mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
Timer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef MBED_TIMER_H 00018 #define MBED_TIMER_H 00019 00020 #include "platform/platform.h" 00021 #include "hal/ticker_api.h" 00022 #include "platform/NonCopyable.h" 00023 #include "platform/mbed_power_mgmt.h" 00024 00025 namespace mbed { 00026 /** \addtogroup drivers */ 00027 00028 /** A general purpose timer 00029 * 00030 * @note Synchronization level: Interrupt safe 00031 * 00032 * Example: 00033 * @code 00034 * // Count the time to toggle an LED 00035 * 00036 * #include "mbed.h" 00037 * 00038 * Timer timer; 00039 * DigitalOut led(LED1); 00040 * int begin, end; 00041 * 00042 * int main() { 00043 * timer.start(); 00044 * begin = timer.read_us(); 00045 * led = !led; 00046 * end = timer.read_us(); 00047 * printf("Toggle the led takes %d us", end - begin); 00048 * } 00049 * @endcode 00050 * @ingroup drivers 00051 */ 00052 class Timer : private NonCopyable<Timer> { 00053 00054 public: 00055 Timer(); 00056 Timer(const ticker_data_t *data); 00057 ~Timer(); 00058 00059 /** Start the timer 00060 */ 00061 void start(); 00062 00063 /** Stop the timer 00064 */ 00065 void stop(); 00066 00067 /** Reset the timer to 0. 00068 * 00069 * If it was already running, it will continue 00070 */ 00071 void reset(); 00072 00073 /** Get the time passed in seconds 00074 * 00075 * @returns Time passed in seconds 00076 */ 00077 float read(); 00078 00079 /** Get the time passed in milliseconds 00080 * 00081 * @returns Time passed in milliseconds 00082 */ 00083 int read_ms(); 00084 00085 /** Get the time passed in microseconds 00086 * 00087 * @returns Time passed in microseconds 00088 */ 00089 int read_us(); 00090 00091 /** An operator shorthand for read() 00092 */ 00093 operator float(); 00094 00095 /** Get in a high resolution type the time passed in microseconds. 00096 * Returns a 64 bit integer. 00097 */ 00098 us_timestamp_t read_high_resolution_us(); 00099 00100 #if !defined(DOXYGEN_ONLY) 00101 protected: 00102 us_timestamp_t slicetime(); 00103 int _running; // whether the timer is running 00104 us_timestamp_t _start; // the start time of the latest slice 00105 us_timestamp_t _time; // any accumulated time from previous slices 00106 const ticker_data_t *_ticker_data; 00107 bool _lock_deepsleep; // flag that indicates if deep sleep should be disabled 00108 }; 00109 #endif 00110 00111 } // namespace mbed 00112 00113 #endif
Generated on Tue Jul 12 2022 20:41:16 by
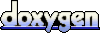