mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
mbed_retarget.h
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); 00007 * you may not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, 00014 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 * 00018 */ 00019 00020 #ifndef RETARGET_H 00021 #define RETARGET_H 00022 00023 #if __cplusplus 00024 #include <cstdio> 00025 #else 00026 #include <stdio.h> 00027 #endif //__cplusplus 00028 #include <stdint.h> 00029 #include <stddef.h> 00030 00031 /* Include logic for errno so we can get errno defined but not bring in error_t, 00032 * including errno here prevents an include later, which would redefine our 00033 * error codes 00034 */ 00035 #ifndef __error_t_defined 00036 #define __error_t_defined 1 00037 #include <errno.h> 00038 #undef __error_t_defined 00039 #else 00040 #include <errno.h> 00041 #endif 00042 00043 /* We can get the following standard types from sys/types for gcc, but we 00044 * need to define the types ourselves for the other compilers that normally 00045 * target embedded systems */ 00046 typedef signed int ssize_t; ///< Signed size type, usually encodes negative errors 00047 typedef signed long off_t; ///< Offset in a data stream 00048 typedef unsigned int nfds_t; ///< Number of file descriptors 00049 typedef unsigned long long fsblkcnt_t; ///< Count of file system blocks 00050 #if defined(__ARMCC_VERSION) || !defined(__GNUC__) 00051 typedef unsigned int mode_t; ///< Mode for opening files 00052 typedef unsigned int dev_t; ///< Device ID type 00053 typedef unsigned long ino_t; ///< File serial number 00054 typedef unsigned int nlink_t; ///< Number of links to a file 00055 typedef unsigned int uid_t; ///< User ID 00056 typedef unsigned int gid_t; ///< Group ID 00057 #endif 00058 00059 /* Flags for open() and fcntl(GETFL/SETFL) 00060 * At present, fcntl only supports reading and writing O_NONBLOCK 00061 */ 00062 #define O_RDONLY 0 ///< Open for reading 00063 #define O_WRONLY 1 ///< Open for writing 00064 #define O_RDWR 2 ///< Open for reading and writing 00065 #define O_NONBLOCK 0x0004 ///< Non-blocking mode 00066 #define O_APPEND 0x0008 ///< Set file offset to end of file prior to each write 00067 #define O_CREAT 0x0200 ///< Create file if it does not exist 00068 #define O_TRUNC 0x0400 ///< Truncate file to zero length 00069 #define O_EXCL 0x0800 ///< Fail if file exists 00070 #define O_BINARY 0x8000 ///< Open file in binary mode 00071 00072 #define O_ACCMODE (O_RDONLY|O_WRONLY|O_RDWR) 00073 00074 #define NAME_MAX 255 ///< Maximum size of a name in a file path 00075 00076 #define STDIN_FILENO 0 00077 #define STDOUT_FILENO 1 00078 #define STDERR_FILENO 2 00079 00080 #include <time.h> 00081 00082 /** \addtogroup platform */ 00083 /** @{*/ 00084 /** 00085 * \defgroup platform_retarget Retarget functions 00086 * @{ 00087 */ 00088 00089 /* DIR declarations must also be here */ 00090 #if __cplusplus 00091 namespace mbed { 00092 00093 class FileHandle; 00094 class DirHandle; 00095 00096 /** Targets may implement this to change stdin, stdout, stderr. 00097 * 00098 * If the application hasn't provided mbed_override_console, this is called 00099 * to give the target a chance to specify a FileHandle for the console. 00100 * 00101 * If this is not provided or returns NULL, the console will be: 00102 * - UARTSerial if configuration option "platform.stdio-buffered-serial" is 00103 * true and the target has DEVICE_SERIAL; 00104 * - Raw HAL serial via serial_getc and serial_putc if 00105 * "platform.stdio-buffered-serial" is false and the target has DEVICE_SERIAL; 00106 * - stdout/stderr will be a sink and stdin will input a stream of 0s if the 00107 * target does not have DEVICE_SERIAL. 00108 * 00109 * @param fd file descriptor - STDIN_FILENO, STDOUT_FILENO or STDERR_FILENO 00110 * @return pointer to FileHandle to override normal stream otherwise NULL 00111 */ 00112 FileHandle *mbed_target_override_console(int fd); 00113 00114 /** Applications may implement this to change stdin, stdout, stderr. 00115 * 00116 * This hook gives the application a chance to specify a custom FileHandle 00117 * for the console. 00118 * 00119 * If this is not provided or returns NULL, the console will be specified 00120 * by mbed_target_override_console, else will default to serial - see 00121 * mbed_target_override_console for more details. 00122 * 00123 * Example: 00124 * @code 00125 * FileHandle* mbed::mbed_override_console(int) { 00126 * static UARTSerial my_serial(D0, D1); 00127 * return &my_serial; 00128 * } 00129 * @endcode 00130 00131 * @param fd file descriptor - STDIN_FILENO, STDOUT_FILENO or STDERR_FILENO 00132 * @return pointer to FileHandle to override normal stream otherwise NULL 00133 */ 00134 FileHandle *mbed_override_console(int fd); 00135 00136 } 00137 00138 typedef mbed::DirHandle DIR; 00139 #else 00140 typedef struct Dir DIR; 00141 #endif 00142 00143 /* The intent of this section is to unify the errno error values to match 00144 * the POSIX definitions for the GCC_ARM, ARMCC and IAR compilers. This is 00145 * necessary because the ARMCC/IAR errno.h, or sys/stat.h are missing some 00146 * symbol definitions used by the POSIX filesystem API to return errno codes. 00147 * Note also that ARMCC errno.h defines some symbol values differently from 00148 * the GCC_ARM/IAR/standard POSIX definitions. The definitions guard against 00149 * this and future changes by changing the symbol definition as shown below. 00150 */ 00151 #undef EPERM 00152 #define EPERM 1 /* Operation not permitted */ 00153 #undef ENOENT 00154 #define ENOENT 2 /* No such file or directory */ 00155 #undef ESRCH 00156 #define ESRCH 3 /* No such process */ 00157 #undef EINTR 00158 #define EINTR 4 /* Interrupted system call */ 00159 #undef EIO 00160 #define EIO 5 /* I/O error */ 00161 #undef ENXIO 00162 #define ENXIO 6 /* No such device or address */ 00163 #undef E2BIG 00164 #define E2BIG 7 /* Argument list too long */ 00165 #undef ENOEXEC 00166 #define ENOEXEC 8 /* Exec format error */ 00167 #undef EBADF 00168 #define EBADF 9 /* Bad file number */ 00169 #undef ECHILD 00170 #define ECHILD 10 /* No child processes */ 00171 #undef EAGAIN 00172 #define EAGAIN 11 /* Try again */ 00173 #undef ENOMEM 00174 #define ENOMEM 12 /* Out of memory */ 00175 #undef EACCES 00176 #define EACCES 13 /* Permission denied */ 00177 #undef EFAULT 00178 #define EFAULT 14 /* Bad address */ 00179 #undef ENOTBLK 00180 #define ENOTBLK 15 /* Block device required */ 00181 #undef EBUSY 00182 #define EBUSY 16 /* Device or resource busy */ 00183 #undef EEXIST 00184 #define EEXIST 17 /* File exists */ 00185 #undef EXDEV 00186 #define EXDEV 18 /* Cross-device link */ 00187 #undef ENODEV 00188 #define ENODEV 19 /* No such device */ 00189 #undef ENOTDIR 00190 #define ENOTDIR 20 /* Not a directory */ 00191 #undef EISDIR 00192 #define EISDIR 21 /* Is a directory */ 00193 #undef EINVAL 00194 #define EINVAL 22 /* Invalid argument */ 00195 #undef ENFILE 00196 #define ENFILE 23 /* File table overflow */ 00197 #undef EMFILE 00198 #define EMFILE 24 /* Too many open files */ 00199 #undef ENOTTY 00200 #define ENOTTY 25 /* Not a typewriter */ 00201 #undef ETXTBSY 00202 #define ETXTBSY 26 /* Text file busy */ 00203 #undef EFBIG 00204 #define EFBIG 27 /* File too large */ 00205 #undef ENOSPC 00206 #define ENOSPC 28 /* No space left on device */ 00207 #undef ESPIPE 00208 #define ESPIPE 29 /* Illegal seek */ 00209 #undef EROFS 00210 #define EROFS 30 /* Read-only file system */ 00211 #undef EMLINK 00212 #define EMLINK 31 /* Too many links */ 00213 #undef EPIPE 00214 #define EPIPE 32 /* Broken pipe */ 00215 #undef EDOM 00216 #define EDOM 33 /* Math argument out of domain of func */ 00217 #undef ERANGE 00218 #define ERANGE 34 /* Math result not representable */ 00219 #undef EDEADLK 00220 #define EDEADLK 35 /* Resource deadlock would occur */ 00221 #undef ENAMETOOLONG 00222 #define ENAMETOOLONG 36 /* File name too long */ 00223 #undef ENOLCK 00224 #define ENOLCK 37 /* No record locks available */ 00225 #undef ENOSYS 00226 #define ENOSYS 38 /* Function not implemented */ 00227 #undef ENOTEMPTY 00228 #define ENOTEMPTY 39 /* Directory not empty */ 00229 #undef ELOOP 00230 #define ELOOP 40 /* Too many symbolic links encountered */ 00231 #undef EWOULDBLOCK 00232 #define EWOULDBLOCK EAGAIN /* Operation would block */ 00233 #undef ENOMSG 00234 #define ENOMSG 42 /* No message of desired type */ 00235 #undef EIDRM 00236 #define EIDRM 43 /* Identifier removed */ 00237 #undef ECHRNG 00238 #define ECHRNG 44 /* Channel number out of range */ 00239 #undef EL2NSYNC 00240 #define EL2NSYNC 45 /* Level 2 not synchronized */ 00241 #undef EL3HLT 00242 #define EL3HLT 46 /* Level 3 halted */ 00243 #undef EL3RST 00244 #define EL3RST 47 /* Level 3 reset */ 00245 #undef ELNRNG 00246 #define ELNRNG 48 /* Link number out of range */ 00247 #undef EUNATCH 00248 #define EUNATCH 49 /* Protocol driver not attached */ 00249 #undef ENOCSI 00250 #define ENOCSI 50 /* No CSI structure available */ 00251 #undef EL2HLT 00252 #define EL2HLT 51 /* Level 2 halted */ 00253 #undef EBADE 00254 #define EBADE 52 /* Invalid exchange */ 00255 #undef EBADR 00256 #define EBADR 53 /* Invalid request descriptor */ 00257 #undef EXFULL 00258 #define EXFULL 54 /* Exchange full */ 00259 #undef ENOANO 00260 #define ENOANO 55 /* No anode */ 00261 #undef EBADRQC 00262 #define EBADRQC 56 /* Invalid request code */ 00263 #undef EBADSLT 00264 #define EBADSLT 57 /* Invalid slot */ 00265 #undef EDEADLOCK 00266 #define EDEADLOCK EDEADLK /* Resource deadlock would occur */ 00267 #undef EBFONT 00268 #define EBFONT 59 /* Bad font file format */ 00269 #undef ENOSTR 00270 #define ENOSTR 60 /* Device not a stream */ 00271 #undef ENODATA 00272 #define ENODATA 61 /* No data available */ 00273 #undef ETIME 00274 #define ETIME 62 /* Timer expired */ 00275 #undef ENOSR 00276 #define ENOSR 63 /* Out of streams resources */ 00277 #undef ENONET 00278 #define ENONET 64 /* Machine is not on the network */ 00279 #undef ENOPKG 00280 #define ENOPKG 65 /* Package not installed */ 00281 #undef EREMOTE 00282 #define EREMOTE 66 /* Object is remote */ 00283 #undef ENOLINK 00284 #define ENOLINK 67 /* Link has been severed */ 00285 #undef EADV 00286 #define EADV 68 /* Advertise error */ 00287 #undef ESRMNT 00288 #define ESRMNT 69 /* Srmount error */ 00289 #undef ECOMM 00290 #define ECOMM 70 /* Communication error on send */ 00291 #undef EPROTO 00292 #define EPROTO 71 /* Protocol error */ 00293 #undef EMULTIHOP 00294 #define EMULTIHOP 72 /* Multihop attempted */ 00295 #undef EDOTDOT 00296 #define EDOTDOT 73 /* RFS specific error */ 00297 #undef EBADMSG 00298 #define EBADMSG 74 /* Not a data message */ 00299 #undef EOVERFLOW 00300 #define EOVERFLOW 75 /* Value too large for defined data type */ 00301 #undef ENOTUNIQ 00302 #define ENOTUNIQ 76 /* Name not unique on network */ 00303 #undef EBADFD 00304 #define EBADFD 77 /* File descriptor in bad state */ 00305 #undef EREMCHG 00306 #define EREMCHG 78 /* Remote address changed */ 00307 #undef ELIBACC 00308 #define ELIBACC 79 /* Can not access a needed shared library */ 00309 #undef ELIBBAD 00310 #define ELIBBAD 80 /* Accessing a corrupted shared library */ 00311 #undef ELIBSCN 00312 #define ELIBSCN 81 /* .lib section in a.out corrupted */ 00313 #undef ELIBMAX 00314 #define ELIBMAX 82 /* Attempting to link in too many shared libraries */ 00315 #undef ELIBEXEC 00316 #define ELIBEXEC 83 /* Cannot exec a shared library directly */ 00317 #undef EILSEQ 00318 #define EILSEQ 84 /* Illegal byte sequence */ 00319 #undef ERESTART 00320 #define ERESTART 85 /* Interrupted system call should be restarted */ 00321 #undef ESTRPIPE 00322 #define ESTRPIPE 86 /* Streams pipe error */ 00323 #undef EUSERS 00324 #define EUSERS 87 /* Too many users */ 00325 #undef ENOTSOCK 00326 #define ENOTSOCK 88 /* Socket operation on non-socket */ 00327 #undef EDESTADDRREQ 00328 #define EDESTADDRREQ 89 /* Destination address required */ 00329 #undef EMSGSIZE 00330 #define EMSGSIZE 90 /* Message too long */ 00331 #undef EPROTOTYPE 00332 #define EPROTOTYPE 91 /* Protocol wrong type for socket */ 00333 #undef ENOPROTOOPT 00334 #define ENOPROTOOPT 92 /* Protocol not available */ 00335 #undef EPROTONOSUPPORT 00336 #define EPROTONOSUPPORT 93 /* Protocol not supported */ 00337 #undef ESOCKTNOSUPPORT 00338 #define ESOCKTNOSUPPORT 94 /* Socket type not supported */ 00339 #undef EOPNOTSUPP 00340 #define EOPNOTSUPP 95 /* Operation not supported on transport endpoint */ 00341 #undef EPFNOSUPPORT 00342 #define EPFNOSUPPORT 96 /* Protocol family not supported */ 00343 #undef EAFNOSUPPORT 00344 #define EAFNOSUPPORT 97 /* Address family not supported by protocol */ 00345 #undef EADDRINUSE 00346 #define EADDRINUSE 98 /* Address already in use */ 00347 #undef EADDRNOTAVAIL 00348 #define EADDRNOTAVAIL 99 /* Cannot assign requested address */ 00349 #undef ENETDOWN 00350 #define ENETDOWN 100 /* Network is down */ 00351 #undef ENETUNREACH 00352 #define ENETUNREACH 101 /* Network is unreachable */ 00353 #undef ENETRESET 00354 #define ENETRESET 102 /* Network dropped connection because of reset */ 00355 #undef ECONNABORTED 00356 #define ECONNABORTED 103 /* Software caused connection abort */ 00357 #undef ECONNRESET 00358 #define ECONNRESET 104 /* Connection reset by peer */ 00359 #undef ENOBUFS 00360 #define ENOBUFS 105 /* No buffer space available */ 00361 #undef EISCONN 00362 #define EISCONN 106 /* Transport endpoint is already connected */ 00363 #undef ENOTCONN 00364 #define ENOTCONN 107 /* Transport endpoint is not connected */ 00365 #undef ESHUTDOWN 00366 #define ESHUTDOWN 108 /* Cannot send after transport endpoint shutdown */ 00367 #undef ETOOMANYREFS 00368 #define ETOOMANYREFS 109 /* Too many references: cannot splice */ 00369 #undef ETIMEDOUT 00370 #define ETIMEDOUT 110 /* Connection timed out */ 00371 #undef ECONNREFUSED 00372 #define ECONNREFUSED 111 /* Connection refused */ 00373 #undef EHOSTDOWN 00374 #define EHOSTDOWN 112 /* Host is down */ 00375 #undef EHOSTUNREACH 00376 #define EHOSTUNREACH 113 /* No route to host */ 00377 #undef EALREADY 00378 #define EALREADY 114 /* Operation already in progress */ 00379 #undef EINPROGRESS 00380 #define EINPROGRESS 115 /* Operation now in progress */ 00381 #undef ESTALE 00382 #define ESTALE 116 /* Stale NFS file handle */ 00383 #undef EUCLEAN 00384 #define EUCLEAN 117 /* Structure needs cleaning */ 00385 #undef ENOTNAM 00386 #define ENOTNAM 118 /* Not a XENIX named type file */ 00387 #undef ENAVAIL 00388 #define ENAVAIL 119 /* No XENIX semaphores available */ 00389 #undef EISNAM 00390 #define EISNAM 120 /* Is a named type file */ 00391 #undef EREMOTEIO 00392 #define EREMOTEIO 121 /* Remote I/O error */ 00393 #undef EDQUOT 00394 #define EDQUOT 122 /* Quota exceeded */ 00395 #undef ENOMEDIUM 00396 #define ENOMEDIUM 123 /* No medium found */ 00397 #undef EMEDIUMTYPE 00398 #define EMEDIUMTYPE 124 /* Wrong medium type */ 00399 #undef ECANCELED 00400 #define ECANCELED 125 /* Operation Canceled */ 00401 #undef ENOKEY 00402 #define ENOKEY 126 /* Required key not available */ 00403 #undef EKEYEXPIRED 00404 #define EKEYEXPIRED 127 /* Key has expired */ 00405 #undef EKEYREVOKED 00406 #define EKEYREVOKED 128 /* Key has been revoked */ 00407 #undef EKEYREJECTED 00408 #define EKEYREJECTED 129 /* Key was rejected by service */ 00409 #undef EOWNERDEAD 00410 #define EOWNERDEAD 130 /* Owner died */ 00411 #undef ENOTRECOVERABLE 00412 #define ENOTRECOVERABLE 131 /* State not recoverable */ 00413 00414 /* Missing stat.h defines. 00415 * The following are sys/stat.h definitions not currently present in the ARMCC 00416 * errno.h. Note, ARMCC errno.h defines some symbol values differing from 00417 * GCC_ARM/IAR/standard POSIX definitions. Guard against this and future 00418 * changes by changing the symbol definition for filesystem use. 00419 */ 00420 #define _IFMT 0170000 //< type of file 00421 #define _IFSOCK 0140000 //< socket 00422 #define _IFLNK 0120000 //< symbolic link 00423 #define _IFREG 0100000 //< regular 00424 #define _IFBLK 0060000 //< block special 00425 #define _IFDIR 0040000 //< directory 00426 #define _IFCHR 0020000 //< character special 00427 #define _IFIFO 0010000 //< fifo special 00428 00429 #define S_IFMT _IFMT //< type of file 00430 #define S_IFSOCK _IFSOCK //< socket 00431 #define S_IFLNK _IFLNK //< symbolic link 00432 #define S_IFREG _IFREG //< regular 00433 #define S_IFBLK _IFBLK //< block special 00434 #define S_IFDIR _IFDIR //< directory 00435 #define S_IFCHR _IFCHR //< character special 00436 #define S_IFIFO _IFIFO //< fifo special 00437 00438 #define S_IRWXU (S_IRUSR | S_IWUSR | S_IXUSR) 00439 #define S_IRUSR 0000400 ///< read permission, owner 00440 #define S_IWUSR 0000200 ///< write permission, owner 00441 #define S_IXUSR 0000100 ///< execute/search permission, owner 00442 #define S_IRWXG (S_IRGRP | S_IWGRP | S_IXGRP) 00443 #define S_IRGRP 0000040 ///< read permission, group 00444 #define S_IWGRP 0000020 ///< write permission, group 00445 #define S_IXGRP 0000010 ///< execute/search permission, group 00446 #define S_IRWXO (S_IROTH | S_IWOTH | S_IXOTH) 00447 #define S_IROTH 0000004 ///< read permission, other 00448 #define S_IWOTH 0000002 ///< write permission, other 00449 #define S_IXOTH 0000001 ///< execute/search permission, other 00450 00451 /* Refer to sys/stat standard 00452 * Note: Not all fields may be supported by the underlying filesystem 00453 */ 00454 struct stat { 00455 dev_t st_dev; ///< Device ID containing file 00456 ino_t st_ino; ///< File serial number 00457 mode_t st_mode; ///< Mode of file 00458 nlink_t st_nlink; ///< Number of links to file 00459 00460 uid_t st_uid; ///< User ID 00461 gid_t st_gid; ///< Group ID 00462 00463 off_t st_size; ///< Size of file in bytes 00464 00465 time_t st_atime; ///< Time of last access 00466 time_t st_mtime; ///< Time of last data modification 00467 time_t st_ctime; ///< Time of last status change 00468 }; 00469 00470 struct statvfs { 00471 unsigned long f_bsize; ///< Filesystem block size 00472 unsigned long f_frsize; ///< Fragment size (block size) 00473 00474 fsblkcnt_t f_blocks; ///< Number of blocks 00475 fsblkcnt_t f_bfree; ///< Number of free blocks 00476 fsblkcnt_t f_bavail; ///< Number of free blocks for unprivileged users 00477 00478 unsigned long f_fsid; ///< Filesystem ID 00479 00480 unsigned long f_namemax; ///< Maximum filename length 00481 }; 00482 00483 /* The following are dirent.h definitions are declared here to guarantee 00484 * consistency where structure may be different with different toolchains 00485 */ 00486 struct dirent { 00487 char d_name[NAME_MAX + 1]; ///< Name of file 00488 uint8_t d_type; ///< Type of file 00489 }; 00490 00491 enum { 00492 DT_UNKNOWN, ///< The file type could not be determined. 00493 DT_FIFO, ///< This is a named pipe (FIFO). 00494 DT_CHR, ///< This is a character device. 00495 DT_DIR, ///< This is a directory. 00496 DT_BLK, ///< This is a block device. 00497 DT_REG, ///< This is a regular file. 00498 DT_LNK, ///< This is a symbolic link. 00499 DT_SOCK, ///< This is a UNIX domain socket. 00500 }; 00501 00502 /* fcntl.h defines */ 00503 #define F_GETFL 3 00504 #define F_SETFL 4 00505 00506 struct pollfd { 00507 int fd; 00508 short events; 00509 short revents; 00510 }; 00511 00512 /* POSIX-compatible I/O functions */ 00513 #if __cplusplus 00514 extern "C" { 00515 #endif 00516 int open(const char *path, int oflag, ...); 00517 #ifndef __IAR_SYSTEMS_ICC__ /* IAR provides fdopen itself */ 00518 #if __cplusplus 00519 std::FILE *fdopen(int fildes, const char *mode); 00520 #else 00521 FILE *fdopen(int fildes, const char *mode); 00522 #endif 00523 #endif 00524 ssize_t write(int fildes, const void *buf, size_t nbyte); 00525 ssize_t read(int fildes, void *buf, size_t nbyte); 00526 off_t lseek(int fildes, off_t offset, int whence); 00527 int isatty(int fildes); 00528 int fsync(int fildes); 00529 int fstat(int fildes, struct stat *st); 00530 int fcntl(int fildes, int cmd, ...); 00531 int poll(struct pollfd fds[], nfds_t nfds, int timeout); 00532 int close(int fildes); 00533 int stat(const char *path, struct stat *st); 00534 int statvfs(const char *path, struct statvfs *buf); 00535 DIR *opendir(const char *); 00536 struct dirent *readdir(DIR *); 00537 int closedir(DIR *); 00538 void rewinddir(DIR *); 00539 long telldir(DIR *); 00540 void seekdir(DIR *, long); 00541 int mkdir(const char *name, mode_t n); 00542 #if __cplusplus 00543 }; // extern "C" 00544 00545 namespace mbed { 00546 00547 /** This call is an analogue to POSIX fdopen(). 00548 * 00549 * It associates a C stream to an already-opened FileHandle, to allow you to 00550 * use C printf/scanf/fwrite etc. The provided FileHandle must remain open - 00551 * it will be closed by the C library when fclose(FILE) is called. 00552 * 00553 * The net effect is fdopen(bind_to_fd(fh), mode), with error handling. 00554 * 00555 * @param fh a pointer to an opened FileHandle 00556 * @param mode operation upon the file descriptor, e.g., "w+" 00557 * 00558 * @returns a pointer to FILE 00559 */ 00560 std::FILE *fdopen(mbed::FileHandle *fh, const char *mode); 00561 00562 /** Bind an mbed FileHandle to a POSIX file descriptor 00563 * 00564 * This is similar to fdopen, but only operating at the POSIX layer - it 00565 * associates a POSIX integer file descriptor with a FileHandle, to allow you 00566 * to use POSIX read/write calls etc. The provided FileHandle must remain open - 00567 * it will be closed when close(int) is called. 00568 * 00569 * @param fh a pointer to an opened FileHandle 00570 * 00571 * @return an integer file descriptor, or negative if no descriptors available 00572 */ 00573 int bind_to_fd(mbed::FileHandle *fh); 00574 00575 } // namespace mbed 00576 00577 #endif // __cplusplus 00578 00579 /**@}*/ 00580 00581 /**@}*/ 00582 00583 #endif /* RETARGET_H */
Generated on Tue Jul 12 2022 20:41:15 by
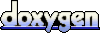