up(netif); 00892 /* netif is now bound to DHCP leased address */ 00893 dhcp_set_state(dhcp, DHCP_BOUND); 00894 } 00895 00896 /** 00897 * Renew an existing DHCP lease at the involved DHCP server. 00898 * 00899 * @param netif network interface which must renew its lease 00900 */ 00901 err_t 00902 dhcp_renew(struct netif *netif) 00903 { 00904 struct dhcp *dhcp = netif->dhcp; 00905 err_t result; 00906 u16_t msecs; 00907 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_renew()\n")); 00908 dhcp_set_state(dhcp, DHCP_RENEWING); 00909 00910 /* create and initialize the DHCP message header */ 00911 result = dhcp_create_request(netif); 00912 if (result == ERR_OK) { 00913 00914 dhcp_option(dhcp, DHCP_OPTION_MESSAGE_TYPE, DHCP_OPTION_MESSAGE_TYPE_LEN); 00915 dhcp_option_byte(dhcp, DHCP_REQUEST); 00916 00917 dhcp_option(dhcp, DHCP_OPTION_MAX_MSG_SIZE, DHCP_OPTION_MAX_MSG_SIZE_LEN); 00918 /* TODO: use netif->mtu in some way */ 00919 dhcp_option_short(dhcp, 576); 00920 00921 #if 0 00922 dhcp_option(dhcp, DHCP_OPTION_REQUESTED_IP, 4); 00923 dhcp_option_long(dhcp, ntohl(dhcp->offered_ip_addr.addr)); 00924 #endif 00925 00926 #if 0 00927 dhcp_option(dhcp, DHCP_OPTION_SERVER_ID, 4); 00928 dhcp_option_long(dhcp, ntohl(dhcp->server_ip_addr.addr)); 00929 #endif 00930 /* append DHCP message trailer */ 00931 dhcp_option_trailer(dhcp); 00932 00933 pbuf_realloc(dhcp->p_out, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN + dhcp->options_out_len); 00934 00935 udp_connect(dhcp->pcb, &dhcp->server_ip_addr, DHCP_SERVER_PORT); 00936 udp_sendto_if(dhcp->pcb, dhcp->p_out, &dhcp->server_ip_addr, DHCP_SERVER_PORT, netif); 00937 dhcp_delete_request(netif); 00938 00939 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_renew: RENEWING\n")); 00940 } else { 00941 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_renew: could not allocate DHCP request\n")); 00942 } 00943 dhcp->tries++; 00944 /* back-off on retries, but to a maximum of 20 seconds */ 00945 msecs = dhcp->tries < 10 ? dhcp->tries * 2000 : 20 * 1000; 00946 dhcp->request_timeout = (msecs + DHCP_FINE_TIMER_MSECS - 1) / DHCP_FINE_TIMER_MSECS; 00947 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_renew(): set request timeout %"U16_F" msecs\n", msecs)); 00948 return result; 00949 } 00950 00951 /** 00952 * Rebind with a DHCP server for an existing DHCP lease. 00953 * 00954 * @param netif network interface which must rebind with a DHCP server 00955 */ 00956 static err_t 00957 dhcp_rebind(struct netif *netif) 00958 { 00959 struct dhcp *dhcp = netif->dhcp; 00960 err_t result; 00961 u16_t msecs; 00962 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_rebind()\n")); 00963 dhcp_set_state(dhcp, DHCP_REBINDING); 00964 00965 /* create and initialize the DHCP message header */ 00966 result = dhcp_create_request(netif); 00967 if (result == ERR_OK) { 00968 00969 dhcp_option(dhcp, DHCP_OPTION_MESSAGE_TYPE, DHCP_OPTION_MESSAGE_TYPE_LEN); 00970 dhcp_option_byte(dhcp, DHCP_REQUEST); 00971 00972 dhcp_option(dhcp, DHCP_OPTION_MAX_MSG_SIZE, DHCP_OPTION_MAX_MSG_SIZE_LEN); 00973 dhcp_option_short(dhcp, 576); 00974 00975 #if 0 00976 dhcp_option(dhcp, DHCP_OPTION_REQUESTED_IP, 4); 00977 dhcp_option_long(dhcp, ntohl(dhcp->offered_ip_addr.addr)); 00978 00979 dhcp_option(dhcp, DHCP_OPTION_SERVER_ID, 4); 00980 dhcp_option_long(dhcp, ntohl(dhcp->server_ip_addr.addr)); 00981 #endif 00982 00983 dhcp_option_trailer(dhcp); 00984 00985 pbuf_realloc(dhcp->p_out, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN + dhcp->options_out_len); 00986 00987 /* broadcast to server */ 00988 udp_connect(dhcp->pcb, IP_ADDR_ANY, DHCP_SERVER_PORT); 00989 udp_sendto_if(dhcp->pcb, dhcp->p_out, IP_ADDR_BROADCAST, DHCP_SERVER_PORT, netif); 00990 dhcp_delete_request(netif); 00991 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_rebind: REBINDING\n")); 00992 } else { 00993 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_rebind: could not allocate DHCP request\n")); 00994 } 00995 dhcp->tries++; 00996 msecs = dhcp->tries < 10 ? dhcp->tries * 1000 : 10 * 1000; 00997 dhcp->request_timeout = (msecs + DHCP_FINE_TIMER_MSECS - 1) / DHCP_FINE_TIMER_MSECS; 00998 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_rebind(): set request timeout %"U16_F" msecs\n", msecs)); 00999 return result; 01000 } 01001 01002 /** 01003 * Release a DHCP lease. 01004 * 01005 * @param netif network interface which must release its lease 01006 */ 01007 err_t 01008 dhcp_release(struct netif *netif) 01009 { 01010 struct dhcp *dhcp = netif->dhcp; 01011 err_t result; 01012 u16_t msecs; 01013 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_release()\n")); 01014 01015 /* idle DHCP client */ 01016 dhcp_set_state(dhcp, DHCP_OFF); 01017 /* clean old DHCP offer */ 01018 dhcp->server_ip_addr.addr = 0; 01019 dhcp->offered_ip_addr.addr = dhcp->offered_sn_mask.addr = 0; 01020 dhcp->offered_gw_addr.addr = dhcp->offered_bc_addr.addr = 0; 01021 dhcp->offered_t0_lease = dhcp->offered_t1_renew = dhcp->offered_t2_rebind = 0; 01022 dhcp->dns_count = 0; 01023 01024 /* create and initialize the DHCP message header */ 01025 result = dhcp_create_request(netif); 01026 if (result == ERR_OK) { 01027 dhcp_option(dhcp, DHCP_OPTION_MESSAGE_TYPE, DHCP_OPTION_MESSAGE_TYPE_LEN); 01028 dhcp_option_byte(dhcp, DHCP_RELEASE); 01029 01030 dhcp_option_trailer(dhcp); 01031 01032 pbuf_realloc(dhcp->p_out, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN + dhcp->options_out_len); 01033 01034 udp_connect(dhcp->pcb, &dhcp->server_ip_addr, DHCP_SERVER_PORT); 01035 udp_sendto_if(dhcp->pcb, dhcp->p_out, &dhcp->server_ip_addr, DHCP_SERVER_PORT, netif); 01036 dhcp_delete_request(netif); 01037 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_release: RELEASED, DHCP_OFF\n")); 01038 } else { 01039 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_release: could not allocate DHCP request\n")); 01040 } 01041 dhcp->tries++; 01042 msecs = dhcp->tries < 10 ? dhcp->tries * 1000 : 10 * 1000; 01043 dhcp->request_timeout = (msecs + DHCP_FINE_TIMER_MSECS - 1) / DHCP_FINE_TIMER_MSECS; 01044 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, ("dhcp_release(): set request timeout %"U16_F" msecs\n", msecs)); 01045 /* bring the interface down */ 01046 netif_set_down(netif); 01047 /* remove IP address from interface */ 01048 netif_set_ipaddr(netif, IP_ADDR_ANY); 01049 netif_set_gw(netif, IP_ADDR_ANY); 01050 netif_set_netmask(netif, IP_ADDR_ANY); 01051 01052 /* TODO: netif_down(netif); */ 01053 return result; 01054 } 01055 01056 /** 01057 * Remove the DHCP client from the interface. 01058 * 01059 * @param netif The network interface to stop DHCP on 01060 */ 01061 void 01062 dhcp_stop(struct netif *netif) 01063 { 01064 struct dhcp *dhcp = netif->dhcp; 01065 LWIP_ERROR("dhcp_stop: netif != NULL", (netif != NULL), return;); 01066 01067 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_stop()\n")); 01068 /* netif is DHCP configured? */ 01069 if (dhcp != NULL) { 01070 if (dhcp->pcb != NULL) { 01071 udp_remove(dhcp->pcb); 01072 dhcp->pcb = NULL; 01073 } 01074 if (dhcp->p != NULL) { 01075 pbuf_free(dhcp->p); 01076 dhcp->p = NULL; 01077 } 01078 /* free unfolded reply */ 01079 dhcp_free_reply(dhcp); 01080 mem_free((void *)dhcp); 01081 netif->dhcp = NULL; 01082 } 01083 } 01084 01085 /* 01086 * Set the DHCP state of a DHCP client. 01087 * 01088 * If the state changed, reset the number of tries. 01089 * 01090 * TODO: we might also want to reset the timeout here? 01091 */ 01092 static void 01093 dhcp_set_state(struct dhcp *dhcp, u8_t new_state) 01094 { 01095 if (new_state != dhcp->state) { 01096 dhcp->state = new_state; 01097 dhcp->tries = 0; 01098 } 01099 } 01100 01101 /* 01102 * Concatenate an option type and length field to the outgoing 01103 * DHCP message. 01104 * 01105 */ 01106 static void 01107 dhcp_option(struct dhcp *dhcp, u8_t option_type, u8_t option_len) 01108 { 01109 LWIP_ASSERT("dhcp_option: dhcp->options_out_len + 2 + option_len <= DHCP_OPTIONS_LEN", dhcp->options_out_len + 2U + option_len <= DHCP_OPTIONS_LEN); 01110 dhcp->msg_out->options[dhcp->options_out_len++] = option_type; 01111 dhcp->msg_out->options[dhcp->options_out_len++] = option_len; 01112 } 01113 /* 01114 * Concatenate a single byte to the outgoing DHCP message. 01115 * 01116 */ 01117 static void 01118 dhcp_option_byte(struct dhcp *dhcp, u8_t value) 01119 { 01120 LWIP_ASSERT("dhcp_option_byte: dhcp->options_out_len < DHCP_OPTIONS_LEN", dhcp->options_out_len < DHCP_OPTIONS_LEN); 01121 dhcp->msg_out->options[dhcp->options_out_len++] = value; 01122 } 01123 01124 static void 01125 dhcp_option_short(struct dhcp *dhcp, u16_t value) 01126 { 01127 LWIP_ASSERT("dhcp_option_short: dhcp->options_out_len + 2 <= DHCP_OPTIONS_LEN", dhcp->options_out_len + 2U <= DHCP_OPTIONS_LEN); 01128 dhcp->msg_out->options[dhcp->options_out_len++] = (u8_t)((value & 0xff00U) >> 8); 01129 dhcp->msg_out->options[dhcp->options_out_len++] = (u8_t) (value & 0x00ffU); 01130 } 01131 01132 static void 01133 dhcp_option_long(struct dhcp *dhcp, u32_t value) 01134 { 01135 LWIP_ASSERT("dhcp_option_long: dhcp->options_out_len + 4 <= DHCP_OPTIONS_LEN", dhcp->options_out_len + 4U <= DHCP_OPTIONS_LEN); 01136 dhcp->msg_out->options[dhcp->options_out_len++] = (u8_t)((value & 0xff000000UL) >> 24); 01137 dhcp->msg_out->options[dhcp->options_out_len++] = (u8_t)((value & 0x00ff0000UL) >> 16); 01138 dhcp->msg_out->options[dhcp->options_out_len++] = (u8_t)((value & 0x0000ff00UL) >> 8); 01139 dhcp->msg_out->options[dhcp->options_out_len++] = (u8_t)((value & 0x000000ffUL)); 01140 } 01141 01142 /** 01143 * Extract the DHCP message and the DHCP options. 01144 * 01145 * Extract the DHCP message and the DHCP options, each into a contiguous 01146 * piece of memory. As a DHCP message is variable sized by its options, 01147 * and also allows overriding some fields for options, the easy approach 01148 * is to first unfold the options into a conitguous piece of memory, and 01149 * use that further on. 01150 * 01151 */ 01152 static err_t 01153 dhcp_unfold_reply(struct dhcp *dhcp) 01154 { 01155 u16_t ret; 01156 LWIP_ERROR("dhcp != NULL", (dhcp != NULL), return ERR_ARG;); 01157 LWIP_ERROR("dhcp->p != NULL", (dhcp->p != NULL), return ERR_VAL;); 01158 /* free any left-overs from previous unfolds */ 01159 dhcp_free_reply(dhcp); 01160 /* options present? */ 01161 if (dhcp->p->tot_len > (sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN)) { 01162 dhcp->options_in_len = dhcp->p->tot_len - (sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN); 01163 dhcp->options_in = (struct dhcp_msg *)mem_malloc(dhcp->options_in_len); // static_cast<struct dhcp_msg *>(x) 01164 if (dhcp->options_in == NULL) { 01165 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_unfold_reply(): could not allocate dhcp->options\n")); 01166 return ERR_MEM; 01167 } 01168 } 01169 dhcp->msg_in = (struct dhcp_msg *)mem_malloc(sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN); // static_cast<struct dhcp_msg *>(x) 01170 if (dhcp->msg_in == NULL) { 01171 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_unfold_reply(): could not allocate dhcp->msg_in\n")); 01172 mem_free((void *)dhcp->options_in); 01173 dhcp->options_in = NULL; 01174 return ERR_MEM; 01175 } 01176 01177 /** copy the DHCP message without options */ 01178 ret = pbuf_copy_partial(dhcp->p, dhcp->msg_in, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN, 0); 01179 LWIP_ASSERT("ret == sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN", ret == sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN); 01180 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_unfold_reply(): copied %"U16_F" bytes into dhcp->msg_in[]\n", 01181 sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN)); 01182 01183 if (dhcp->options_in != NULL) { 01184 /** copy the DHCP options */ 01185 ret = pbuf_copy_partial(dhcp->p, dhcp->options_in, dhcp->options_in_len, sizeof(struct dhcp_msg) - DHCP_OPTIONS_LEN); 01186 LWIP_ASSERT("ret == dhcp->options_in_len", ret == dhcp->options_in_len); 01187 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("dhcp_unfold_reply(): copied %"U16_F" bytes to dhcp->options_in[]\n", 01188 dhcp->options_in_len)); 01189 } 01190 LWIP_UNUSED_ARG(ret); 01191 return ERR_OK; 01192 } 01193 01194 /** 01195 * Free the incoming DHCP message including contiguous copy of 01196 * its DHCP options. 01197 * 01198 */ 01199 static void dhcp_free_reply(struct dhcp *dhcp) 01200 { 01201 if (dhcp->msg_in != NULL) { 01202 mem_free((void *)dhcp->msg_in); 01203 dhcp->msg_in = NULL; 01204 } 01205 if (dhcp->options_in) { 01206 mem_free((void *)dhcp->options_in); 01207 dhcp->options_in = NULL; 01208 dhcp->options_in_len = 0; 01209 } 01210 LWIP_DEBUGF(DHCP_DEBUG, ("dhcp_free_reply(): free'd\n")); 01211 } 01212 01213 01214 /** 01215 * If an incoming DHCP message is in response to us, then trigger the state machine 01216 */ 01217 static void dhcp_recv(void *arg, struct udp_pcb *pcb, struct pbuf *p, struct ip_addr *addr, u16_t port) 01218 { 01219 struct netif *netif = (struct netif *)arg; 01220 struct dhcp *dhcp = netif->dhcp; 01221 struct dhcp_msg *reply_msg = (struct dhcp_msg *)p->payload; 01222 u8_t *options_ptr; 01223 u8_t msg_type; 01224 u8_t i; 01225 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 3, ("dhcp_recv(pbuf = %p) from DHCP server %"U16_F".%"U16_F".%"U16_F".%"U16_F" port %"U16_F"\n", (void*)p, 01226 (u16_t)(ntohl(addr->addr) >> 24 & 0xff), (u16_t)(ntohl(addr->addr) >> 16 & 0xff), 01227 (u16_t)(ntohl(addr->addr) >> 8 & 0xff), (u16_t)(ntohl(addr->addr) & 0xff), port)); 01228 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("pbuf->len = %"U16_F"\n", p->len)); 01229 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("pbuf->tot_len = %"U16_F"\n", p->tot_len)); 01230 /* prevent warnings about unused arguments */ 01231 LWIP_UNUSED_ARG(pcb); 01232 LWIP_UNUSED_ARG(addr); 01233 LWIP_UNUSED_ARG(port); 01234 dhcp->p = p; 01235 /* TODO: check packet length before reading them */ 01236 if (reply_msg->op != DHCP_BOOTREPLY) { 01237 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("not a DHCP reply message, but type %"U16_F"\n", (u16_t)reply_msg->op)); 01238 goto free_pbuf_and_return; 01239 } 01240 /* iterate through hardware address and match against DHCP message */ 01241 for (i = 0; i < netif->hwaddr_len; i++) { 01242 if (netif->hwaddr[i] != reply_msg->chaddr[i]) { 01243 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("netif->hwaddr[%"U16_F"]==%02"X16_F" != reply_msg->chaddr[%"U16_F"]==%02"X16_F"\n", 01244 (u16_t)i, (u16_t)netif->hwaddr[i], (u16_t)i, (u16_t)reply_msg->chaddr[i])); 01245 goto free_pbuf_and_return; 01246 } 01247 } 01248 /* match transaction ID against what we expected */ 01249 if (ntohl(reply_msg->xid) != dhcp->xid) { 01250 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("transaction id mismatch reply_msg->xid(%"X32_F")!=dhcp->xid(%"X32_F")\n",ntohl(reply_msg->xid),dhcp->xid)); 01251 goto free_pbuf_and_return; 01252 } 01253 /* option fields could be unfold? */ 01254 if (dhcp_unfold_reply(dhcp) != ERR_OK) { 01255 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("problem unfolding DHCP message - too short on memory?\n")); 01256 goto free_pbuf_and_return; 01257 } 01258 01259 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("searching DHCP_OPTION_MESSAGE_TYPE\n")); 01260 /* obtain pointer to DHCP message type */ 01261 options_ptr = dhcp_get_option_ptr(dhcp, DHCP_OPTION_MESSAGE_TYPE); 01262 if (options_ptr == NULL) { 01263 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("DHCP_OPTION_MESSAGE_TYPE option not found\n")); 01264 goto free_pbuf_and_return; 01265 } 01266 01267 /* read DHCP message type */ 01268 msg_type = dhcp_get_option_byte(options_ptr + 2); 01269 /* message type is DHCP ACK? */ 01270 if (msg_type == DHCP_ACK) { 01271 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("DHCP_ACK received\n")); 01272 /* in requesting state? */ 01273 if (dhcp->state == DHCP_REQUESTING) { 01274 dhcp_handle_ack(netif); 01275 dhcp->request_timeout = 0; 01276 #if DHCP_DOES_ARP_CHECK 01277 /* check if the acknowledged lease address is already in use */ 01278 dhcp_check(netif); 01279 #else 01280 /* bind interface to the acknowledged lease address */ 01281 dhcp_bind(netif); 01282 #endif 01283 } 01284 /* already bound to the given lease address? */ 01285 else if ((dhcp->state == DHCP_REBOOTING) || (dhcp->state == DHCP_REBINDING) || (dhcp->state == DHCP_RENEWING)) { 01286 dhcp->request_timeout = 0; 01287 dhcp_bind(netif); 01288 } 01289 } 01290 /* received a DHCP_NAK in appropriate state? */ 01291 else if ((msg_type == DHCP_NAK) && 01292 ((dhcp->state == DHCP_REBOOTING) || (dhcp->state == DHCP_REQUESTING) || 01293 (dhcp->state == DHCP_REBINDING) || (dhcp->state == DHCP_RENEWING ))) { 01294 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("DHCP_NAK received\n")); 01295 dhcp->request_timeout = 0; 01296 dhcp_handle_nak(netif); 01297 } 01298 /* received a DHCP_OFFER in DHCP_SELECTING state? */ 01299 else if ((msg_type == DHCP_OFFER) && (dhcp->state == DHCP_SELECTING)) { 01300 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("DHCP_OFFER received in DHCP_SELECTING state\n")); 01301 dhcp->request_timeout = 0; 01302 /* remember offered lease */ 01303 dhcp_handle_offer(netif); 01304 } 01305 free_pbuf_and_return: 01306 pbuf_free(p); 01307 dhcp->p = NULL; 01308 } 01309 01310 /** 01311 * Create a DHCP request, fill in common headers 01312 * 01313 * @param netif the netif under DHCP control 01314 */ 01315 static err_t 01316 dhcp_create_request(struct netif *netif) 01317 { 01318 struct dhcp *dhcp; 01319 u16_t i; 01320 LWIP_ERROR("dhcp_create_request: netif != NULL", (netif != NULL), return ERR_ARG;); 01321 dhcp = netif->dhcp; 01322 LWIP_ERROR("dhcp_create_request: dhcp != NULL", (dhcp != NULL), return ERR_VAL;); 01323 LWIP_ASSERT("dhcp_create_request: dhcp->p_out == NULL", dhcp->p_out == NULL); 01324 LWIP_ASSERT("dhcp_create_request: dhcp->msg_out == NULL", dhcp->msg_out == NULL); 01325 dhcp->p_out = pbuf_alloc(PBUF_TRANSPORT, sizeof(struct dhcp_msg), PBUF_RAM); 01326 if (dhcp->p_out == NULL) { 01327 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("dhcp_create_request(): could not allocate pbuf\n")); 01328 return ERR_MEM; 01329 } 01330 LWIP_ASSERT("dhcp_create_request: check that first pbuf can hold struct dhcp_msg", 01331 (dhcp->p_out->len >= sizeof(struct dhcp_msg))); 01332 01333 /* give unique transaction identifier to this request */ 01334 dhcp->xid = xid++; 01335 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("transaction id xid++(%"X32_F") dhcp->xid(%"U32_F")\n",xid,dhcp->xid)); 01336 01337 dhcp->msg_out = (struct dhcp_msg *)dhcp->p_out->payload; 01338 01339 dhcp->msg_out->op = DHCP_BOOTREQUEST; 01340 /* TODO: make link layer independent */ 01341 dhcp->msg_out->htype = DHCP_HTYPE_ETH; 01342 /* TODO: make link layer independent */ 01343 dhcp->msg_out->hlen = DHCP_HLEN_ETH; 01344 dhcp->msg_out->hops = 0; 01345 dhcp->msg_out->xid = htonl(dhcp->xid); 01346 dhcp->msg_out->secs = 0; 01347 dhcp->msg_out->flags = 0; 01348 dhcp->msg_out->ciaddr.addr = netif->ip_addr.addr; 01349 dhcp->msg_out->yiaddr.addr = 0; 01350 dhcp->msg_out->siaddr.addr = 0; 01351 dhcp->msg_out->giaddr.addr = 0; 01352 for (i = 0; i < DHCP_CHADDR_LEN; i++) { 01353 /* copy netif hardware address, pad with zeroes */ 01354 dhcp->msg_out->chaddr[i] = (i < netif->hwaddr_len) ? netif->hwaddr[i] : 0/* pad byte*/; 01355 } 01356 for (i = 0; i < DHCP_SNAME_LEN; i++) { 01357 dhcp->msg_out->sname[i] = 0; 01358 } 01359 for (i = 0; i < DHCP_FILE_LEN; i++) { 01360 dhcp->msg_out->file[i] = 0; 01361 } 01362 dhcp->msg_out->cookie = htonl(0x63825363UL); 01363 dhcp->options_out_len = 0; 01364 /* fill options field with an incrementing array (for debugging purposes) */ 01365 for (i = 0; i < DHCP_OPTIONS_LEN; i++) { 01366 dhcp->msg_out->options[i] = (u8_t)i; /* for debugging only, no matter if truncated */ 01367 } 01368 return ERR_OK; 01369 } 01370 01371 /** 01372 * Free previously allocated memory used to send a DHCP request. 01373 * 01374 * @param netif the netif under DHCP control 01375 */ 01376 static void 01377 dhcp_delete_request(struct netif *netif) 01378 { 01379 struct dhcp *dhcp; 01380 LWIP_ERROR("dhcp_delete_request: netif != NULL", (netif != NULL), return;); 01381 dhcp = netif->dhcp; 01382 LWIP_ERROR("dhcp_delete_request: dhcp != NULL", (dhcp != NULL), return;); 01383 LWIP_ASSERT("dhcp_delete_request: dhcp->p_out != NULL", dhcp->p_out != NULL); 01384 LWIP_ASSERT("dhcp_delete_request: dhcp->msg_out != NULL", dhcp->msg_out != NULL); 01385 if (dhcp->p_out != NULL) { 01386 pbuf_free(dhcp->p_out); 01387 } 01388 dhcp->p_out = NULL; 01389 dhcp->msg_out = NULL; 01390 } 01391 01392 /** 01393 * Add a DHCP message trailer 01394 * 01395 * Adds the END option to the DHCP message, and if 01396 * necessary, up to three padding bytes. 01397 * 01398 * @param dhcp DHCP state structure 01399 */ 01400 static void 01401 dhcp_option_trailer(struct dhcp *dhcp) 01402 { 01403 LWIP_ERROR("dhcp_option_trailer: dhcp != NULL", (dhcp != NULL), return;); 01404 LWIP_ASSERT("dhcp_option_trailer: dhcp->msg_out != NULL\n", dhcp->msg_out != NULL); 01405 LWIP_ASSERT("dhcp_option_trailer: dhcp->options_out_len < DHCP_OPTIONS_LEN\n", dhcp->options_out_len < DHCP_OPTIONS_LEN); 01406 dhcp->msg_out->options[dhcp->options_out_len++] = DHCP_OPTION_END; 01407 /* packet is too small, or not 4 byte aligned? */ 01408 while ((dhcp->options_out_len < DHCP_MIN_OPTIONS_LEN) || (dhcp->options_out_len & 3)) { 01409 /* LWIP_DEBUGF(DHCP_DEBUG,("dhcp_option_trailer:dhcp->options_out_len=%"U16_F", DHCP_OPTIONS_LEN=%"U16_F, dhcp->options_out_len, DHCP_OPTIONS_LEN)); */ 01410 LWIP_ASSERT("dhcp_option_trailer: dhcp->options_out_len < DHCP_OPTIONS_LEN\n", dhcp->options_out_len < DHCP_OPTIONS_LEN); 01411 /* add a fill/padding byte */ 01412 dhcp->msg_out->options[dhcp->options_out_len++] = 0; 01413 } 01414 } 01415 01416 /** 01417 * Find the offset of a DHCP option inside the DHCP message. 01418 * 01419 * @param dhcp DHCP client 01420 * @param option_type 01421 * 01422 * @return a byte offset into the UDP message where the option was found, or 01423 * zero if the given option was not found. 01424 */ 01425 static u8_t *dhcp_get_option_ptr(struct dhcp *dhcp, u8_t option_type) 01426 { 01427 u8_t overload = DHCP_OVERLOAD_NONE; 01428 01429 /* options available? */ 01430 if ((dhcp->options_in != NULL) && (dhcp->options_in_len > 0)) { 01431 /* start with options field */ 01432 u8_t *options = (u8_t *)dhcp->options_in; 01433 u16_t offset = 0; 01434 /* at least 1 byte to read and no end marker, then at least 3 bytes to read? */ 01435 while ((offset < dhcp->options_in_len) && (options[offset] != DHCP_OPTION_END)) { 01436 /* LWIP_DEBUGF(DHCP_DEBUG, ("msg_offset=%"U16_F", q->len=%"U16_F, msg_offset, q->len)); */ 01437 /* are the sname and/or file field overloaded with options? */ 01438 if (options[offset] == DHCP_OPTION_OVERLOAD) { 01439 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 2, ("overloaded message detected\n")); 01440 /* skip option type and length */ 01441 offset += 2; 01442 overload = options[offset++]; 01443 } 01444 /* requested option found */ 01445 else if (options[offset] == option_type) { 01446 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("option found at offset %"U16_F" in options\n", offset)); 01447 return &options[offset]; 01448 /* skip option */ 01449 } else { 01450 LWIP_DEBUGF(DHCP_DEBUG, ("skipping option %"U16_F" in options\n", options[offset])); 01451 /* skip option type */ 01452 offset++; 01453 /* skip option length, and then length bytes */ 01454 offset += 1 + options[offset]; 01455 } 01456 } 01457 /* is this an overloaded message? */ 01458 if (overload != DHCP_OVERLOAD_NONE) { 01459 u16_t field_len; 01460 if (overload == DHCP_OVERLOAD_FILE) { 01461 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("overloaded file field\n")); 01462 options = (u8_t *)&dhcp->msg_in->file; 01463 field_len = DHCP_FILE_LEN; 01464 } else if (overload == DHCP_OVERLOAD_SNAME) { 01465 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("overloaded sname field\n")); 01466 options = (u8_t *)&dhcp->msg_in->sname; 01467 field_len = DHCP_SNAME_LEN; 01468 /* TODO: check if else if () is necessary */ 01469 } else { 01470 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE | 1, ("overloaded sname and file field\n")); 01471 options = (u8_t *)&dhcp->msg_in->sname; 01472 field_len = DHCP_FILE_LEN + DHCP_SNAME_LEN; 01473 } 01474 offset = 0; 01475 01476 /* at least 1 byte to read and no end marker */ 01477 while ((offset < field_len) && (options[offset] != DHCP_OPTION_END)) { 01478 if (options[offset] == option_type) { 01479 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("option found at offset=%"U16_F"\n", offset)); 01480 return &options[offset]; 01481 /* skip option */ 01482 } else { 01483 LWIP_DEBUGF(DHCP_DEBUG | LWIP_DBG_TRACE, ("skipping option %"U16_F"\n", options[offset])); 01484 /* skip option type */ 01485 offset++; 01486 offset += 1 + options[offset]; 01487 } 01488 } 01489 } 01490 } 01491 return NULL; 01492 } 01493 01494 /** 01495 * Return the byte of DHCP option data. 01496 * 01497 * @param client DHCP client. 01498 * @param ptr pointer obtained by dhcp_get_option_ptr(). 01499 * 01500 * @return byte value at the given address. 01501 */ 01502 static u8_t 01503 dhcp_get_option_byte(u8_t *ptr) 01504 { 01505 LWIP_DEBUGF(DHCP_DEBUG, ("option byte value=%"U16_F"\n", (u16_t)(*ptr))); 01506 return *ptr; 01507 } 01508 01509 #if 0 /* currently unused */ 01510 /** 01511 * Return the 16-bit value of DHCP option data. 01512 * 01513 * @param client DHCP client. 01514 * @param ptr pointer obtained by dhcp_get_option_ptr(). 01515 * 01516 * @return byte value at the given address. 01517 */ 01518 static u16_t 01519 dhcp_get_option_short(u8_t *ptr) 01520 { 01521 u16_t value; 01522 value = *ptr++ << 8; 01523 value |= *ptr; 01524 LWIP_DEBUGF(DHCP_DEBUG, ("option short value=%"U16_F"\n", value)); 01525 return value; 01526 } 01527 #endif 01528 01529 /** 01530 * Return the 32-bit value of DHCP option data. 01531 * 01532 * @param client DHCP client. 01533 * @param ptr pointer obtained by dhcp_get_option_ptr(). 01534 * 01535 * @return byte value at the given address. 01536 */ 01537 static u32_t dhcp_get_option_long(u8_t *ptr) 01538 { 01539 u32_t value; 01540 value = (u32_t)(*ptr++) << 24; 01541 value |= (u32_t)(*ptr++) << 16; 01542 value |= (u32_t)(*ptr++) << 8; 01543 value |= (u32_t)(*ptr++); 01544 LWIP_DEBUGF(DHCP_DEBUG, ("option long value=%"U32_F"\n", value)); 01545 return value; 01546 } 01547 01548 #endif /* LWIP_DHCP */
Generated on Tue Jul 12 2022 16:06:03 by
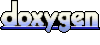