
WebSocket Hello World using the wifly interface
Dependencies: WebSocketClient WiflyInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "Websocket.h" 00004 00005 Ticker flash; 00006 DigitalOut led(LED1); 00007 void flashLED(void){led = !led;} 00008 00009 /* wifly interface: 00010 * - p9 and p10 are for the serial communication 00011 * - p19 is for the reset pin 00012 * - p26 is for the connection status 00013 * - "mbed" is the ssid of the network 00014 * - "password" is the password 00015 * - WPA is the security 00016 */ 00017 WiflyInterface wifly(p9, p10, p30, p29, "mbed", "password", WPA); 00018 00019 int main() 00020 { 00021 flash.attach(&flashLED, 1.0f); 00022 00023 wifly.init(); //Use DHCP 00024 while(!wifly.connect()); 00025 printf("IP Address: %s\n", wifly.getIPAddress()); 00026 00027 // view @ http://sockets.mbed.org/demo/viewer 00028 Websocket ws("ws://sockets.mbed.org:443/ws/demo/rw"); 00029 while (!ws.connect()); 00030 char str[100]; 00031 00032 for(int i=0; i<0x7fffffff; ++i) { 00033 // string with a message 00034 sprintf(str, "%d WebSocket Hello World over Wifi", i); 00035 ws.send(str); 00036 00037 // clear the buffer and wait a sec... 00038 memset(str, 0, 100); 00039 wait(0.5f); 00040 00041 // websocket server should echo whatever we sent it 00042 if (ws.read(str)) { 00043 printf("rcv'd: %s\n", str); 00044 } 00045 } 00046 ws.close(); 00047 wifly.disconnect(); 00048 00049 while(true); 00050 }
Generated on Thu Jul 14 2022 16:58:36 by
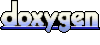