A version of LWIP, provided for backwards compatibility.
Dependents: AA_DemoBoard DemoBoard HelloServerDemo DemoBoard_RangeIndicator ... more
autoip.c
00001 /** 00002 * @file 00003 * AutoIP Automatic LinkLocal IP Configuration 00004 * 00005 */ 00006 00007 /* 00008 * 00009 * Copyright (c) 2007 Dominik Spies <kontakt@dspies.de> 00010 * All rights reserved. 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. The name of the author may not be used to endorse or promote products 00021 * derived from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00024 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00025 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00026 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00027 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00028 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00029 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00030 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00031 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00032 * OF SUCH DAMAGE. 00033 * 00034 * Author: Dominik Spies <kontakt@dspies.de> 00035 * 00036 * This is a AutoIP implementation for the lwIP TCP/IP stack. It aims to conform 00037 * with RFC 3927. 00038 * 00039 * 00040 * Please coordinate changes and requests with Dominik Spies 00041 * <kontakt@dspies.de> 00042 */ 00043 00044 /******************************************************************************* 00045 * USAGE: 00046 * 00047 * define LWIP_AUTOIP 1 in your lwipopts.h 00048 * 00049 * If you don't use tcpip.c (so, don't call, you don't call tcpip_init): 00050 * - First, call autoip_init(). 00051 * - call autoip_tmr() all AUTOIP_TMR_INTERVAL msces, 00052 * that should be defined in autoip.h. 00053 * I recommend a value of 100. The value must divide 1000 with a remainder almost 0. 00054 * Possible values are 1000, 500, 333, 250, 200, 166, 142, 125, 111, 100 .... 00055 * 00056 * Without DHCP: 00057 * - Call autoip_start() after netif_add(). 00058 * 00059 * With DHCP: 00060 * - define LWIP_DHCP_AUTOIP_COOP 1 in your lwipopts.h. 00061 * - Configure your DHCP Client. 00062 * 00063 */ 00064 00065 #include "lwip/opt.h" 00066 00067 #if LWIP_AUTOIP /* don't build if not configured for use in lwipopts.h */ 00068 00069 #include "lwip/mem.h" 00070 #include "lwip/udp.h" 00071 #include "lwip/ip_addr.h" 00072 #include "lwip/netif.h" 00073 #include "lwip/autoip.h" 00074 #include "netif/etharp.h" 00075 00076 #include <stdlib.h> 00077 #include <string.h> 00078 00079 /* Pseudo random macro based on netif informations. 00080 * You could use "rand()" from the C Library if you define LWIP_AUTOIP_RAND in lwipopts.h */ 00081 #ifndef LWIP_AUTOIP_RAND 00082 #define LWIP_AUTOIP_RAND(netif) ( (((u32_t)((netif->hwaddr[5]) & 0xff) << 24) | \ 00083 ((u32_t)((netif->hwaddr[3]) & 0xff) << 16) | \ 00084 ((u32_t)((netif->hwaddr[2]) & 0xff) << 8) | \ 00085 ((u32_t)((netif->hwaddr[4]) & 0xff))) + \ 00086 (netif->autoip?netif->autoip->tried_llipaddr:0)) 00087 #endif /* LWIP_AUTOIP_RAND */ 00088 00089 /* static functions */ 00090 static void autoip_handle_arp_conflict(struct netif *netif); 00091 00092 /* creates random LL IP-Address for a network interface */ 00093 static void autoip_create_rand_addr(struct netif *netif, struct ip_addr *RandomIPAddr); 00094 00095 /* sends an ARP announce */ 00096 static err_t autoip_arp_announce(struct netif *netif); 00097 00098 /* configure interface for use with current LL IP-Address */ 00099 static err_t autoip_bind(struct netif *netif); 00100 00101 /** 00102 * Initialize this module 00103 */ 00104 void 00105 autoip_init(void) 00106 { 00107 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | 3, ("autoip_init()\n")); 00108 } 00109 00110 /** 00111 * Handle a IP address conflict after an ARP conflict detection 00112 */ 00113 static void 00114 autoip_handle_arp_conflict(struct netif *netif) 00115 { 00116 /* Somehow detect if we are defending or retreating */ 00117 unsigned char defend = 1; /* tbd */ 00118 00119 if(defend) { 00120 if(netif->autoip->lastconflict > 0) { 00121 /* retreat, there was a conflicting ARP in the last 00122 * DEFEND_INTERVAL seconds 00123 */ 00124 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 1, 00125 ("autoip_handle_arp_conflict(): we are defending, but in DEFEND_INTERVAL, retreating\n")); 00126 00127 /* TODO: close all TCP sessions */ 00128 autoip_start(netif); 00129 } else { 00130 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 1, 00131 ("autoip_handle_arp_conflict(): we are defend, send ARP Announce\n")); 00132 autoip_arp_announce(netif); 00133 netif->autoip->lastconflict = DEFEND_INTERVAL * AUTOIP_TICKS_PER_SECOND; 00134 } 00135 } else { 00136 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 1, 00137 ("autoip_handle_arp_conflict(): we do not defend, retreating\n")); 00138 /* TODO: close all TCP sessions */ 00139 autoip_start(netif); 00140 } 00141 } 00142 00143 /** 00144 * Create an IP-Address out of range 169.254.1.0 to 169.254.254.255 00145 * 00146 * @param netif network interface on which create the IP-Address 00147 * @param RandomIPAddr ip address to initialize 00148 */ 00149 static void 00150 autoip_create_rand_addr(struct netif *netif, struct ip_addr *RandomIPAddr) 00151 { 00152 /* Here we create an IP-Address out of range 169.254.1.0 to 169.254.254.255 00153 * compliant to RFC 3927 Section 2.1 00154 * We have 254 * 256 possibilities 00155 */ 00156 00157 RandomIPAddr->addr = (0xA9FE0100 + ((u32_t)(((u8_t)(netif->hwaddr[4])) | 00158 ((u32_t)((u8_t)(netif->hwaddr[5]))) << 8)) + netif->autoip->tried_llipaddr); 00159 00160 if (RandomIPAddr->addr>0xA9FEFEFF) { 00161 RandomIPAddr->addr = (0xA9FE0100 + (RandomIPAddr->addr-0xA9FEFEFF)); 00162 } 00163 if (RandomIPAddr->addr<0xA9FE0100) { 00164 RandomIPAddr->addr = (0xA9FEFEFF - (0xA9FE0100-RandomIPAddr->addr)); 00165 } 00166 RandomIPAddr->addr = htonl(RandomIPAddr->addr); 00167 00168 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 1, 00169 ("autoip_create_rand_addr(): tried_llipaddr=%"U16_F", 0x%08"X32_F"\n", 00170 (u16_t)(netif->autoip->tried_llipaddr), (u32_t)(RandomIPAddr->addr))); 00171 } 00172 00173 /** 00174 * Sends an ARP announce from a network interface 00175 * 00176 * @param netif network interface used to send the announce 00177 */ 00178 static err_t 00179 autoip_arp_announce(struct netif *netif) 00180 { 00181 return etharp_raw(netif, (struct eth_addr *)netif->hwaddr, ðbroadcast, 00182 (struct eth_addr *)netif->hwaddr, &netif->autoip->llipaddr, ðzero, 00183 &netif->autoip->llipaddr, ARP_REQUEST); 00184 } 00185 00186 /** 00187 * Configure interface for use with current LL IP-Address 00188 * 00189 * @param netif network interface to configure with current LL IP-Address 00190 */ 00191 static err_t 00192 autoip_bind(struct netif *netif) 00193 { 00194 struct autoip *autoip = netif->autoip; 00195 struct ip_addr sn_mask, gw_addr; 00196 00197 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | 3, 00198 ("autoip_bind(netif=%p) %c%c%"U16_F" 0x%08"X32_F"\n", 00199 (void*)netif, netif->name[0], netif->name[1], (u16_t)netif->num, autoip->llipaddr.addr)); 00200 00201 IP4_ADDR(&sn_mask, 255, 255, 0, 0); 00202 IP4_ADDR(&gw_addr, 0, 0, 0, 0); 00203 00204 netif_set_ipaddr(netif, &autoip->llipaddr); 00205 netif_set_netmask(netif, &sn_mask); 00206 netif_set_gw(netif, &gw_addr); 00207 00208 /* bring the interface up */ 00209 netif_set_up(netif); 00210 00211 return ERR_OK; 00212 } 00213 00214 /** 00215 * Start AutoIP client 00216 * 00217 * @param netif network interface on which start the AutoIP client 00218 */ 00219 err_t 00220 autoip_start(struct netif *netif) 00221 { 00222 struct autoip *autoip = netif->autoip; 00223 err_t result = ERR_OK; 00224 00225 if(netif_is_up(netif)) { 00226 netif_set_down(netif); 00227 } 00228 00229 /* Set IP-Address, Netmask and Gateway to 0 to make sure that 00230 * ARP Packets are formed correctly 00231 */ 00232 netif->ip_addr.addr = 0; 00233 netif->netmask.addr = 0; 00234 netif->gw.addr = 0; 00235 00236 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE, 00237 ("autoip_start(netif=%p) %c%c%"U16_F"\n", (void*)netif, netif->name[0], 00238 netif->name[1], (u16_t)netif->num)); 00239 if(autoip == NULL) { 00240 /* no AutoIP client attached yet? */ 00241 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE, 00242 ("autoip_start(): starting new AUTOIP client\n")); 00243 autoip = (struct autoip *)mem_malloc(sizeof(struct autoip)); // XXX: static_cast<struct autoip *>(x) 00244 if(autoip == NULL) { 00245 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE, 00246 ("autoip_start(): could not allocate autoip\n")); 00247 return ERR_MEM; 00248 } 00249 memset( autoip, 0, sizeof(struct autoip)); 00250 /* store this AutoIP client in the netif */ 00251 netif->autoip = autoip; 00252 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE, ("autoip_start(): allocated autoip")); 00253 } else { 00254 autoip->state = AUTOIP_STATE_OFF; 00255 autoip->ttw = 0; 00256 autoip->sent_num = 0; 00257 memset(&autoip->llipaddr, 0, sizeof(struct ip_addr)); 00258 autoip->lastconflict = 0; 00259 } 00260 00261 autoip_create_rand_addr(netif, &(autoip->llipaddr)); 00262 autoip->tried_llipaddr++; 00263 autoip->state = AUTOIP_STATE_PROBING; 00264 autoip->sent_num = 0; 00265 00266 /* time to wait to first probe, this is randomly 00267 * choosen out of 0 to PROBE_WAIT seconds. 00268 * compliant to RFC 3927 Section 2.2.1 00269 */ 00270 autoip->ttw = (u16_t)(LWIP_AUTOIP_RAND(netif) % (PROBE_WAIT * AUTOIP_TICKS_PER_SECOND)); 00271 00272 /* 00273 * if we tried more then MAX_CONFLICTS we must limit our rate for 00274 * accquiring and probing address 00275 * compliant to RFC 3927 Section 2.2.1 00276 */ 00277 00278 if(autoip->tried_llipaddr > MAX_CONFLICTS) { 00279 autoip->ttw = RATE_LIMIT_INTERVAL * AUTOIP_TICKS_PER_SECOND; 00280 } 00281 00282 return result; 00283 } 00284 00285 /** 00286 * Stop AutoIP client 00287 * 00288 * @param netif network interface on which stop the AutoIP client 00289 */ 00290 err_t 00291 autoip_stop(struct netif *netif) 00292 { 00293 netif->autoip->state = AUTOIP_STATE_OFF; 00294 netif_set_down(netif); 00295 return ERR_OK; 00296 } 00297 00298 /** 00299 * Has to be called in loop every AUTOIP_TMR_INTERVAL milliseconds 00300 */ 00301 void 00302 autoip_tmr() 00303 { 00304 struct netif *netif = netif_list; 00305 /* loop through netif's */ 00306 while (netif != NULL) { 00307 /* only act on AutoIP configured interfaces */ 00308 if (netif->autoip != NULL) { 00309 if(netif->autoip->lastconflict > 0) { 00310 netif->autoip->lastconflict--; 00311 } 00312 00313 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE, 00314 ("autoip_tmr() AutoIP-State: %"U16_F", ttw=%"U16_F"\n", 00315 (u16_t)(netif->autoip->state), netif->autoip->ttw)); 00316 00317 switch(netif->autoip->state) { 00318 case AUTOIP_STATE_PROBING: 00319 if(netif->autoip->ttw > 0) { 00320 netif->autoip->ttw--; 00321 } else { 00322 if(netif->autoip->sent_num == PROBE_NUM) { 00323 netif->autoip->state = AUTOIP_STATE_ANNOUNCING; 00324 netif->autoip->sent_num = 0; 00325 netif->autoip->ttw = ANNOUNCE_WAIT * AUTOIP_TICKS_PER_SECOND; 00326 } else { 00327 etharp_request(netif, &(netif->autoip->llipaddr)); 00328 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | 3, 00329 ("autoip_tmr() PROBING Sent Probe\n")); 00330 netif->autoip->sent_num++; 00331 /* calculate time to wait to next probe */ 00332 netif->autoip->ttw = (u16_t)((LWIP_AUTOIP_RAND(netif) % 00333 ((PROBE_MAX - PROBE_MIN) * AUTOIP_TICKS_PER_SECOND) ) + 00334 PROBE_MIN * AUTOIP_TICKS_PER_SECOND); 00335 } 00336 } 00337 break; 00338 00339 case AUTOIP_STATE_ANNOUNCING: 00340 if(netif->autoip->ttw > 0) { 00341 netif->autoip->ttw--; 00342 } else { 00343 if(netif->autoip->sent_num == 0) { 00344 /* We are here the first time, so we waited ANNOUNCE_WAIT seconds 00345 * Now we can bind to an IP address and use it 00346 */ 00347 autoip_bind(netif); 00348 } 00349 00350 if(netif->autoip->sent_num == ANNOUNCE_NUM) { 00351 netif->autoip->state = AUTOIP_STATE_BOUND; 00352 netif->autoip->sent_num = 0; 00353 netif->autoip->ttw = 0; 00354 } else { 00355 autoip_arp_announce(netif); 00356 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | 3, 00357 ("autoip_tmr() ANNOUNCING Sent Announce\n")); 00358 netif->autoip->sent_num++; 00359 netif->autoip->ttw = ANNOUNCE_INTERVAL * AUTOIP_TICKS_PER_SECOND; 00360 } 00361 } 00362 break; 00363 } 00364 } 00365 /* proceed to next network interface */ 00366 netif = netif->next; 00367 } 00368 } 00369 00370 /** 00371 * Handles every incoming ARP Packet, called by etharp_arp_input. 00372 * 00373 * @param netif network interface to use for autoip processing 00374 * @param hdr Incoming ARP packet 00375 */ 00376 void 00377 autoip_arp_reply(struct netif *netif, struct etharp_hdr *hdr) 00378 { 00379 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | 3, ("autoip_arp_reply()\n")); 00380 if ((netif->autoip != NULL) && (netif->autoip->state != AUTOIP_STATE_OFF)) { 00381 /* when ip.src == llipaddr && hw.src != netif->hwaddr 00382 * 00383 * when probing ip.dst == llipaddr && hw.src != netif->hwaddr 00384 * we have a conflict and must solve it 00385 */ 00386 struct ip_addr sipaddr, dipaddr; 00387 struct eth_addr netifaddr; 00388 netifaddr.addr[0] = netif->hwaddr[0]; 00389 netifaddr.addr[1] = netif->hwaddr[1]; 00390 netifaddr.addr[2] = netif->hwaddr[2]; 00391 netifaddr.addr[3] = netif->hwaddr[3]; 00392 netifaddr.addr[4] = netif->hwaddr[4]; 00393 netifaddr.addr[5] = netif->hwaddr[5]; 00394 00395 /* Copy struct ip_addr2 to aligned ip_addr, to support compilers without 00396 * structure packing (not using structure copy which breaks strict-aliasing rules). 00397 */ 00398 MEMCPY(&sipaddr, &hdr->sipaddr, sizeof(sipaddr)); 00399 MEMCPY(&dipaddr, &hdr->dipaddr, sizeof(dipaddr)); 00400 00401 if ((netif->autoip->state == AUTOIP_STATE_PROBING) || 00402 ((netif->autoip->state == AUTOIP_STATE_ANNOUNCING) && 00403 (netif->autoip->sent_num == 0))) { 00404 /* RFC 3927 Section 2.2.1: 00405 * from beginning to after ANNOUNCE_WAIT 00406 * seconds we have a conflict if 00407 * ip.src == llipaddr OR 00408 * ip.dst == llipaddr && hw.src != own hwaddr 00409 */ 00410 if ((ip_addr_cmp(&sipaddr, &netif->autoip->llipaddr)) || 00411 (ip_addr_cmp(&dipaddr, &netif->autoip->llipaddr) && 00412 !eth_addr_cmp(&netifaddr, &hdr->shwaddr))) { 00413 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 1, 00414 ("autoip_arp_reply(): Probe Conflict detected\n")); 00415 autoip_start(netif); 00416 } 00417 } else { 00418 /* RFC 3927 Section 2.5: 00419 * in any state we have a conflict if 00420 * ip.src == llipaddr && hw.src != own hwaddr 00421 */ 00422 if (ip_addr_cmp(&sipaddr, &netif->autoip->llipaddr) && 00423 !eth_addr_cmp(&netifaddr, &hdr->shwaddr)) { 00424 LWIP_DEBUGF(AUTOIP_DEBUG | LWIP_DBG_TRACE | LWIP_DBG_STATE | 1, 00425 ("autoip_arp_reply(): Conflicting ARP-Packet detected\n")); 00426 autoip_handle_arp_conflict(netif); 00427 } 00428 } 00429 } 00430 } 00431 00432 #endif /* LWIP_AUTOIP */
Generated on Tue Jul 12 2022 16:06:00 by
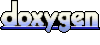