
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
platform.h
00001 /** 00002 * \file platform.h 00003 * 00004 * \brief mbed TLS Platform abstraction layer 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_PLATFORM_H 00024 #define MBEDTLS_PLATFORM_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 /** 00037 * \name SECTION: Module settings 00038 * 00039 * The configuration options you can set for this module are in this section. 00040 * Either change them in config.h or define them on the compiler command line. 00041 * \{ 00042 */ 00043 00044 #if !defined(MBEDTLS_PLATFORM_NO_STD_FUNCTIONS) 00045 #include <stdio.h> 00046 #include <stdlib.h> 00047 #if !defined(MBEDTLS_PLATFORM_STD_SNPRINTF) 00048 #if defined(_WIN32) 00049 #define MBEDTLS_PLATFORM_STD_SNPRINTF mbedtls_platform_win32_snprintf /**< Default snprintf to use */ 00050 #else 00051 #define MBEDTLS_PLATFORM_STD_SNPRINTF snprintf /**< Default snprintf to use */ 00052 #endif 00053 #endif 00054 #if !defined(MBEDTLS_PLATFORM_STD_PRINTF) 00055 #define MBEDTLS_PLATFORM_STD_PRINTF printf /**< Default printf to use */ 00056 #endif 00057 #if !defined(MBEDTLS_PLATFORM_STD_FPRINTF) 00058 #define MBEDTLS_PLATFORM_STD_FPRINTF fprintf /**< Default fprintf to use */ 00059 #endif 00060 #if !defined(MBEDTLS_PLATFORM_STD_CALLOC) 00061 #define MBEDTLS_PLATFORM_STD_CALLOC calloc /**< Default allocator to use */ 00062 #endif 00063 #if !defined(MBEDTLS_PLATFORM_STD_FREE) 00064 #define MBEDTLS_PLATFORM_STD_FREE free /**< Default free to use */ 00065 #endif 00066 #if !defined(MBEDTLS_PLATFORM_STD_EXIT) 00067 #define MBEDTLS_PLATFORM_STD_EXIT exit /**< Default free to use */ 00068 #endif 00069 #else /* MBEDTLS_PLATFORM_NO_STD_FUNCTIONS */ 00070 #if defined(MBEDTLS_PLATFORM_STD_MEM_HDR) 00071 #include MBEDTLS_PLATFORM_STD_MEM_HDR 00072 #endif 00073 #endif /* MBEDTLS_PLATFORM_NO_STD_FUNCTIONS */ 00074 00075 /* \} name SECTION: Module settings */ 00076 00077 /* 00078 * The function pointers for calloc and free 00079 */ 00080 #if defined(MBEDTLS_PLATFORM_MEMORY) 00081 #if defined(MBEDTLS_PLATFORM_FREE_MACRO) && \ 00082 defined(MBEDTLS_PLATFORM_CALLOC_MACRO) 00083 #define mbedtls_free MBEDTLS_PLATFORM_FREE_MACRO 00084 #define mbedtls_calloc MBEDTLS_PLATFORM_CALLOC_MACRO 00085 #else 00086 /* For size_t */ 00087 #include <stddef.h> 00088 extern void * (*mbedtls_calloc)( size_t n, size_t size ); 00089 extern void (*mbedtls_free)( void *ptr ); 00090 00091 /** 00092 * \brief Set your own memory implementation function pointers 00093 * 00094 * \param calloc_func the calloc function implementation 00095 * \param free_func the free function implementation 00096 * 00097 * \return 0 if successful 00098 */ 00099 int mbedtls_platform_set_calloc_free( void * (*calloc_func)( size_t, size_t ), 00100 void (*free_func)( void * ) ); 00101 #endif /* MBEDTLS_PLATFORM_FREE_MACRO && MBEDTLS_PLATFORM_CALLOC_MACRO */ 00102 #else /* !MBEDTLS_PLATFORM_MEMORY */ 00103 #define mbedtls_free free 00104 #define mbedtls_calloc calloc 00105 #endif /* MBEDTLS_PLATFORM_MEMORY && !MBEDTLS_PLATFORM_{FREE,CALLOC}_MACRO */ 00106 00107 /* 00108 * The function pointers for fprintf 00109 */ 00110 #if defined(MBEDTLS_PLATFORM_FPRINTF_ALT) 00111 /* We need FILE * */ 00112 #include <stdio.h> 00113 extern int (*mbedtls_fprintf)( FILE *stream, const char *format, ... ); 00114 00115 /** 00116 * \brief Set your own fprintf function pointer 00117 * 00118 * \param fprintf_func the fprintf function implementation 00119 * 00120 * \return 0 00121 */ 00122 int mbedtls_platform_set_fprintf( int (*fprintf_func)( FILE *stream, const char *, 00123 ... ) ); 00124 #else 00125 #if defined(MBEDTLS_PLATFORM_FPRINTF_MACRO) 00126 #define mbedtls_fprintf MBEDTLS_PLATFORM_FPRINTF_MACRO 00127 #else 00128 #define mbedtls_fprintf fprintf 00129 #endif /* MBEDTLS_PLATFORM_FPRINTF_MACRO */ 00130 #endif /* MBEDTLS_PLATFORM_FPRINTF_ALT */ 00131 00132 /* 00133 * The function pointers for printf 00134 */ 00135 #if defined(MBEDTLS_PLATFORM_PRINTF_ALT) 00136 extern int (*mbedtls_printf)( const char *format, ... ); 00137 00138 /** 00139 * \brief Set your own printf function pointer 00140 * 00141 * \param printf_func the printf function implementation 00142 * 00143 * \return 0 00144 */ 00145 int mbedtls_platform_set_printf( int (*printf_func)( const char *, ... ) ); 00146 #else /* !MBEDTLS_PLATFORM_PRINTF_ALT */ 00147 #if defined(MBEDTLS_PLATFORM_PRINTF_MACRO) 00148 #define mbedtls_printf MBEDTLS_PLATFORM_PRINTF_MACRO 00149 #else 00150 #define mbedtls_printf printf 00151 #endif /* MBEDTLS_PLATFORM_PRINTF_MACRO */ 00152 #endif /* MBEDTLS_PLATFORM_PRINTF_ALT */ 00153 00154 /* 00155 * The function pointers for snprintf 00156 * 00157 * The snprintf implementation should conform to C99: 00158 * - it *must* always correctly zero-terminate the buffer 00159 * (except when n == 0, then it must leave the buffer untouched) 00160 * - however it is acceptable to return -1 instead of the required length when 00161 * the destination buffer is too short. 00162 */ 00163 #if defined(_WIN32) 00164 /* For Windows (inc. MSYS2), we provide our own fixed implementation */ 00165 int mbedtls_platform_win32_snprintf( char *s, size_t n, const char *fmt, ... ); 00166 #endif 00167 00168 #if defined(MBEDTLS_PLATFORM_SNPRINTF_ALT) 00169 extern int (*mbedtls_snprintf)( char * s, size_t n, const char * format, ... ); 00170 00171 /** 00172 * \brief Set your own snprintf function pointer 00173 * 00174 * \param snprintf_func the snprintf function implementation 00175 * 00176 * \return 0 00177 */ 00178 int mbedtls_platform_set_snprintf( int (*snprintf_func)( char * s, size_t n, 00179 const char * format, ... ) ); 00180 #else /* MBEDTLS_PLATFORM_SNPRINTF_ALT */ 00181 #if defined(MBEDTLS_PLATFORM_SNPRINTF_MACRO) 00182 #define mbedtls_snprintf MBEDTLS_PLATFORM_SNPRINTF_MACRO 00183 #else 00184 #define mbedtls_snprintf snprintf 00185 #endif /* MBEDTLS_PLATFORM_SNPRINTF_MACRO */ 00186 #endif /* MBEDTLS_PLATFORM_SNPRINTF_ALT */ 00187 00188 /* 00189 * The function pointers for exit 00190 */ 00191 #if defined(MBEDTLS_PLATFORM_EXIT_ALT) 00192 extern void (*mbedtls_exit)( int status ); 00193 00194 /** 00195 * \brief Set your own exit function pointer 00196 * 00197 * \param exit_func the exit function implementation 00198 * 00199 * \return 0 00200 */ 00201 int mbedtls_platform_set_exit( void (*exit_func)( int status ) ); 00202 #else 00203 #if defined(MBEDTLS_PLATFORM_EXIT_MACRO) 00204 #define mbedtls_exit MBEDTLS_PLATFORM_EXIT_MACRO 00205 #else 00206 #define mbedtls_exit exit 00207 #endif /* MBEDTLS_PLATFORM_EXIT_MACRO */ 00208 #endif /* MBEDTLS_PLATFORM_EXIT_ALT */ 00209 00210 #ifdef __cplusplus 00211 } 00212 #endif 00213 00214 #endif /* platform.h */
Generated on Tue Jul 12 2022 18:27:25 by
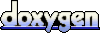