mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
mbed_sdk_boot.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #include "mbed_toolchain.h" 00019 #include <stdlib.h> 00020 #include <stdint.h> 00021 #include "cmsis.h" 00022 00023 /* This startup is for mbed 2 baremetal. There is no config for RTOS for mbed 2, 00024 * therefore we protect this file with MBED_CONF_RTOS_PRESENT 00025 * Note: The new consolidated started for mbed OS is in rtos/mbed_boot code file. 00026 */ 00027 #if !defined(MBED_CONF_RTOS_PRESENT) 00028 00029 /* mbed_main is a function that is called before main() 00030 * mbed_sdk_init() is also a function that is called before main(), but unlike 00031 * mbed_main(), it is not meant for user code, but for the SDK itself to perform 00032 * initializations before main() is called. 00033 */ 00034 MBED_WEAK void mbed_main(void) 00035 { 00036 00037 } 00038 00039 /* This function can be implemented by the target to perform higher level target initialization 00040 */ 00041 MBED_WEAK void mbed_sdk_init(void) 00042 { 00043 00044 } 00045 00046 MBED_WEAK void software_init_hook_rtos() 00047 { 00048 // Nothing by default 00049 } 00050 00051 void mbed_copy_nvic(void) 00052 { 00053 /* If vector address in RAM is defined, copy and switch to dynamic vectors. Exceptions for M0 which doesn't have 00054 VTOR register and for A9 for which CMSIS doesn't define NVIC_SetVector; in both cases target code is 00055 responsible for correctly handling the vectors. 00056 */ 00057 #if !defined(__CORTEX_M0) && !defined(__CORTEX_A9) 00058 #ifdef NVIC_RAM_VECTOR_ADDRESS 00059 uint32_t *old_vectors = (uint32_t *)SCB->VTOR; 00060 uint32_t *vectors = (uint32_t *)NVIC_RAM_VECTOR_ADDRESS; 00061 for (int i = 0; i < NVIC_NUM_VECTORS; i++) { 00062 vectors[i] = old_vectors[i]; 00063 } 00064 SCB->VTOR = (uint32_t)NVIC_RAM_VECTOR_ADDRESS; 00065 #endif /* NVIC_RAM_VECTOR_ADDRESS */ 00066 #endif /* !defined(__CORTEX_M0) && !defined(__CORTEX_A9) */ 00067 } 00068 00069 /* Toolchain specific main code */ 00070 00071 #if defined (__CC_ARM) || (defined(__ARMCC_VERSION) && (__ARMCC_VERSION >= 5010060)) 00072 00073 int $Super$$main(void); 00074 00075 int $Sub$$main(void) 00076 { 00077 mbed_main(); 00078 return $Super$$main(); 00079 } 00080 00081 void _platform_post_stackheap_init(void) 00082 { 00083 mbed_copy_nvic(); 00084 mbed_sdk_init(); 00085 } 00086 00087 #elif defined (__GNUC__) 00088 00089 extern int __real_main(void); 00090 00091 void software_init_hook(void) 00092 { 00093 mbed_copy_nvic(); 00094 mbed_sdk_init(); 00095 software_init_hook_rtos(); 00096 } 00097 00098 00099 int __wrap_main(void) 00100 { 00101 mbed_main(); 00102 return __real_main(); 00103 } 00104 00105 #elif defined (__ICCARM__) 00106 00107 int __low_level_init(void) 00108 { 00109 mbed_copy_nvic(); 00110 return 1; 00111 } 00112 00113 #endif 00114 00115 #endif
Generated on Tue Jul 12 2022 20:41:15 by
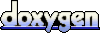