
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
threadwrapper.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_THREAD_WRAPPER_H__ 00017 #define M2M_THREAD_WRAPPER_H__ 00018 00019 #include <Thread.h> 00020 00021 namespace rtos { 00022 00023 // Wrapper for more complex function as thread targets 00024 template <typename T, void (*F)(T *)> 00025 static void __thread_pointer_wrapper(const void *data) 00026 { 00027 F((T*)data); 00028 } 00029 00030 template <typename T, void (*F)(T *)> 00031 static Thread *create_thread(T *arg=NULL, 00032 osPriority priority=osPriorityNormal, 00033 uint32_t stack_size=DEFAULT_STACK_SIZE, 00034 unsigned char *stack_pointer=NULL) 00035 { 00036 return new Thread(__thread_pointer_wrapper<F,T>, (void *)arg, 00037 priority, stack_size, stack_pointer); 00038 } 00039 00040 template <class T, void (T::*M)()> 00041 static void __thread_class_wrapper(const void *data) 00042 { 00043 (((T*)data)->*M)(); 00044 } 00045 00046 template <class T, void (T::*M)()> 00047 static Thread *create_thread(T *obj, 00048 osPriority priority=osPriorityNormal, 00049 uint32_t stack_size=DEFAULT_STACK_SIZE, 00050 unsigned char *stack_pointer=NULL) 00051 { 00052 return new Thread(__thread_class_wrapper<T,M>, (void *)obj, 00053 priority, stack_size, stack_pointer); 00054 } 00055 00056 } 00057 00058 00059 #endif //M2M_OBJECT_THREAD_H__
Generated on Tue Jul 12 2022 18:27:26 by
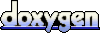