mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
spi_api.h
00001 00002 /** \addtogroup hal */ 00003 /** @{*/ 00004 /* mbed Microcontroller Library 00005 * Copyright (c) 2006-2013 ARM Limited 00006 * SPDX-License-Identifier: Apache-2.0 00007 * 00008 * Licensed under the Apache License, Version 2.0 (the "License"); 00009 * you may not use this file except in compliance with the License. 00010 * You may obtain a copy of the License at 00011 * 00012 * http://www.apache.org/licenses/LICENSE-2.0 00013 * 00014 * Unless required by applicable law or agreed to in writing, software 00015 * distributed under the License is distributed on an "AS IS" BASIS, 00016 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 #ifndef MBED_SPI_API_H 00021 #define MBED_SPI_API_H 00022 00023 #include "device.h" 00024 #include "hal/dma_api.h" 00025 #include "hal/buffer.h" 00026 00027 #if DEVICE_SPI 00028 00029 #define SPI_EVENT_ERROR (1 << 1) 00030 #define SPI_EVENT_COMPLETE (1 << 2) 00031 #define SPI_EVENT_RX_OVERFLOW (1 << 3) 00032 #define SPI_EVENT_ALL (SPI_EVENT_ERROR | SPI_EVENT_COMPLETE | SPI_EVENT_RX_OVERFLOW) 00033 00034 #define SPI_EVENT_INTERNAL_TRANSFER_COMPLETE (1 << 30) // Internal flag to report that an event occurred 00035 00036 #define SPI_FILL_WORD (0xFFFF) 00037 #define SPI_FILL_CHAR (0xFF) 00038 00039 #if DEVICE_SPI_ASYNCH 00040 /** Asynch SPI HAL structure 00041 */ 00042 typedef struct { 00043 struct spi_s spi; /**< Target specific SPI structure */ 00044 struct buffer_s tx_buff; /**< Tx buffer */ 00045 struct buffer_s rx_buff; /**< Rx buffer */ 00046 } spi_t; 00047 00048 #else 00049 /** Non-asynch SPI HAL structure 00050 */ 00051 typedef struct spi_s spi_t; 00052 00053 #endif 00054 00055 #ifdef __cplusplus 00056 extern "C" { 00057 #endif 00058 00059 /** 00060 * \defgroup hal_GeneralSPI SPI Configuration Functions 00061 * @{ 00062 */ 00063 00064 /** Initialize the SPI peripheral 00065 * 00066 * Configures the pins used by SPI, sets a default format and frequency, and enables the peripheral 00067 * @param[out] obj The SPI object to initialize 00068 * @param[in] mosi The pin to use for MOSI 00069 * @param[in] miso The pin to use for MISO 00070 * @param[in] sclk The pin to use for SCLK 00071 * @param[in] ssel The pin to use for SSEL 00072 */ 00073 void spi_init(spi_t *obj, PinName mosi, PinName miso, PinName sclk, PinName ssel); 00074 00075 /** Release a SPI object 00076 * 00077 * TODO: spi_free is currently unimplemented 00078 * This will require reference counting at the C++ level to be safe 00079 * 00080 * Return the pins owned by the SPI object to their reset state 00081 * Disable the SPI peripheral 00082 * Disable the SPI clock 00083 * @param[in] obj The SPI object to deinitialize 00084 */ 00085 void spi_free(spi_t *obj); 00086 00087 /** Configure the SPI format 00088 * 00089 * Set the number of bits per frame, configure clock polarity and phase, shift order and master/slave mode. 00090 * The default bit order is MSB. 00091 * @param[in,out] obj The SPI object to configure 00092 * @param[in] bits The number of bits per frame 00093 * @param[in] mode The SPI mode (clock polarity, phase, and shift direction) 00094 * @param[in] slave Zero for master mode or non-zero for slave mode 00095 */ 00096 void spi_format(spi_t *obj, int bits, int mode, int slave); 00097 00098 /** Set the SPI baud rate 00099 * 00100 * Actual frequency may differ from the desired frequency due to available dividers and bus clock 00101 * Configures the SPI peripheral's baud rate 00102 * @param[in,out] obj The SPI object to configure 00103 * @param[in] hz The baud rate in Hz 00104 */ 00105 void spi_frequency(spi_t *obj, int hz); 00106 00107 /**@}*/ 00108 /** 00109 * \defgroup SynchSPI Synchronous SPI Hardware Abstraction Layer 00110 * @{ 00111 */ 00112 00113 /** Write a byte out in master mode and receive a value 00114 * 00115 * @param[in] obj The SPI peripheral to use for sending 00116 * @param[in] value The value to send 00117 * @return Returns the value received during send 00118 */ 00119 int spi_master_write(spi_t *obj, int value); 00120 00121 /** Write a block out in master mode and receive a value 00122 * 00123 * The total number of bytes sent and received will be the maximum of 00124 * tx_length and rx_length. The bytes written will be padded with the 00125 * value 0xff. 00126 * 00127 * @param[in] obj The SPI peripheral to use for sending 00128 * @param[in] tx_buffer Pointer to the byte-array of data to write to the device 00129 * @param[in] tx_length Number of bytes to write, may be zero 00130 * @param[in] rx_buffer Pointer to the byte-array of data to read from the device 00131 * @param[in] rx_length Number of bytes to read, may be zero 00132 * @param[in] write_fill Default data transmitted while performing a read 00133 * @returns 00134 * The number of bytes written and read from the device. This is 00135 * maximum of tx_length and rx_length. 00136 */ 00137 int spi_master_block_write(spi_t *obj, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, char write_fill); 00138 00139 /** Check if a value is available to read 00140 * 00141 * @param[in] obj The SPI peripheral to check 00142 * @return non-zero if a value is available 00143 */ 00144 int spi_slave_receive(spi_t *obj); 00145 00146 /** Get a received value out of the SPI receive buffer in slave mode 00147 * 00148 * Blocks until a value is available 00149 * @param[in] obj The SPI peripheral to read 00150 * @return The value received 00151 */ 00152 int spi_slave_read(spi_t *obj); 00153 00154 /** Write a value to the SPI peripheral in slave mode 00155 * 00156 * Blocks until the SPI peripheral can be written to 00157 * @param[in] obj The SPI peripheral to write 00158 * @param[in] value The value to write 00159 */ 00160 void spi_slave_write(spi_t *obj, int value); 00161 00162 /** Checks if the specified SPI peripheral is in use 00163 * 00164 * @param[in] obj The SPI peripheral to check 00165 * @return non-zero if the peripheral is currently transmitting 00166 */ 00167 int spi_busy(spi_t *obj); 00168 00169 /** Get the module number 00170 * 00171 * @param[in] obj The SPI peripheral to check 00172 * @return The module number 00173 */ 00174 uint8_t spi_get_module(spi_t *obj); 00175 00176 /**@}*/ 00177 00178 #if DEVICE_SPI_ASYNCH 00179 /** 00180 * \defgroup AsynchSPI Asynchronous SPI Hardware Abstraction Layer 00181 * @{ 00182 */ 00183 00184 /** Begin the SPI transfer. Buffer pointers and lengths are specified in tx_buff and rx_buff 00185 * 00186 * @param[in] obj The SPI object that holds the transfer information 00187 * @param[in] tx The transmit buffer 00188 * @param[in] tx_length The number of bytes to transmit 00189 * @param[in] rx The receive buffer 00190 * @param[in] rx_length The number of bytes to receive 00191 * @param[in] bit_width The bit width of buffer words 00192 * @param[in] event The logical OR of events to be registered 00193 * @param[in] handler SPI interrupt handler 00194 * @param[in] hint A suggestion for how to use DMA with this transfer 00195 */ 00196 void spi_master_transfer(spi_t *obj, const void *tx, size_t tx_length, void *rx, size_t rx_length, uint8_t bit_width, uint32_t handler, uint32_t event, DMAUsage hint); 00197 00198 /** The asynchronous IRQ handler 00199 * 00200 * Reads the received values out of the RX FIFO, writes values into the TX FIFO and checks for transfer termination 00201 * conditions, such as buffer overflows or transfer complete. 00202 * @param[in] obj The SPI object that holds the transfer information 00203 * @return Event flags if a transfer termination condition was met; otherwise 0. 00204 */ 00205 uint32_t spi_irq_handler_asynch(spi_t *obj); 00206 00207 /** Attempts to determine if the SPI peripheral is already in use 00208 * 00209 * If a temporary DMA channel has been allocated, peripheral is in use. 00210 * If a permanent DMA channel has been allocated, check if the DMA channel is in use. If not, proceed as though no DMA 00211 * channel were allocated. 00212 * If no DMA channel is allocated, check whether tx and rx buffers have been assigned. For each assigned buffer, check 00213 * if the corresponding buffer position is less than the buffer length. If buffers do not indicate activity, check if 00214 * there are any bytes in the FIFOs. 00215 * @param[in] obj The SPI object to check for activity 00216 * @return Non-zero if the SPI port is active or zero if it is not. 00217 */ 00218 uint8_t spi_active(spi_t *obj); 00219 00220 /** Abort an SPI transfer 00221 * 00222 * @param obj The SPI peripheral to stop 00223 */ 00224 void spi_abort_asynch(spi_t *obj); 00225 00226 00227 #endif 00228 00229 /**@}*/ 00230 00231 #ifdef __cplusplus 00232 } 00233 #endif // __cplusplus 00234 00235 #endif // SPI_DEVICE 00236 00237 #endif // MBED_SPI_API_H 00238 00239 /** @}*/
Generated on Tue Jul 12 2022 20:41:16 by
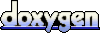