mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
mbed_mktime.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #include "mbed_mktime.h" 00019 00020 /* Time constants. */ 00021 #define SECONDS_BY_MINUTES 60 00022 #define MINUTES_BY_HOUR 60 00023 #define SECONDS_BY_HOUR (SECONDS_BY_MINUTES * MINUTES_BY_HOUR) 00024 #define HOURS_BY_DAY 24 00025 #define SECONDS_BY_DAY (SECONDS_BY_HOUR * HOURS_BY_DAY) 00026 #define LAST_VALID_YEAR 206 00027 00028 /* Macros which will be used to determine if we are within valid range. */ 00029 #define EDGE_TIMESTAMP_FULL_LEAP_YEAR_SUPPORT 3220095 // 7th of February 1970 at 06:28:15 00030 #define EDGE_TIMESTAMP_4_YEAR_LEAP_YEAR_SUPPORT 3133695 // 6th of February 1970 at 06:28:15 00031 00032 /* 00033 * 2 dimensional array containing the number of seconds elapsed before a given 00034 * month. 00035 * The second index map to the month while the first map to the type of year: 00036 * - 0: non leap year 00037 * - 1: leap year 00038 */ 00039 static const uint32_t seconds_before_month[2][12] = { 00040 { 00041 0, 00042 31 * SECONDS_BY_DAY, 00043 (31 + 28) *SECONDS_BY_DAY, 00044 (31 + 28 + 31) *SECONDS_BY_DAY, 00045 (31 + 28 + 31 + 30) *SECONDS_BY_DAY, 00046 (31 + 28 + 31 + 30 + 31) *SECONDS_BY_DAY, 00047 (31 + 28 + 31 + 30 + 31 + 30) *SECONDS_BY_DAY, 00048 (31 + 28 + 31 + 30 + 31 + 30 + 31) *SECONDS_BY_DAY, 00049 (31 + 28 + 31 + 30 + 31 + 30 + 31 + 31) *SECONDS_BY_DAY, 00050 (31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30) *SECONDS_BY_DAY, 00051 (31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31) *SECONDS_BY_DAY, 00052 (31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30) *SECONDS_BY_DAY, 00053 }, 00054 { 00055 0, 00056 31 * SECONDS_BY_DAY, 00057 (31 + 29) *SECONDS_BY_DAY, 00058 (31 + 29 + 31) *SECONDS_BY_DAY, 00059 (31 + 29 + 31 + 30) *SECONDS_BY_DAY, 00060 (31 + 29 + 31 + 30 + 31) *SECONDS_BY_DAY, 00061 (31 + 29 + 31 + 30 + 31 + 30) *SECONDS_BY_DAY, 00062 (31 + 29 + 31 + 30 + 31 + 30 + 31) *SECONDS_BY_DAY, 00063 (31 + 29 + 31 + 30 + 31 + 30 + 31 + 31) *SECONDS_BY_DAY, 00064 (31 + 29 + 31 + 30 + 31 + 30 + 31 + 31 + 30) *SECONDS_BY_DAY, 00065 (31 + 29 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31) *SECONDS_BY_DAY, 00066 (31 + 29 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30) *SECONDS_BY_DAY, 00067 } 00068 }; 00069 00070 bool _rtc_is_leap_year(int year, rtc_leap_year_support_t leap_year_support) 00071 { 00072 /* 00073 * since in practice, the value manipulated by this algorithm lie in the 00074 * range: [70 : 206] the algorithm can be reduced to: year % 4 with exception for 200 (year 2100 is not leap year). 00075 * The algorithm valid over the full range of value is: 00076 00077 year = 1900 + year; 00078 if (year % 4) { 00079 return false; 00080 } else if (year % 100) { 00081 return true; 00082 } else if (year % 400) { 00083 return false; 00084 } 00085 return true; 00086 00087 */ 00088 if (leap_year_support == RTC_FULL_LEAP_YEAR_SUPPORT && year == 200) { 00089 return false; // 2100 is not a leap year 00090 } 00091 00092 return (year) % 4 ? false : true; 00093 } 00094 00095 bool _rtc_maketime(const struct tm *time, time_t *seconds, rtc_leap_year_support_t leap_year_support) 00096 { 00097 if (seconds == NULL || time == NULL) { 00098 return false; 00099 } 00100 00101 /* Partial check for the upper bound of the range - check years only. Full check will be performed after the 00102 * elapsed time since the beginning of the year is calculated. 00103 */ 00104 if ((time->tm_year < 70) || (time->tm_year > LAST_VALID_YEAR)) { 00105 return false; 00106 } 00107 00108 uint32_t result = time->tm_sec; 00109 result += time->tm_min * SECONDS_BY_MINUTES; 00110 result += time->tm_hour * SECONDS_BY_HOUR; 00111 result += (time->tm_mday - 1) * SECONDS_BY_DAY; 00112 result += seconds_before_month[_rtc_is_leap_year(time->tm_year, leap_year_support)][time->tm_mon]; 00113 00114 /* Check if we are within valid range. */ 00115 if (time->tm_year == LAST_VALID_YEAR) { 00116 if ((leap_year_support == RTC_FULL_LEAP_YEAR_SUPPORT && result > EDGE_TIMESTAMP_FULL_LEAP_YEAR_SUPPORT) || 00117 (leap_year_support == RTC_4_YEAR_LEAP_YEAR_SUPPORT && result > EDGE_TIMESTAMP_4_YEAR_LEAP_YEAR_SUPPORT)) { 00118 return false; 00119 } 00120 } 00121 00122 if (time->tm_year > 70) { 00123 /* Valid in the range [70:206]. */ 00124 uint32_t count_of_leap_days = ((time->tm_year - 1) / 4) - (70 / 4); 00125 if (leap_year_support == RTC_FULL_LEAP_YEAR_SUPPORT) { 00126 if (time->tm_year > 200) { 00127 count_of_leap_days--; // 2100 is not a leap year 00128 } 00129 } 00130 00131 result += (((time->tm_year - 70) * 365) + count_of_leap_days) * SECONDS_BY_DAY; 00132 } 00133 00134 *seconds = result; 00135 00136 return true; 00137 } 00138 00139 bool _rtc_localtime(time_t timestamp, struct tm *time_info, rtc_leap_year_support_t leap_year_support) 00140 { 00141 if (time_info == NULL) { 00142 return false; 00143 } 00144 00145 uint32_t seconds = (uint32_t)timestamp; 00146 00147 time_info->tm_sec = seconds % 60; 00148 seconds = seconds / 60; // timestamp in minutes 00149 time_info->tm_min = seconds % 60; 00150 seconds = seconds / 60; // timestamp in hours 00151 time_info->tm_hour = seconds % 24; 00152 seconds = seconds / 24; // timestamp in days; 00153 00154 /* Compute the weekday. 00155 * The 1st of January 1970 was a Thursday which is equal to 4 in the weekday representation ranging from [0:6]. 00156 */ 00157 time_info->tm_wday = (seconds + 4) % 7; 00158 00159 /* Years start at 70. */ 00160 time_info->tm_year = 70; 00161 while (true) { 00162 if (_rtc_is_leap_year(time_info->tm_year, leap_year_support) && seconds >= 366) { 00163 ++time_info->tm_year; 00164 seconds -= 366; 00165 } else if (!_rtc_is_leap_year(time_info->tm_year, leap_year_support) && seconds >= 365) { 00166 ++time_info->tm_year; 00167 seconds -= 365; 00168 } else { 00169 /* The remaining days are less than a years. */ 00170 break; 00171 } 00172 } 00173 00174 time_info->tm_yday = seconds; 00175 00176 /* Convert days into seconds and find the current month. */ 00177 seconds *= SECONDS_BY_DAY; 00178 time_info->tm_mon = 11; 00179 bool leap = _rtc_is_leap_year(time_info->tm_year, leap_year_support); 00180 for (uint32_t i = 0; i < 12; ++i) { 00181 if ((uint32_t) seconds < seconds_before_month[leap][i]) { 00182 time_info->tm_mon = i - 1; 00183 break; 00184 } 00185 } 00186 00187 /* Remove month from timestamp and compute the number of days. 00188 * Note: unlike other fields, days are not 0 indexed. 00189 */ 00190 seconds -= seconds_before_month[leap][time_info->tm_mon]; 00191 time_info->tm_mday = (seconds / SECONDS_BY_DAY) + 1; 00192 00193 return true; 00194 }
Generated on Tue Jul 12 2022 20:41:15 by
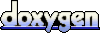