mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
crc_api.h
00001 /** \addtogroup hal */ 00002 /** @{*/ 00003 /* mbed Microcontroller Library 00004 * Copyright (c) 2018 ARM Limited 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 #ifndef MBED_CRC_HAL_API_H 00020 #define MBED_CRC_HAL_API_H 00021 00022 #include <stdbool.h> 00023 #include <stddef.h> 00024 #include <stdint.h> 00025 00026 /** CRC Polynomial value 00027 * 00028 * Different polynomial values supported 00029 */ 00030 typedef enum crc_polynomial { 00031 POLY_OTHER = 0, 00032 POLY_8BIT_CCITT = 0x07, // x8+x2+x+1 00033 POLY_7BIT_SD = 0x9, // x7+x3+1; 00034 POLY_16BIT_CCITT = 0x1021, // x16+x12+x5+1 00035 POLY_16BIT_IBM = 0x8005, // x16+x15+x2+1 00036 POLY_32BIT_ANSI = 0x04C11DB7, // x32+x26+x23+x22+x16+x12+x11+x10+x8+x7+x5+x4+x2+x+1 00037 POLY_32BIT_REV_ANSI = 0xEDB88320 00038 } crc_polynomial_t; 00039 00040 typedef struct crc_mbed_config { 00041 /** CRC Polynomial. Example polynomial: 0x21 = 0010_0011 = x^5+x+1 */ 00042 uint32_t polynomial; 00043 /** CRC Bit Width */ 00044 uint32_t width; 00045 /** Initial seed value for the computation. */ 00046 uint32_t initial_xor; 00047 /** Final xor value for the computation. */ 00048 uint32_t final_xor; 00049 /** Reflect bits on input. */ 00050 bool reflect_in; 00051 /** Reflect bits in final result before returning. */ 00052 bool reflect_out; 00053 } crc_mbed_config_t; 00054 00055 #if DEVICE_CRC 00056 00057 #ifdef __cplusplus 00058 extern "C" { 00059 #endif 00060 00061 /** 00062 * \defgroup hal_crc Hardware CRC 00063 * 00064 * The Hardware CRC HAL API provides a low-level interface to the Hardware CRC 00065 * module of a target platform. 00066 * 00067 * # Defined behaviour 00068 * 00069 * * Function hal_crc_is_supported() returns true if platform supports hardware 00070 * CRC for the given polynomial/width - verified by test ::crc_is_supported_test. 00071 * * Function hal_crc_is_supported() returns false if platform does not support hardware 00072 * CRC for the given polynomial/width - verified by test ::crc_is_supported_test. 00073 * * Function hal_crc_is_supported() returns false if given pointer to configuration 00074 * structure is undefined (NULL) - verified by test ::crc_is_supported_invalid_param_test. 00075 * * If CRC module does not support one of the following settings: initial_xor, final_xor 00076 * reflect_in, reflect_out, then these operations should be handled by the driver 00077 * - Verified by test ::crc_calc_single_test. 00078 * * Platform which supports hardware CRC must be able to handle at least one of the predefined 00079 * polynomial/width configurations that can be constructed in the MbedCRC class: POLY_8BIT_CCITT, 00080 * POLY_7BIT_SD, POLY_16BIT_CCITT, POLY_16BIT_IBM, POLY_32BIT_ANSI 00081 * - verified by test ::crc_is_supported_test, ::crc_calc_single_test. 00082 * * Function hal_crc_compute_partial_start() configures CRC module with the given configuration 00083 * - Verified by test ::crc_calc_single_test. 00084 * * Calling hal_crc_compute_partial_start() without finalising the 00085 * CRC calculation overrides the current configuration - Verified by test ::crc_reconfigure_test. 00086 * * Function hal_crc_compute_partial() writes data to the CRC module - verified by test ::crc_calc_single_test. 00087 * * Function hal_crc_compute_partial() can be call multiple times in succession in order to 00088 * provide additional data to CRC module - verified by test ::crc_calc_multi_test. 00089 * * Function hal_crc_compute_partial() does nothing if pointer to buffer is undefined or 00090 * data length is equal to 0 - verified by test ::crc_compute_partial_invalid_param_test. 00091 * * Function hal_crc_get_result() returns the checksum result from the CRC module 00092 * - verified by tests ::crc_calc_single_test, ::crc_calc_multi_test, ::crc_reconfigure_test. 00093 * 00094 * # Undefined behaviour 00095 * 00096 * * Calling hal_crc_compute_partial_start() function with invalid (unsupported) polynomial. 00097 * * Calling hal_crc_compute_partial() or hal_crc_get_result() functions before hal_crc_compute_partial_start(). 00098 * * Calling hal_crc_get_result() function multiple times. 00099 * 00100 * # Non-functional requirements 00101 * 00102 * * CRC configuration provides the following settings: 00103 * * polynomial - CRC Polynomial, 00104 * * width - CRC bit width, 00105 * * initial_xor - seed value for the computation, 00106 * * final_xor - final xor value for the computation, 00107 * * reflect_in - reflect bits on input, 00108 * * reflect_out - reflect bits in final result before returning. 00109 * 00110 * # Potential bugs 00111 * 00112 * @{ 00113 */ 00114 00115 /** 00116 * \defgroup hal_crc_tests crc hal tests 00117 * The crc HAL tests ensure driver conformance to defined behaviour. 00118 * 00119 * To run the crc hal tests use the command: 00120 * 00121 * mbed test -t <toolchain> -m <target> -n tests-mbed_hal-crc* 00122 * 00123 */ 00124 00125 /** Determine if the current platform supports hardware CRC for given polynomial 00126 * 00127 * The purpose of this function is to inform the CRC Platform API whether the 00128 * current platform has a hardware CRC module and that it can support the 00129 * requested polynomial. 00130 * 00131 * Supported polynomials are restricted to the named polynomials that can be 00132 * constructed in the MbedCRC class, POLY_8BIT_CCITT, POLY_7BIT_SD, 00133 * POLY_16BIT_CCITT, POLY_16BIT_IBM and POLY_32BIT_ANSI. 00134 * 00135 * The current platform must support the given polynomials default parameters 00136 * in order to return a true response. These include: reflect in, reflect out, 00137 * initial xor and final xor. For example, POLY_32BIT_ANSI requires an initial 00138 * and final xor of 0xFFFFFFFF, and reflection of both input and output. If any 00139 * of these settings cannot be configured, the polynomial is not supported. 00140 * 00141 * This function is thread safe; it safe to call from multiple contexts if 00142 * required. 00143 * 00144 * \param config Contains CRC configuration parameters for initializing the 00145 * hardware CRC module. For example, polynomial and initial seed 00146 * values. 00147 * 00148 * \return True if running if the polynomial is supported, false if not. 00149 */ 00150 bool hal_crc_is_supported(const crc_mbed_config_t *config); 00151 00152 /** Initialize the hardware CRC module with the given polynomial 00153 * 00154 * After calling this function, the CRC HAL module is ready to receive data 00155 * using the hal_crc_compute_partial() function. The CRC module on the board 00156 * is configured internally with the specified configuration and is ready 00157 * to receive data. 00158 * 00159 * The platform configures itself based on the default configuration 00160 * parameters of the input polynomial. 00161 * 00162 * This function must be called before calling hal_crc_compute_partial(). 00163 * 00164 * This function must be called with a valid polynomial supported by the 00165 * platform. The polynomial must be checked for support using the 00166 * hal_crc_is_supported() function. 00167 * 00168 * Calling hal_crc_compute_partial_start() multiple times without finalizing the 00169 * CRC calculation with hal_crc_get_result() overrides the current 00170 * configuration and state, and the intermediate result of the computation is 00171 * lost. 00172 * 00173 * This function is not thread safe. A CRC calculation must not be started from 00174 * two different threads or contexts at the same time; calling this function 00175 * from two different contexts may lead to configurations being overwritten and 00176 * results being lost. 00177 * 00178 * \param config Contains CRC configuration parameters for initializing the 00179 * hardware CRC module. For example, polynomial and initial seed 00180 * values. 00181 */ 00182 void hal_crc_compute_partial_start(const crc_mbed_config_t *config); 00183 00184 /** Writes data to the current CRC module. 00185 * 00186 * Writes input data buffer bytes to the CRC data register. The CRC module 00187 * must interpret the data as an array of bytes. 00188 * 00189 * The final transformations are not applied to the data; the CRC module must 00190 * retain the intermediate result so that additional calls to this function 00191 * can be made, appending the additional data to the calculation. 00192 * 00193 * To obtain the final result of the CRC calculation, hal_crc_get_result() is 00194 * called to apply the final transformations to the data. 00195 * 00196 * If the function is passed an undefined pointer, or the size of the buffer is 00197 * specified to be 0, this function does nothing and returns. 00198 * 00199 * This function can be called multiple times in succession. This can be used 00200 * to calculate the CRC result of streamed data. 00201 * 00202 * This function is not thread safe. There is only one instance of the CRC 00203 * module active at a time. Calling this function from multiple contexts 00204 * appends different data to the same, single instance of the module, which causes an 00205 * erroneous value to be calculated. 00206 * 00207 * \param data Input data stream to be written into the CRC calculation 00208 * \param size Size of the data stream in bytes 00209 */ 00210 void hal_crc_compute_partial(const uint8_t *data, const size_t size); 00211 00212 /* Reads the checksum result from the CRC module. 00213 * 00214 * Reads the final checksum result for the final checksum value. The returned 00215 * value is cast as an unsigned 32-bit integer. The actual size of the returned 00216 * result depends on the polynomial used to configure the CRC module. 00217 * 00218 * Additional transformations that are used in the default configuration of the 00219 * input polynomial are applied to the result before it is returned from this 00220 * function. These transformations include: the final xor being appended to the 00221 * calculation, and the result being reflected if required. 00222 * 00223 * Calling this function multiple times is undefined. The first call to this 00224 * function returns the final result of the CRC calculation. The return 00225 * value on successive calls is undefined because the contents of the register after 00226 * accessing them is platform-specific. 00227 * 00228 * This function is not thread safe. There is only one instance of the CRC 00229 * module active at a time. Calling this function from multiple contexts may 00230 * return incorrect data or affect the current state of the module. 00231 * 00232 * \return The final CRC checksum after the reflections and final calculations 00233 * have been applied. 00234 */ 00235 uint32_t hal_crc_get_result(void); 00236 00237 /**@}*/ 00238 00239 #ifdef __cplusplus 00240 }; 00241 #endif 00242 00243 #endif // DEVICE_CRC 00244 #endif // MBED_CRC_HAL_API_H 00245 00246 /**@}*/
Generated on Tue Jul 12 2022 20:41:14 by
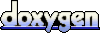