A version of LWIP, provided for backwards compatibility.
Dependents: AA_DemoBoard DemoBoard HelloServerDemo DemoBoard_RangeIndicator ... more
main.cpp
00001 #include "lwip/opt.h" 00002 #include "lwip/stats.h" 00003 #include "lwip/sys.h" 00004 #include "lwip/pbuf.h" 00005 #include "lwip/udp.h" 00006 #include "lwip/tcp.h" 00007 #include "lwip/dns.h" 00008 #include "lwip/dhcp.h" 00009 #include "lwip/init.h" 00010 #include "lwip/netif.h" 00011 #include "netif/etharp.h" 00012 #include "netif/loopif.h" 00013 #include "device.h" 00014 00015 #include "mbed.h" 00016 00017 DigitalOut led(LED1); 00018 00019 /* Struct with hold the Ethernet Data */ 00020 struct netif netif_data; 00021 00022 /* Every time called if a packet is received for a */ 00023 /* TCPConnection which registerd this Callback. */ 00024 err_t recv_callback(void *arg, struct tcp_pcb *pcb, struct pbuf *p, err_t err) { 00025 printf("recv_callback\n"); 00026 if (p==NULL) { 00027 printf("Connection closed by server!\n"); 00028 return ERR_OK; 00029 } 00030 00031 while (p) { 00032 char * ch = (char*)p->payload; 00033 printf("\n>>>>>>>>>>>>\n"); 00034 for (int i=0; i < p->len; i++) { 00035 printf("%c", ch[i]); 00036 } 00037 printf("\n<<<<<<<<<<<<\n"); 00038 p = p->next; 00039 } 00040 tcp_recved(pcb, p->tot_len); 00041 pbuf_free(p); 00042 return ERR_OK; 00043 } 00044 00045 /* Connection etablished, lets try to get a http page */ 00046 err_t connected_callback(void *arg, struct tcp_pcb *pcb, err_t err) { 00047 tcp_recv(pcb, &recv_callback); 00048 printf("Connected Callback!\n"); 00049 char msg[] = "GET / HTTP/1.1\r\nHost: www.google.co.uk\r\n\r\n"; 00050 if (tcp_write(pcb, msg, strlen(msg), 1) != ERR_OK) { 00051 error("Could not write", 0); 00052 } 00053 return ERR_OK; 00054 } 00055 00056 int main(void) { 00057 /* Create and initialise variables */ 00058 struct netif *netif = &netif_data; 00059 struct ip_addr ipaddr; 00060 struct ip_addr target; 00061 struct ip_addr netmask; 00062 struct ip_addr gateway; 00063 00064 Ticker tickFast, tickSlow, tickARP, eth_tick, dns_tick, dhcp_coarse, dhcp_fine; 00065 char *hostname = "mbed-c3p0"; 00066 00067 /* Start Network with DHCP */ 00068 IP4_ADDR(&netmask, 255,255,255,255); 00069 IP4_ADDR(&gateway, 0,0,0,0); 00070 IP4_ADDR(&ipaddr, 0,0,0,0); 00071 IP4_ADDR(&target, 209,85,229,147); // www.google.co.uk 00072 00073 /* Initialise after configuration */ 00074 lwip_init(); 00075 00076 netif->hwaddr_len = ETHARP_HWADDR_LEN; 00077 device_address((char *)netif->hwaddr); 00078 00079 netif = netif_add(netif, &ipaddr, &netmask, &gateway, NULL, device_init, ip_input); 00080 netif->hostname = hostname; 00081 netif_set_default(netif); 00082 dhcp_start(netif); // <-- Use DHCP 00083 00084 /* Initialise all needed timers */ 00085 tickARP.attach_us( ðarp_tmr, ARP_TMR_INTERVAL * 1000); 00086 tickFast.attach_us(&tcp_fasttmr, TCP_FAST_INTERVAL * 1000); 00087 tickSlow.attach_us(&tcp_slowtmr, TCP_SLOW_INTERVAL * 1000); 00088 dns_tick.attach_us(&dns_tmr, DNS_TMR_INTERVAL * 1000); 00089 dhcp_coarse.attach_us(&dhcp_coarse_tmr, DHCP_COARSE_TIMER_MSECS * 1000); 00090 dhcp_fine.attach_us(&dhcp_fine_tmr, DHCP_FINE_TIMER_MSECS * 1000); 00091 00092 // Wait for an IP Address 00093 while (!netif_is_up(netif)) { 00094 device_poll(); 00095 } 00096 00097 /* Connect do google */ 00098 struct tcp_pcb *pcb = tcp_new(); 00099 tcp_connect(pcb, &target, 80, &connected_callback); 00100 00101 /* Give the signal that we are ready */ 00102 printf("entering while loop\n"); 00103 while (1) { 00104 led = !led; 00105 wait(0.1); 00106 device_poll(); 00107 } 00108 } 00109
Generated on Tue Jul 12 2022 16:06:15 by
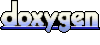