mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
itm_api.h
00001 /** \addtogroup hal */ 00002 /** @{*/ 00003 /* mbed Microcontroller Library 00004 * Copyright (c) 2017 ARM Limited 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 00020 #ifndef MBED_ITM_API_H 00021 #define MBED_ITM_API_H 00022 00023 #if DEVICE_ITM 00024 00025 #include <stdint.h> 00026 #include <stddef.h> 00027 00028 #ifdef __cplusplus 00029 extern "C" { 00030 #endif 00031 00032 /** 00033 * \defgroup itm_hal Instrumented Trace Macrocell HAL API 00034 * @{ 00035 */ 00036 00037 enum { 00038 ITM_PORT_SWO = 0 00039 }; 00040 00041 /** 00042 * @brief Target specific initialization function. 00043 * This function is responsible for initializing and configuring 00044 * the debug clock for the ITM and setting up the SWO pin for 00045 * debug output. 00046 * 00047 * The only Cortex-M register that should be modified is the clock 00048 * prescaler in TPI->ACPR. 00049 * 00050 * The generic mbed_itm_init initialization function will setup: 00051 * 00052 * ITM->LAR 00053 * ITM->TPR 00054 * ITM->TCR 00055 * ITM->TER 00056 * TPI->SPPR 00057 * TPI->FFCR 00058 * DWT->CTRL 00059 * 00060 * for SWO output on stimulus port 0. 00061 */ 00062 void itm_init(void); 00063 00064 /** 00065 * @brief Initialization function for both generic registers and target specific clock and pin. 00066 */ 00067 void mbed_itm_init(void); 00068 00069 /** 00070 * @brief Send data over ITM stimulus port. 00071 * 00072 * @param[in] port The stimulus port to send data over. 00073 * @param[in] data The 32-bit data to send. 00074 * 00075 * The data is written as a single 32-bit write to the port. 00076 * 00077 * @return value of data sent. 00078 */ 00079 uint32_t mbed_itm_send(uint32_t port, uint32_t data); 00080 00081 /** 00082 * @brief Send a block of data over ITM stimulus port. 00083 * 00084 * @param[in] port The stimulus port to send data over. 00085 * @param[in] data The block of data to send. 00086 * @param[in] len The number of bytes of data to send. 00087 * 00088 * The data is written using multiple appropriately-sized port accesses for 00089 * efficient transfer. 00090 */ 00091 void mbed_itm_send_block(uint32_t port, const void *data, size_t len); 00092 00093 /**@}*/ 00094 00095 #ifdef __cplusplus 00096 } 00097 #endif 00098 00099 #endif 00100 00101 #endif /* MBED_ITM_API_H */ 00102 00103 /**@}*/
Generated on Tue Jul 12 2022 20:41:15 by
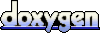