mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
FlashIAP.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef MBED_FLASHIAP_H 00023 #define MBED_FLASHIAP_H 00024 00025 #if DEVICE_FLASH || defined(DOXYGEN_ONLY) 00026 00027 #include "flash_api.h" 00028 #include "platform/SingletonPtr.h" 00029 #include "platform/PlatformMutex.h" 00030 #include "platform/NonCopyable.h" 00031 #include <algorithm> 00032 00033 // Export ROM end address 00034 #if defined(TOOLCHAIN_GCC_ARM) 00035 extern uint32_t __etext; 00036 extern uint32_t __data_start__; 00037 extern uint32_t __data_end__; 00038 #define FLASHIAP_APP_ROM_END_ADDR (((uint32_t) &__etext) + ((uint32_t) &__data_end__) - ((uint32_t) &__data_start__)) 00039 #elif defined(TOOLCHAIN_ARM) 00040 extern uint32_t Load$$LR$$LR_IROM1$$Limit[]; 00041 #define FLASHIAP_APP_ROM_END_ADDR ((uint32_t)Load$$LR$$LR_IROM1$$Limit) 00042 #elif defined(TOOLCHAIN_IAR) 00043 #pragma section=".rodata" 00044 #pragma section=".text" 00045 #pragma section=".init_array" 00046 #define FLASHIAP_APP_ROM_END_ADDR std::max(std::max((uint32_t) __section_end(".rodata"), (uint32_t) __section_end(".text")), \ 00047 (uint32_t) __section_end(".init_array")) 00048 #endif 00049 00050 namespace mbed { 00051 00052 /** \addtogroup drivers */ 00053 00054 /** Flash IAP driver. It invokes flash HAL functions. 00055 * 00056 * @note Synchronization level: Thread safe 00057 * @ingroup drivers 00058 */ 00059 class FlashIAP : private NonCopyable<FlashIAP> { 00060 public: 00061 FlashIAP(); 00062 ~FlashIAP(); 00063 00064 /** Initialize a flash IAP device 00065 * 00066 * Should be called once per lifetime of the object. 00067 * @return 0 on success or a negative error code on failure 00068 */ 00069 int init(); 00070 00071 /** Deinitialize a flash IAP device 00072 * 00073 * @return 0 on success or a negative error code on failure 00074 */ 00075 int deinit(); 00076 00077 /** Read data from a flash device. 00078 * 00079 * This method invokes memcpy - reads number of bytes from the address 00080 * 00081 * @param buffer Buffer to write to 00082 * @param addr Flash address to begin reading from 00083 * @param size Size to read in bytes 00084 * @return 0 on success, negative error code on failure 00085 */ 00086 int read(void *buffer, uint32_t addr, uint32_t size); 00087 00088 /** Program data to pages 00089 * 00090 * The sectors must have been erased prior to being programmed 00091 * 00092 * @param buffer Buffer of data to be written 00093 * @param addr Address of a page to begin writing to 00094 * @param size Size to write in bytes, must be a multiple of program size 00095 * @return 0 on success, negative error code on failure 00096 */ 00097 int program(const void *buffer, uint32_t addr, uint32_t size); 00098 00099 /** Erase sectors 00100 * 00101 * The state of an erased sector is undefined until it has been programmed 00102 * 00103 * @param addr Address of a sector to begin erasing, must be a multiple of the sector size 00104 * @param size Size to erase in bytes, must be a multiple of the sector size 00105 * @return 0 on success, negative error code on failure 00106 */ 00107 int erase(uint32_t addr, uint32_t size); 00108 00109 /** Get the sector size at the defined address 00110 * 00111 * Sector size might differ at address ranges. 00112 * An example <0-0x1000, sector size=1024; 0x10000-0x20000, size=2048> 00113 * 00114 * @param addr Address of or inside the sector to query 00115 * @return Size of a sector in bytes or MBED_FLASH_INVALID_SIZE if not mapped 00116 */ 00117 uint32_t get_sector_size(uint32_t addr) const; 00118 00119 /** Get the flash start address 00120 * 00121 * @return Flash start address 00122 */ 00123 uint32_t get_flash_start() const; 00124 00125 /** Get the flash size 00126 * 00127 * @return Flash size 00128 */ 00129 uint32_t get_flash_size() const; 00130 00131 /** Get the program page size 00132 * 00133 * The page size defines the writable page size 00134 * @return Size of a program page in bytes 00135 */ 00136 uint32_t get_page_size() const; 00137 00138 /** Get the flash erase value 00139 * 00140 * Get the value we read after erase operation 00141 * @return flash erase value 00142 */ 00143 uint8_t get_erase_value() const; 00144 00145 #if !defined(DOXYGEN_ONLY) 00146 private: 00147 00148 /* Check if address and size are aligned to a sector 00149 * 00150 * @param addr Address of block to check for alignment 00151 * @param size Size of block to check for alignment 00152 * @return true if the block is sector aligned, false otherwise 00153 */ 00154 bool is_aligned_to_sector(uint32_t addr, uint32_t size); 00155 00156 flash_t _flash; 00157 uint8_t *_page_buf; 00158 static SingletonPtr<PlatformMutex> _mutex; 00159 #endif 00160 }; 00161 00162 } /* namespace mbed */ 00163 00164 #endif /* DEVICE_FLASH */ 00165 00166 #endif /* MBED_FLASHIAP_H */
Generated on Tue Jul 12 2022 20:41:14 by
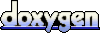