
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
ssl_cache.h
00001 /** 00002 * \file ssl_cache.h 00003 * 00004 * \brief SSL session cache implementation 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_SSL_CACHE_H 00024 #define MBEDTLS_SSL_CACHE_H 00025 00026 #include "ssl.h" 00027 00028 #if defined(MBEDTLS_THREADING_C) 00029 #include "threading.h" 00030 #endif 00031 00032 /** 00033 * \name SECTION: Module settings 00034 * 00035 * The configuration options you can set for this module are in this section. 00036 * Either change them in config.h or define them on the compiler command line. 00037 * \{ 00038 */ 00039 00040 #if !defined(MBEDTLS_SSL_CACHE_DEFAULT_TIMEOUT) 00041 #define MBEDTLS_SSL_CACHE_DEFAULT_TIMEOUT 86400 /*!< 1 day */ 00042 #endif 00043 00044 #if !defined(MBEDTLS_SSL_CACHE_DEFAULT_MAX_ENTRIES) 00045 #define MBEDTLS_SSL_CACHE_DEFAULT_MAX_ENTRIES 50 /*!< Maximum entries in cache */ 00046 #endif 00047 00048 /* \} name SECTION: Module settings */ 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif 00053 00054 typedef struct mbedtls_ssl_cache_context mbedtls_ssl_cache_context; 00055 typedef struct mbedtls_ssl_cache_entry mbedtls_ssl_cache_entry; 00056 00057 /** 00058 * \brief This structure is used for storing cache entries 00059 */ 00060 struct mbedtls_ssl_cache_entry 00061 { 00062 #if defined(MBEDTLS_HAVE_TIME) 00063 time_t timestamp ; /*!< entry timestamp */ 00064 #endif 00065 mbedtls_ssl_session session ; /*!< entry session */ 00066 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00067 mbedtls_x509_buf peer_cert ; /*!< entry peer_cert */ 00068 #endif 00069 mbedtls_ssl_cache_entry *next ; /*!< chain pointer */ 00070 }; 00071 00072 /** 00073 * \brief Cache context 00074 */ 00075 struct mbedtls_ssl_cache_context 00076 { 00077 mbedtls_ssl_cache_entry *chain ; /*!< start of the chain */ 00078 int timeout ; /*!< cache entry timeout */ 00079 int max_entries ; /*!< maximum entries */ 00080 #if defined(MBEDTLS_THREADING_C) 00081 mbedtls_threading_mutex_t mutex ; /*!< mutex */ 00082 #endif 00083 }; 00084 00085 /** 00086 * \brief Initialize an SSL cache context 00087 * 00088 * \param cache SSL cache context 00089 */ 00090 void mbedtls_ssl_cache_init( mbedtls_ssl_cache_context *cache ); 00091 00092 /** 00093 * \brief Cache get callback implementation 00094 * (Thread-safe if MBEDTLS_THREADING_C is enabled) 00095 * 00096 * \param data SSL cache context 00097 * \param session session to retrieve entry for 00098 */ 00099 int mbedtls_ssl_cache_get( void *data, mbedtls_ssl_session *session ); 00100 00101 /** 00102 * \brief Cache set callback implementation 00103 * (Thread-safe if MBEDTLS_THREADING_C is enabled) 00104 * 00105 * \param data SSL cache context 00106 * \param session session to store entry for 00107 */ 00108 int mbedtls_ssl_cache_set( void *data, const mbedtls_ssl_session *session ); 00109 00110 #if defined(MBEDTLS_HAVE_TIME) 00111 /** 00112 * \brief Set the cache timeout 00113 * (Default: MBEDTLS_SSL_CACHE_DEFAULT_TIMEOUT (1 day)) 00114 * 00115 * A timeout of 0 indicates no timeout. 00116 * 00117 * \param cache SSL cache context 00118 * \param timeout cache entry timeout in seconds 00119 */ 00120 void mbedtls_ssl_cache_set_timeout( mbedtls_ssl_cache_context *cache, int timeout ); 00121 #endif /* MBEDTLS_HAVE_TIME */ 00122 00123 /** 00124 * \brief Set the maximum number of cache entries 00125 * (Default: MBEDTLS_SSL_CACHE_DEFAULT_MAX_ENTRIES (50)) 00126 * 00127 * \param cache SSL cache context 00128 * \param max cache entry maximum 00129 */ 00130 void mbedtls_ssl_cache_set_max_entries( mbedtls_ssl_cache_context *cache, int max ); 00131 00132 /** 00133 * \brief Free referenced items in a cache context and clear memory 00134 * 00135 * \param cache SSL cache context 00136 */ 00137 void mbedtls_ssl_cache_free( mbedtls_ssl_cache_context *cache ); 00138 00139 #ifdef __cplusplus 00140 } 00141 #endif 00142 00143 #endif /* ssl_cache.h */
Generated on Tue Jul 12 2022 18:27:25 by
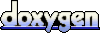