mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
MbedCRC.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2018 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef MBED_CRC_API_H 00018 #define MBED_CRC_API_H 00019 00020 #include "drivers/TableCRC.h" 00021 #include "hal/crc_api.h" 00022 #include "platform/mbed_assert.h" 00023 #include "platform/SingletonPtr.h" 00024 #include "platform/PlatformMutex.h" 00025 00026 /* This is invalid warning from the compiler for below section of code 00027 if ((width < 8) && (NULL == _crc_table)) { 00028 p_crc = (uint32_t)(p_crc << (8 - width)); 00029 } 00030 Compiler warns of the shift operation with width as it is width=(std::uint8_t), 00031 but we check for ( width < 8) before performing shift, so it should not be an issue. 00032 */ 00033 #if defined ( __CC_ARM ) 00034 #pragma diag_suppress 62 // Shift count is negative 00035 #elif defined ( __GNUC__ ) 00036 #pragma GCC diagnostic push 00037 #pragma GCC diagnostic ignored "-Wshift-count-negative" 00038 #elif defined (__ICCARM__) 00039 #pragma diag_suppress=Pe062 // Shift count is negative 00040 #endif 00041 00042 namespace mbed { 00043 /** \addtogroup drivers */ 00044 /** @{*/ 00045 00046 /** CRC object provides CRC generation through hardware/software 00047 * 00048 * ROM polynomial tables for supported polynomials (:: crc_polynomial_t) will be used for 00049 * software CRC computation, if ROM tables are not available then CRC is computed runtime 00050 * bit by bit for all data input. 00051 * @note Synchronization level: Thread safe 00052 * 00053 * @tparam polynomial CRC polynomial value in hex 00054 * @tparam width CRC polynomial width 00055 * 00056 * Example: Compute CRC data 00057 * @code 00058 * 00059 * #include "mbed.h" 00060 * 00061 * int main() { 00062 * MbedCRC<POLY_32BIT_ANSI, 32> ct; 00063 * 00064 * char test[] = "123456789"; 00065 * uint32_t crc = 0; 00066 * 00067 * printf("\nPolynomial = 0x%lx Width = %d \n", ct.get_polynomial(), ct.get_width()); 00068 * 00069 * ct.compute((void *)test, strlen((const char*)test), &crc); 00070 * 00071 * printf("The CRC of data \"123456789\" is : 0x%lx\n", crc); 00072 * return 0; 00073 * } 00074 * @endcode 00075 * Example: Compute CRC with data available in parts 00076 * @code 00077 * 00078 * #include "mbed.h" 00079 * int main() { 00080 * MbedCRC<POLY_32BIT_ANSI, 32> ct; 00081 * 00082 * char test[] = "123456789"; 00083 * uint32_t crc = 0; 00084 * 00085 * printf("\nPolynomial = 0x%lx Width = %d \n", ct.get_polynomial(), ct.get_width()); 00086 * ct.compute_partial_start(&crc); 00087 * ct.compute_partial((void *)&test, 4, &crc); 00088 * ct.compute_partial((void *)&test[4], 5, &crc); 00089 * ct.compute_partial_stop(&crc); 00090 * printf("The CRC of data \"123456789\" is : 0x%lx\n", crc); 00091 * return 0; 00092 * } 00093 * @endcode 00094 * @ingroup drivers 00095 */ 00096 00097 extern SingletonPtr<PlatformMutex> mbed_crc_mutex; 00098 00099 template <uint32_t polynomial = POLY_32BIT_ANSI, uint8_t width = 32> 00100 class MbedCRC { 00101 00102 public: 00103 enum CrcMode { 00104 #if DEVICE_CRC 00105 HARDWARE = 0, 00106 #endif 00107 TABLE = 1, 00108 BITWISE 00109 }; 00110 00111 typedef uint64_t crc_data_size_t; 00112 00113 /** Lifetime of CRC object 00114 * 00115 * @param initial_xor Inital value/seed to Xor 00116 * @param final_xor Final Xor value 00117 * @param reflect_data 00118 * @param reflect_remainder 00119 * @note Default constructor without any arguments is valid only for supported CRC polynomials. :: crc_polynomial_t 00120 * MbedCRC <POLY_7BIT_SD, 7> ct; --- Valid POLY_7BIT_SD 00121 * MbedCRC <0x1021, 16> ct; --- Valid POLY_16BIT_CCITT 00122 * MbedCRC <POLY_16BIT_CCITT, 32> ct; --- Invalid, compilation error 00123 * MbedCRC <POLY_16BIT_CCITT, 32> ct (i,f,rd,rr) Constructor can be used for not supported polynomials 00124 * MbedCRC<POLY_16BIT_CCITT, 16> sd(0, 0, false, false); Constructor can also be used for supported 00125 * polynomials with different intial/final/reflect values 00126 * 00127 */ 00128 MbedCRC(uint32_t initial_xor, uint32_t final_xor, bool reflect_data, bool reflect_remainder) : 00129 _initial_value(initial_xor), _final_xor(final_xor), _reflect_data(reflect_data), 00130 _reflect_remainder(reflect_remainder) 00131 { 00132 mbed_crc_ctor(); 00133 } 00134 MbedCRC(); 00135 virtual ~MbedCRC() 00136 { 00137 // Do nothing 00138 } 00139 00140 /** Compute CRC for the data input 00141 * Compute CRC performs the initialization, computation and collection of 00142 * final CRC. 00143 * 00144 * @param buffer Data bytes 00145 * @param size Size of data 00146 * @param crc CRC is the output value 00147 * @return 0 on success, negative error code on failure 00148 */ 00149 int32_t compute(void *buffer, crc_data_size_t size, uint32_t *crc) 00150 { 00151 MBED_ASSERT(crc != NULL); 00152 int32_t status = 0; 00153 00154 status = compute_partial_start(crc); 00155 if (0 != status) { 00156 unlock(); 00157 return status; 00158 } 00159 00160 status = compute_partial(buffer, size, crc); 00161 if (0 != status) { 00162 unlock(); 00163 return status; 00164 } 00165 00166 status = compute_partial_stop(crc); 00167 if (0 != status) { 00168 *crc = 0; 00169 } 00170 00171 return status; 00172 00173 } 00174 00175 /** Compute partial CRC for the data input. 00176 * 00177 * CRC data if not available fully, CRC can be computed in parts with available data. 00178 * 00179 * In case of hardware, intermediate values and states are saved by hardware. Mutex 00180 * locking is used to serialize access to hardware CRC. 00181 * 00182 * In case of software CRC, previous CRC output should be passed as argument to the 00183 * current compute_partial call. Please note the intermediate CRC value is maintained by 00184 * application and not the driver. 00185 * 00186 * @pre: Call `compute_partial_start` to start the partial CRC calculation. 00187 * @post: Call `compute_partial_stop` to get the final CRC value. 00188 * 00189 * @param buffer Data bytes 00190 * @param size Size of data 00191 * @param crc CRC value is intermediate CRC value filled by API. 00192 * @return 0 on success or a negative error code on failure 00193 * @note: CRC as output in compute_partial is not final CRC value, call `compute_partial_stop` 00194 * to get final correct CRC value. 00195 */ 00196 int32_t compute_partial(void *buffer, crc_data_size_t size, uint32_t *crc) 00197 { 00198 int32_t status = 0; 00199 00200 switch (_mode) { 00201 #if DEVICE_CRC 00202 case HARDWARE: 00203 hal_crc_compute_partial((uint8_t *)buffer, size); 00204 *crc = 0; 00205 break; 00206 #endif 00207 case TABLE: 00208 status = table_compute_partial(buffer, size, crc); 00209 break; 00210 case BITWISE: 00211 status = bitwise_compute_partial(buffer, size, crc); 00212 break; 00213 default: 00214 status = -1; 00215 break; 00216 } 00217 00218 return status; 00219 } 00220 00221 /** Compute partial start, indicate start of partial computation. 00222 * 00223 * This API should be called before performing any partial computation 00224 * with compute_partial API. 00225 * 00226 * @param crc Initial CRC value set by the API 00227 * @return 0 on success or a negative in case of failure 00228 * @note: CRC is an out parameter and must be reused with compute_partial 00229 * and `compute_partial_stop` without any modifications in application. 00230 */ 00231 int32_t compute_partial_start(uint32_t *crc) 00232 { 00233 MBED_ASSERT(crc != NULL); 00234 00235 #if DEVICE_CRC 00236 if (_mode == HARDWARE) { 00237 lock(); 00238 crc_mbed_config_t config; 00239 config.polynomial = polynomial; 00240 config.width = width; 00241 config.initial_xor = _initial_value; 00242 config.final_xor = _final_xor; 00243 config.reflect_in = _reflect_data; 00244 config.reflect_out = _reflect_remainder; 00245 00246 hal_crc_compute_partial_start(&config); 00247 } 00248 #endif 00249 00250 *crc = _initial_value; 00251 return 0; 00252 } 00253 00254 /** Get the final CRC value of partial computation. 00255 * 00256 * CRC value available in partial computation is not correct CRC, as some 00257 * algorithms require remainder to be reflected and final value to be XORed 00258 * This API is used to perform final computation to get correct CRC value. 00259 * 00260 * @param crc CRC result 00261 * @return 0 on success or a negative in case of failure. 00262 */ 00263 int32_t compute_partial_stop(uint32_t *crc) 00264 { 00265 MBED_ASSERT(crc != NULL); 00266 00267 #if DEVICE_CRC 00268 if (_mode == HARDWARE) { 00269 *crc = hal_crc_get_result(); 00270 unlock(); 00271 return 0; 00272 } 00273 #endif 00274 uint32_t p_crc = *crc; 00275 if ((width < 8) && (NULL == _crc_table)) { 00276 p_crc = (uint32_t)(p_crc << (8 - width)); 00277 } 00278 // Optimized algorithm for 32BitANSI does not need additional reflect_remainder 00279 if ((TABLE == _mode) && (POLY_32BIT_REV_ANSI == polynomial)) { 00280 *crc = (p_crc ^ _final_xor) & get_crc_mask(); 00281 } else { 00282 *crc = (reflect_remainder(p_crc) ^ _final_xor) & get_crc_mask(); 00283 } 00284 unlock(); 00285 return 0; 00286 } 00287 00288 /** Get the current CRC polynomial. 00289 * 00290 * @return Polynomial value 00291 */ 00292 uint32_t get_polynomial(void) const 00293 { 00294 return polynomial; 00295 } 00296 00297 /** Get the current CRC width 00298 * 00299 * @return CRC width 00300 */ 00301 uint8_t get_width(void) const 00302 { 00303 return width; 00304 } 00305 00306 #if !defined(DOXYGEN_ONLY) 00307 private: 00308 uint32_t _initial_value; 00309 uint32_t _final_xor; 00310 bool _reflect_data; 00311 bool _reflect_remainder; 00312 uint32_t *_crc_table; 00313 CrcMode _mode; 00314 00315 /** Acquire exclusive access to CRC hardware/software. 00316 */ 00317 void lock() 00318 { 00319 #if DEVICE_CRC 00320 if (_mode == HARDWARE) { 00321 mbed_crc_mutex->lock(); 00322 } 00323 #endif 00324 } 00325 00326 /** Release exclusive access to CRC hardware/software. 00327 */ 00328 virtual void unlock() 00329 { 00330 #if DEVICE_CRC 00331 if (_mode == HARDWARE) { 00332 mbed_crc_mutex->unlock(); 00333 } 00334 #endif 00335 } 00336 00337 /** Get the current CRC data size. 00338 * 00339 * @return CRC data size in bytes 00340 */ 00341 uint8_t get_data_size(void) const 00342 { 00343 return (width <= 8 ? 1 : (width <= 16 ? 2 : 4)); 00344 } 00345 00346 /** Get the top bit of current CRC. 00347 * 00348 * @return Top bit is set high for respective data width of current CRC 00349 * Top bit for CRC width less then 8 bits will be set as 8th bit. 00350 */ 00351 uint32_t get_top_bit(void) const 00352 { 00353 return (width < 8 ? (1u << 7) : (uint32_t)(1ul << (width - 1))); 00354 } 00355 00356 /** Get the CRC data mask. 00357 * 00358 * @return CRC data mask is generated based on current CRC width 00359 */ 00360 uint32_t get_crc_mask(void) const 00361 { 00362 return (width < 8 ? ((1u << 8) - 1) : (uint32_t)((uint64_t)(1ull << width) - 1)); 00363 } 00364 00365 /** Final value of CRC is reflected. 00366 * 00367 * @param data final crc value, which should be reflected 00368 * @return Reflected CRC value 00369 */ 00370 uint32_t reflect_remainder(uint32_t data) const 00371 { 00372 if (_reflect_remainder) { 00373 uint32_t reflection = 0x0; 00374 uint8_t const nBits = (width < 8 ? 8 : width); 00375 00376 for (uint8_t bit = 0; bit < nBits; ++bit) { 00377 if (data & 0x01) { 00378 reflection |= (1 << ((nBits - 1) - bit)); 00379 } 00380 data = (data >> 1); 00381 } 00382 return (reflection); 00383 } else { 00384 return data; 00385 } 00386 } 00387 00388 /** Data bytes are reflected. 00389 * 00390 * @param data value to be reflected 00391 * @return Reflected data value 00392 */ 00393 uint32_t reflect_bytes(uint32_t data) const 00394 { 00395 if (_reflect_data) { 00396 uint32_t reflection = 0x0; 00397 00398 for (uint8_t bit = 0; bit < 8; ++bit) { 00399 if (data & 0x01) { 00400 reflection |= (1 << (7 - bit)); 00401 } 00402 data = (data >> 1); 00403 } 00404 return (reflection); 00405 } else { 00406 return data; 00407 } 00408 } 00409 00410 /** Bitwise CRC computation. 00411 * 00412 * @param buffer data buffer 00413 * @param size size of the data 00414 * @param crc CRC value is filled in, but the value is not the final 00415 * @return 0 on success or a negative error code on failure 00416 */ 00417 int32_t bitwise_compute_partial(const void *buffer, crc_data_size_t size, uint32_t *crc) const 00418 { 00419 MBED_ASSERT(crc != NULL); 00420 00421 const uint8_t *data = static_cast<const uint8_t *>(buffer); 00422 uint32_t p_crc = *crc; 00423 00424 if (width < 8) { 00425 uint8_t data_byte; 00426 for (crc_data_size_t byte = 0; byte < size; byte++) { 00427 data_byte = reflect_bytes(data[byte]); 00428 for (uint8_t bit = 8; bit > 0; --bit) { 00429 p_crc <<= 1; 00430 if ((data_byte ^ p_crc) & get_top_bit()) { 00431 p_crc ^= polynomial; 00432 } 00433 data_byte <<= 1; 00434 } 00435 } 00436 } else { 00437 for (crc_data_size_t byte = 0; byte < size; byte++) { 00438 p_crc ^= (reflect_bytes(data[byte]) << (width - 8)); 00439 00440 // Perform modulo-2 division, a bit at a time 00441 for (uint8_t bit = 8; bit > 0; --bit) { 00442 if (p_crc & get_top_bit()) { 00443 p_crc = (p_crc << 1) ^ polynomial; 00444 } else { 00445 p_crc = (p_crc << 1); 00446 } 00447 } 00448 } 00449 } 00450 *crc = p_crc & get_crc_mask(); 00451 return 0; 00452 } 00453 00454 /** CRC computation using ROM tables. 00455 * 00456 * @param buffer data buffer 00457 * @param size size of the data 00458 * @param crc CRC value is filled in, but the value is not the final 00459 * @return 0 on success or a negative error code on failure 00460 */ 00461 int32_t table_compute_partial(const void *buffer, crc_data_size_t size, uint32_t *crc) const 00462 { 00463 MBED_ASSERT(crc != NULL); 00464 00465 const uint8_t *data = static_cast<const uint8_t *>(buffer); 00466 uint32_t p_crc = *crc; 00467 uint8_t data_byte = 0; 00468 00469 if (width <= 8) { 00470 uint8_t *crc_table = (uint8_t *)_crc_table; 00471 for (crc_data_size_t byte = 0; byte < size; byte++) { 00472 data_byte = reflect_bytes(data[byte]) ^ p_crc; 00473 p_crc = crc_table[data_byte]; 00474 } 00475 } else if (width <= 16) { 00476 uint16_t *crc_table = (uint16_t *)_crc_table; 00477 for (crc_data_size_t byte = 0; byte < size; byte++) { 00478 data_byte = reflect_bytes(data[byte]) ^ (p_crc >> (width - 8)); 00479 p_crc = crc_table[data_byte] ^ (p_crc << 8); 00480 } 00481 } else { 00482 uint32_t *crc_table = (uint32_t *)_crc_table; 00483 if (POLY_32BIT_REV_ANSI == polynomial) { 00484 for (crc_data_size_t i = 0; i < size; i++) { 00485 p_crc = (p_crc >> 4) ^ crc_table[(p_crc ^ (data[i] >> 0)) & 0xf]; 00486 p_crc = (p_crc >> 4) ^ crc_table[(p_crc ^ (data[i] >> 4)) & 0xf]; 00487 } 00488 } else { 00489 for (crc_data_size_t byte = 0; byte < size; byte++) { 00490 data_byte = reflect_bytes(data[byte]) ^ (p_crc >> (width - 8)); 00491 p_crc = crc_table[data_byte] ^ (p_crc << 8); 00492 } 00493 } 00494 } 00495 *crc = p_crc & get_crc_mask(); 00496 return 0; 00497 } 00498 00499 /** Constructor init called from all specialized cases of constructor. 00500 * Note: All constructor common code should be in this function. 00501 */ 00502 void mbed_crc_ctor(void) 00503 { 00504 MBED_STATIC_ASSERT(width <= 32, "Max 32-bit CRC supported"); 00505 00506 #if DEVICE_CRC 00507 if (POLY_32BIT_REV_ANSI == polynomial) { 00508 _crc_table = (uint32_t *)Table_CRC_32bit_Rev_ANSI; 00509 _mode = TABLE; 00510 return; 00511 } 00512 crc_mbed_config_t config; 00513 config.polynomial = polynomial; 00514 config.width = width; 00515 config.initial_xor = _initial_value; 00516 config.final_xor = _final_xor; 00517 config.reflect_in = _reflect_data; 00518 config.reflect_out = _reflect_remainder; 00519 00520 if (hal_crc_is_supported(&config)) { 00521 _mode = HARDWARE; 00522 return; 00523 } 00524 #endif 00525 00526 switch (polynomial) { 00527 case POLY_32BIT_ANSI: 00528 _crc_table = (uint32_t *)Table_CRC_32bit_ANSI; 00529 break; 00530 case POLY_32BIT_REV_ANSI: 00531 _crc_table = (uint32_t *)Table_CRC_32bit_Rev_ANSI; 00532 break; 00533 case POLY_8BIT_CCITT: 00534 _crc_table = (uint32_t *)Table_CRC_8bit_CCITT; 00535 break; 00536 case POLY_7BIT_SD: 00537 _crc_table = (uint32_t *)Table_CRC_7Bit_SD; 00538 break; 00539 case POLY_16BIT_CCITT: 00540 _crc_table = (uint32_t *)Table_CRC_16bit_CCITT; 00541 break; 00542 case POLY_16BIT_IBM: 00543 _crc_table = (uint32_t *)Table_CRC_16bit_IBM; 00544 break; 00545 default: 00546 _crc_table = NULL; 00547 break; 00548 } 00549 _mode = (_crc_table != NULL) ? TABLE : BITWISE; 00550 } 00551 #endif 00552 }; 00553 00554 #if defined ( __CC_ARM ) 00555 #elif defined ( __GNUC__ ) 00556 #pragma GCC diagnostic pop 00557 #elif defined (__ICCARM__) 00558 #endif 00559 00560 /** @}*/ 00561 } // namespace mbed 00562 00563 #endif
Generated on Tue Jul 12 2022 20:41:15 by
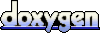