
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
xtea.h
00001 /** 00002 * \file xtea.h 00003 * 00004 * \brief XTEA block cipher (32-bit) 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_XTEA_H 00024 #define MBEDTLS_XTEA_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 #include <stdint.h> 00034 00035 #define MBEDTLS_XTEA_ENCRYPT 1 00036 #define MBEDTLS_XTEA_DECRYPT 0 00037 00038 #define MBEDTLS_ERR_XTEA_INVALID_INPUT_LENGTH -0x0028 /**< The data input has an invalid length. */ 00039 00040 #if !defined(MBEDTLS_XTEA_ALT) 00041 // Regular implementation 00042 // 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /** 00049 * \brief XTEA context structure 00050 */ 00051 typedef struct 00052 { 00053 uint32_t k[4]; /*!< key */ 00054 } 00055 mbedtls_xtea_context; 00056 00057 /** 00058 * \brief Initialize XTEA context 00059 * 00060 * \param ctx XTEA context to be initialized 00061 */ 00062 void mbedtls_xtea_init( mbedtls_xtea_context *ctx ); 00063 00064 /** 00065 * \brief Clear XTEA context 00066 * 00067 * \param ctx XTEA context to be cleared 00068 */ 00069 void mbedtls_xtea_free( mbedtls_xtea_context *ctx ); 00070 00071 /** 00072 * \brief XTEA key schedule 00073 * 00074 * \param ctx XTEA context to be initialized 00075 * \param key the secret key 00076 */ 00077 void mbedtls_xtea_setup( mbedtls_xtea_context *ctx, const unsigned char key[16] ); 00078 00079 /** 00080 * \brief XTEA cipher function 00081 * 00082 * \param ctx XTEA context 00083 * \param mode MBEDTLS_XTEA_ENCRYPT or MBEDTLS_XTEA_DECRYPT 00084 * \param input 8-byte input block 00085 * \param output 8-byte output block 00086 * 00087 * \return 0 if successful 00088 */ 00089 int mbedtls_xtea_crypt_ecb( mbedtls_xtea_context *ctx, 00090 int mode, 00091 const unsigned char input[8], 00092 unsigned char output[8] ); 00093 00094 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00095 /** 00096 * \brief XTEA CBC cipher function 00097 * 00098 * \param ctx XTEA context 00099 * \param mode MBEDTLS_XTEA_ENCRYPT or MBEDTLS_XTEA_DECRYPT 00100 * \param length the length of input, multiple of 8 00101 * \param iv initialization vector for CBC mode 00102 * \param input input block 00103 * \param output output block 00104 * 00105 * \return 0 if successful, 00106 * MBEDTLS_ERR_XTEA_INVALID_INPUT_LENGTH if the length % 8 != 0 00107 */ 00108 int mbedtls_xtea_crypt_cbc( mbedtls_xtea_context *ctx, 00109 int mode, 00110 size_t length, 00111 unsigned char iv[8], 00112 const unsigned char *input, 00113 unsigned char *output); 00114 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00115 00116 #ifdef __cplusplus 00117 } 00118 #endif 00119 00120 #else /* MBEDTLS_XTEA_ALT */ 00121 #include "xtea_alt.h" 00122 #endif /* MBEDTLS_XTEA_ALT */ 00123 00124 #ifdef __cplusplus 00125 extern "C" { 00126 #endif 00127 00128 /** 00129 * \brief Checkup routine 00130 * 00131 * \return 0 if successful, or 1 if the test failed 00132 */ 00133 int mbedtls_xtea_self_test( int verbose ); 00134 00135 #ifdef __cplusplus 00136 } 00137 #endif 00138 00139 #endif /* xtea.h */
Generated on Tue Jul 12 2022 18:27:27 by
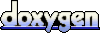