mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
InterruptIn.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef MBED_INTERRUPTIN_H 00018 #define MBED_INTERRUPTIN_H 00019 00020 #include "platform/platform.h" 00021 00022 #if DEVICE_INTERRUPTIN || defined(DOXYGEN_ONLY) 00023 00024 #include "hal/gpio_api.h" 00025 #include "hal/gpio_irq_api.h" 00026 #include "platform/Callback.h" 00027 #include "platform/mbed_critical.h" 00028 #include "platform/mbed_toolchain.h" 00029 #include "platform/NonCopyable.h" 00030 00031 namespace mbed { 00032 /** \addtogroup drivers */ 00033 00034 /** A digital interrupt input, used to call a function on a rising or falling edge 00035 * 00036 * @note Synchronization level: Interrupt safe 00037 * 00038 * Example: 00039 * @code 00040 * // Flash an LED while waiting for events 00041 * 00042 * #include "mbed.h" 00043 * 00044 * InterruptIn event(p16); 00045 * DigitalOut led(LED1); 00046 * 00047 * void trigger() { 00048 * printf("triggered!\n"); 00049 * } 00050 * 00051 * int main() { 00052 * // register trigger() to be called upon the rising edge of event 00053 * event.rise(&trigger); 00054 * while(1) { 00055 * led = !led; 00056 * wait(0.25); 00057 * } 00058 * } 00059 * @endcode 00060 * @ingroup drivers 00061 */ 00062 class InterruptIn : private NonCopyable<InterruptIn> { 00063 00064 public: 00065 00066 /** Create an InterruptIn connected to the specified pin 00067 * 00068 * @param pin InterruptIn pin to connect to 00069 */ 00070 InterruptIn(PinName pin); 00071 00072 /** Create an InterruptIn connected to the specified pin, 00073 * and the pin configured to the specified mode. 00074 * 00075 * @param pin InterruptIn pin to connect to 00076 * @param mode Desired Pin mode configuration. 00077 * (Valid values could be PullNone, PullDown, PullUp and PullDefault. 00078 * See PinNames.h for your target for definitions) 00079 * 00080 */ 00081 InterruptIn(PinName pin, PinMode mode); 00082 00083 virtual ~InterruptIn(); 00084 00085 /** Read the input, represented as 0 or 1 (int) 00086 * 00087 * @returns 00088 * An integer representing the state of the input pin, 00089 * 0 for logical 0, 1 for logical 1 00090 */ 00091 int read(); 00092 00093 /** An operator shorthand for read() 00094 */ 00095 operator int(); 00096 00097 00098 /** Attach a function to call when a rising edge occurs on the input 00099 * 00100 * @param func A pointer to a void function, or 0 to set as none 00101 */ 00102 void rise(Callback<void()> func); 00103 00104 /** Attach a member function to call when a rising edge occurs on the input 00105 * 00106 * @param obj pointer to the object to call the member function on 00107 * @param method pointer to the member function to be called 00108 * @deprecated 00109 * The rise function does not support cv-qualifiers. Replaced by 00110 * rise(callback(obj, method)). 00111 */ 00112 template<typename T, typename M> 00113 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00114 "The rise function does not support cv-qualifiers. Replaced by " 00115 "rise(callback(obj, method)).") 00116 void rise(T *obj, M method) 00117 { 00118 core_util_critical_section_enter(); 00119 rise(callback(obj, method)); 00120 core_util_critical_section_exit(); 00121 } 00122 00123 /** Attach a function to call when a falling edge occurs on the input 00124 * 00125 * @param func A pointer to a void function, or 0 to set as none 00126 */ 00127 void fall(Callback<void()> func); 00128 00129 /** Attach a member function to call when a falling edge occurs on the input 00130 * 00131 * @param obj pointer to the object to call the member function on 00132 * @param method pointer to the member function to be called 00133 * @deprecated 00134 * The rise function does not support cv-qualifiers. Replaced by 00135 * rise(callback(obj, method)). 00136 */ 00137 template<typename T, typename M> 00138 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00139 "The fall function does not support cv-qualifiers. Replaced by " 00140 "fall(callback(obj, method)).") 00141 void fall(T *obj, M method) 00142 { 00143 core_util_critical_section_enter(); 00144 fall(callback(obj, method)); 00145 core_util_critical_section_exit(); 00146 } 00147 00148 /** Set the input pin mode 00149 * 00150 * @param pull PullUp, PullDown, PullNone, PullDefault 00151 * See PinNames.h for your target for definitions) 00152 */ 00153 void mode(PinMode pull); 00154 00155 /** Enable IRQ. This method depends on hardware implementation, might enable one 00156 * port interrupts. For further information, check gpio_irq_enable(). 00157 */ 00158 void enable_irq(); 00159 00160 /** Disable IRQ. This method depends on hardware implementation, might disable one 00161 * port interrupts. For further information, check gpio_irq_disable(). 00162 */ 00163 void disable_irq(); 00164 00165 static void _irq_handler(uint32_t id, gpio_irq_event event); 00166 #if !defined(DOXYGEN_ONLY) 00167 protected: 00168 gpio_t gpio; 00169 gpio_irq_t gpio_irq; 00170 00171 Callback<void()> _rise; 00172 Callback<void()> _fall; 00173 00174 void irq_init(PinName pin); 00175 #endif 00176 }; 00177 00178 } // namespace mbed 00179 00180 #endif 00181 00182 #endif
Generated on Tue Jul 12 2022 20:41:15 by
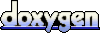