mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
SerialBase.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef MBED_SERIALBASE_H 00018 #define MBED_SERIALBASE_H 00019 00020 #include "platform/platform.h" 00021 00022 #if DEVICE_SERIAL || defined(DOXYGEN_ONLY) 00023 00024 #include "platform/Callback.h" 00025 #include "hal/serial_api.h" 00026 #include "platform/mbed_toolchain.h" 00027 #include "platform/NonCopyable.h" 00028 00029 #if DEVICE_SERIAL_ASYNCH 00030 #include "platform/CThunk.h" 00031 #include "hal/dma_api.h" 00032 #endif 00033 00034 namespace mbed { 00035 /** \addtogroup drivers */ 00036 00037 /** A base class for serial port implementations 00038 * Can't be instantiated directly (use Serial or RawSerial) 00039 * 00040 * @note Synchronization level: Set by subclass 00041 * @ingroup drivers 00042 */ 00043 class SerialBase : private NonCopyable<SerialBase> { 00044 00045 public: 00046 /** Set the baud rate of the serial port 00047 * 00048 * @param baudrate The baudrate of the serial port (default = 9600). 00049 */ 00050 void baud(int baudrate); 00051 00052 enum Parity { 00053 None = 0, 00054 Odd, 00055 Even, 00056 Forced1, 00057 Forced0 00058 }; 00059 00060 enum IrqType { 00061 RxIrq = 0, 00062 TxIrq, 00063 00064 IrqCnt 00065 }; 00066 00067 enum Flow { 00068 Disabled = 0, 00069 RTS, 00070 CTS, 00071 RTSCTS 00072 }; 00073 00074 /** Set the transmission format used by the serial port 00075 * 00076 * @param bits The number of bits in a word (5-8; default = 8) 00077 * @param parity The parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) 00078 * @param stop_bits The number of stop bits (1 or 2; default = 1) 00079 */ 00080 void format(int bits = 8, Parity parity = SerialBase::None, int stop_bits = 1); 00081 00082 /** Determine if there is a character available to read 00083 * 00084 * @returns 00085 * 1 if there is a character available to read, 00086 * 0 otherwise 00087 */ 00088 int readable(); 00089 00090 /** Determine if there is space available to write a character 00091 * 00092 * @returns 00093 * 1 if there is space to write a character, 00094 * 0 otherwise 00095 */ 00096 int writeable(); 00097 00098 /** Attach a function to call whenever a serial interrupt is generated 00099 * 00100 * @param func A pointer to a void function, or 0 to set as none 00101 * @param type Which serial interrupt to attach the member function to (Serial::RxIrq for receive, TxIrq for transmit buffer empty) 00102 */ 00103 void attach(Callback<void()> func, IrqType type = RxIrq); 00104 00105 /** Attach a member function to call whenever a serial interrupt is generated 00106 * 00107 * @param obj pointer to the object to call the member function on 00108 * @param method pointer to the member function to be called 00109 * @param type Which serial interrupt to attach the member function to (Serial::RxIrq for receive, TxIrq for transmit buffer empty) 00110 * @deprecated 00111 * The attach function does not support cv-qualifiers. Replaced by 00112 * attach(callback(obj, method), type). 00113 */ 00114 template<typename T> 00115 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00116 "The attach function does not support cv-qualifiers. Replaced by " 00117 "attach(callback(obj, method), type).") 00118 void attach(T *obj, void (T::*method)(), IrqType type = RxIrq) 00119 { 00120 attach(callback(obj, method), type); 00121 } 00122 00123 /** Attach a member function to call whenever a serial interrupt is generated 00124 * 00125 * @param obj pointer to the object to call the member function on 00126 * @param method pointer to the member function to be called 00127 * @param type Which serial interrupt to attach the member function to (Serial::RxIrq for receive, TxIrq for transmit buffer empty) 00128 * @deprecated 00129 * The attach function does not support cv-qualifiers. Replaced by 00130 * attach(callback(obj, method), type). 00131 */ 00132 template<typename T> 00133 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00134 "The attach function does not support cv-qualifiers. Replaced by " 00135 "attach(callback(obj, method), type).") 00136 void attach(T *obj, void (*method)(T *), IrqType type = RxIrq) 00137 { 00138 attach(callback(obj, method), type); 00139 } 00140 00141 /** Generate a break condition on the serial line 00142 * NOTE: Clear break needs to run at least one frame after set_break is called 00143 */ 00144 void set_break(); 00145 00146 /** Clear a break condition on the serial line 00147 * NOTE: Should be run at least one frame after set_break is called 00148 */ 00149 void clear_break(); 00150 00151 /** Generate a break condition on the serial line 00152 */ 00153 void send_break(); 00154 00155 #if !defined(DOXYGEN_ONLY) 00156 protected: 00157 00158 /** Acquire exclusive access to this serial port 00159 */ 00160 virtual void lock(void); 00161 00162 /** Release exclusive access to this serial port 00163 */ 00164 virtual void unlock(void); 00165 #endif 00166 public: 00167 00168 #if DEVICE_SERIAL_FC 00169 /** Set the flow control type on the serial port 00170 * 00171 * @param type the flow control type (Disabled, RTS, CTS, RTSCTS) 00172 * @param flow1 the first flow control pin (RTS for RTS or RTSCTS, CTS for CTS) 00173 * @param flow2 the second flow control pin (CTS for RTSCTS) 00174 */ 00175 void set_flow_control(Flow type, PinName flow1 = NC, PinName flow2 = NC); 00176 #endif 00177 00178 static void _irq_handler(uint32_t id, SerialIrq irq_type); 00179 00180 #if DEVICE_SERIAL_ASYNCH 00181 00182 /** Begin asynchronous write using 8bit buffer. The completion invokes registered TX event callback 00183 * 00184 * This function locks the deep sleep until any event has occurred 00185 * 00186 * @param buffer The buffer where received data will be stored 00187 * @param length The buffer length in bytes 00188 * @param callback The event callback function 00189 * @param event The logical OR of TX events 00190 */ 00191 int write(const uint8_t *buffer, int length, const event_callback_t &callback, int event = SERIAL_EVENT_TX_COMPLETE); 00192 00193 /** Begin asynchronous write using 16bit buffer. The completion invokes registered TX event callback 00194 * 00195 * This function locks the deep sleep until any event has occurred 00196 * 00197 * @param buffer The buffer where received data will be stored 00198 * @param length The buffer length in bytes 00199 * @param callback The event callback function 00200 * @param event The logical OR of TX events 00201 */ 00202 int write(const uint16_t *buffer, int length, const event_callback_t &callback, int event = SERIAL_EVENT_TX_COMPLETE); 00203 00204 /** Abort the on-going write transfer 00205 */ 00206 void abort_write(); 00207 00208 /** Begin asynchronous reading using 8bit buffer. The completion invokes registered RX event callback. 00209 * 00210 * This function locks the deep sleep until any event has occurred 00211 * 00212 * @param buffer The buffer where received data will be stored 00213 * @param length The buffer length in bytes 00214 * @param callback The event callback function 00215 * @param event The logical OR of RX events 00216 * @param char_match The matching character 00217 */ 00218 int read(uint8_t *buffer, int length, const event_callback_t &callback, int event = SERIAL_EVENT_RX_COMPLETE, unsigned char char_match = SERIAL_RESERVED_CHAR_MATCH); 00219 00220 /** Begin asynchronous reading using 16bit buffer. The completion invokes registered RX event callback. 00221 * 00222 * This function locks the deep sleep until any event has occurred 00223 * 00224 * @param buffer The buffer where received data will be stored 00225 * @param length The buffer length in bytes 00226 * @param callback The event callback function 00227 * @param event The logical OR of RX events 00228 * @param char_match The matching character 00229 */ 00230 int read(uint16_t *buffer, int length, const event_callback_t &callback, int event = SERIAL_EVENT_RX_COMPLETE, unsigned char char_match = SERIAL_RESERVED_CHAR_MATCH); 00231 00232 /** Abort the on-going read transfer 00233 */ 00234 void abort_read(); 00235 00236 /** Configure DMA usage suggestion for non-blocking TX transfers 00237 * 00238 * @param usage The usage DMA hint for peripheral 00239 * @return Zero if the usage was set, -1 if a transaction is on-going 00240 */ 00241 int set_dma_usage_tx(DMAUsage usage); 00242 00243 /** Configure DMA usage suggestion for non-blocking RX transfers 00244 * 00245 * @param usage The usage DMA hint for peripheral 00246 * @return Zero if the usage was set, -1 if a transaction is on-going 00247 */ 00248 int set_dma_usage_rx(DMAUsage usage); 00249 00250 #if !defined(DOXYGEN_ONLY) 00251 protected: 00252 void start_read(void *buffer, int buffer_size, char buffer_width, const event_callback_t &callback, int event, unsigned char char_match); 00253 void start_write(const void *buffer, int buffer_size, char buffer_width, const event_callback_t &callback, int event); 00254 void interrupt_handler_asynch(void); 00255 #endif 00256 #endif 00257 00258 #if !defined(DOXYGEN_ONLY) 00259 protected: 00260 SerialBase(PinName tx, PinName rx, int baud); 00261 virtual ~SerialBase(); 00262 00263 int _base_getc(); 00264 int _base_putc(int c); 00265 00266 #if DEVICE_SERIAL_ASYNCH 00267 CThunk<SerialBase> _thunk_irq; 00268 DMAUsage _tx_usage; 00269 DMAUsage _rx_usage; 00270 event_callback_t _tx_callback; 00271 event_callback_t _rx_callback; 00272 #endif 00273 00274 serial_t _serial; 00275 Callback<void()> _irq[IrqCnt]; 00276 int _baud; 00277 #endif 00278 }; 00279 00280 } // namespace mbed 00281 00282 #endif 00283 00284 #endif
Generated on Tue Jul 12 2022 20:41:15 by
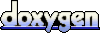