mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
mbed_assert.h
00001 00002 /** \addtogroup platform */ 00003 /** @{*/ 00004 /** 00005 * \defgroup platform_Assert Assert macros 00006 * @{ 00007 */ 00008 /* mbed Microcontroller Library 00009 * Copyright (c) 2006-2013 ARM Limited 00010 * SPDX-License-Identifier: Apache-2.0 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the "License"); 00013 * you may not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * http://www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an "AS IS" BASIS, 00020 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 #ifndef MBED_ASSERT_H 00025 #define MBED_ASSERT_H 00026 00027 #include "mbed_preprocessor.h" 00028 #include "mbed_toolchain.h" 00029 00030 #ifdef __cplusplus 00031 extern "C" { 00032 #endif 00033 00034 /** Internal mbed assert function which is invoked when MBED_ASSERT macro fails. 00035 * This function is active only if NDEBUG is not defined prior to including this 00036 * assert header file. 00037 * In case of MBED_ASSERT failing condition, error() is called with the assertation message. 00038 * @param expr Expression to be checked. 00039 * @param file File where assertation failed. 00040 * @param line Failing assertation line number. 00041 */ 00042 MBED_NORETURN void mbed_assert_internal(const char *expr, const char *file, int line); 00043 00044 #ifdef __cplusplus 00045 } 00046 #endif 00047 00048 /** MBED_ASSERT 00049 * Declare runtime assertions: results in runtime error if condition is false 00050 * 00051 * @note 00052 * Use of MBED_ASSERT is limited to Debug and Develop builds. 00053 * 00054 * @code 00055 * 00056 * int Configure(serial_t *obj) { 00057 * MBED_ASSERT(obj); 00058 * } 00059 * @endcode 00060 */ 00061 #ifdef NDEBUG 00062 #define MBED_ASSERT(expr) ((void)0) 00063 00064 #else 00065 #define MBED_ASSERT(expr) \ 00066 do { \ 00067 if (!(expr)) { \ 00068 mbed_assert_internal(#expr, __FILE__, __LINE__); \ 00069 } \ 00070 } while (0) 00071 #endif 00072 00073 00074 /** MBED_STATIC_ASSERT 00075 * Declare compile-time assertions, results in compile-time error if condition is false 00076 * 00077 * The assertion acts as a declaration that can be placed at file scope, in a 00078 * code block (except after a label), or as a member of a C++ class/struct/union. 00079 * 00080 * @note 00081 * Use of MBED_STATIC_ASSERT as a member of a struct/union is limited: 00082 * - In C++, MBED_STATIC_ASSERT is valid in class/struct/union scope. 00083 * - In C, MBED_STATIC_ASSERT is not valid in struct/union scope, and 00084 * MBED_STRUCT_STATIC_ASSERT is provided as an alternative that is valid 00085 * in C and C++ class/struct/union scope. 00086 * 00087 * @code 00088 * MBED_STATIC_ASSERT(MBED_LIBRARY_VERSION >= 120, 00089 * "The mbed library must be at least version 120"); 00090 * 00091 * int main() { 00092 * MBED_STATIC_ASSERT(sizeof(int) >= sizeof(char), 00093 * "An int must be larger than a char"); 00094 * } 00095 * @endcode 00096 */ 00097 #if defined(__cplusplus) && (__cplusplus >= 201103L || __cpp_static_assert >= 200410L) 00098 #define MBED_STATIC_ASSERT(expr, msg) static_assert(expr, msg) 00099 #elif !defined(__cplusplus) && __STDC_VERSION__ >= 201112L 00100 #define MBED_STATIC_ASSERT(expr, msg) _Static_assert(expr, msg) 00101 #elif defined(__cplusplus) && defined(__GNUC__) && defined(__GXX_EXPERIMENTAL_CXX0X__) \ 00102 && (__GNUC__*100 + __GNUC_MINOR__) > 403L 00103 #define MBED_STATIC_ASSERT(expr, msg) __extension__ static_assert(expr, msg) 00104 #elif !defined(__cplusplus) && defined(__GNUC__) && !defined(__CC_ARM) \ 00105 && (__GNUC__*100 + __GNUC_MINOR__) > 406L 00106 #define MBED_STATIC_ASSERT(expr, msg) __extension__ _Static_assert(expr, msg) 00107 #elif defined(__ICCARM__) 00108 #define MBED_STATIC_ASSERT(expr, msg) static_assert(expr, msg) 00109 #else 00110 #define MBED_STATIC_ASSERT(expr, msg) \ 00111 enum {MBED_CONCAT(MBED_ASSERTION_AT_, __LINE__) = sizeof(char[(expr) ? 1 : -1])} 00112 #endif 00113 00114 /** MBED_STRUCT_STATIC_ASSERT 00115 * Declare compile-time assertions, results in compile-time error if condition is false 00116 * 00117 * Unlike MBED_STATIC_ASSERT, MBED_STRUCT_STATIC_ASSERT can and must be used 00118 * as a member of a C/C++ class/struct/union. 00119 * 00120 * @code 00121 * struct thing { 00122 * MBED_STATIC_ASSERT(2 + 2 == 4, 00123 * "Hopefully the universe is mathematically consistent"); 00124 * }; 00125 * @endcode 00126 */ 00127 #define MBED_STRUCT_STATIC_ASSERT(expr, msg) int : (expr) ? 0 : -1 00128 00129 00130 #endif 00131 00132 /**@}*/ 00133 00134 /**@}*/ 00135
Generated on Tue Jul 12 2022 20:41:15 by
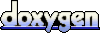