mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
Ethernet.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "drivers/Ethernet.h" 00018 00019 #if DEVICE_ETHERNET 00020 00021 #include "hal/ethernet_api.h" 00022 00023 namespace mbed { 00024 00025 Ethernet::Ethernet() 00026 { 00027 ethernet_init(); 00028 } 00029 00030 Ethernet::~Ethernet() 00031 { 00032 ethernet_free(); 00033 } 00034 00035 int Ethernet::write(const char *data, int size) 00036 { 00037 return ethernet_write(data, size); 00038 } 00039 00040 int Ethernet::send() 00041 { 00042 return ethernet_send(); 00043 } 00044 00045 int Ethernet::receive() 00046 { 00047 return ethernet_receive(); 00048 } 00049 00050 int Ethernet::read(char *data, int size) 00051 { 00052 return ethernet_read(data, size); 00053 } 00054 00055 void Ethernet::address(char *mac) 00056 { 00057 return ethernet_address(mac); 00058 } 00059 00060 int Ethernet::link() 00061 { 00062 return ethernet_link(); 00063 } 00064 00065 void Ethernet::set_link(Mode mode) 00066 { 00067 int speed = -1; 00068 int duplex = 0; 00069 00070 switch (mode) { 00071 case AutoNegotiate : 00072 speed = -1; 00073 duplex = 0; 00074 break; 00075 case HalfDuplex10 : 00076 speed = 0; 00077 duplex = 0; 00078 break; 00079 case FullDuplex10 : 00080 speed = 0; 00081 duplex = 1; 00082 break; 00083 case HalfDuplex100 : 00084 speed = 1; 00085 duplex = 0; 00086 break; 00087 case FullDuplex100 : 00088 speed = 1; 00089 duplex = 1; 00090 break; 00091 } 00092 00093 ethernet_set_link(speed, duplex); 00094 } 00095 00096 } // namespace mbed 00097 00098 #endif
Generated on Tue Jul 12 2022 20:41:14 by
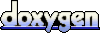