
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
m2mtimerpimpl.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed-client-classic/m2mtimerpimpl.h" 00017 #include "mbed-client/m2mtimerobserver.h" 00018 #include <cstdio> 00019 00020 M2MTimerPimpl::M2MTimerPimpl(M2MTimerObserver& observer) 00021 : _observer(observer), 00022 _single_shot(true), 00023 _interval(0), 00024 _type(M2MTimerObserver::Notdefined), 00025 _intermediate_interval(0), 00026 _total_interval(0), 00027 _status(0), 00028 _dtls_type(false), 00029 _thread(0) 00030 { 00031 00032 } 00033 00034 M2MTimerPimpl::~M2MTimerPimpl() 00035 { 00036 stop_timer(); 00037 } 00038 00039 void M2MTimerPimpl::start_timer(uint64_t interval, 00040 M2MTimerObserver::Type type, 00041 bool single_shot) 00042 { 00043 _dtls_type = false; 00044 _intermediate_interval = 0; 00045 _total_interval = 0; 00046 _status = 0; 00047 _single_shot = single_shot; 00048 _interval = interval; 00049 _type = type; 00050 _running = true; 00051 00052 _thread = rtos::create_thread<M2MTimerPimpl, &M2MTimerPimpl::timer_run>(this); 00053 } 00054 00055 void M2MTimerPimpl::start_dtls_timer(uint64_t intermediate_interval, uint64_t total_interval, M2MTimerObserver::Type type) 00056 { 00057 _dtls_type = true; 00058 _intermediate_interval = intermediate_interval; 00059 _total_interval = total_interval; 00060 _status = 0; 00061 _type = type; 00062 _running = true; 00063 00064 _thread = rtos::create_thread<M2MTimerPimpl, &M2MTimerPimpl::timer_run>(this); 00065 } 00066 00067 void M2MTimerPimpl::stop_timer() 00068 { 00069 _running = false; 00070 00071 if (_thread) { 00072 delete _thread; 00073 _thread = NULL; 00074 } 00075 } 00076 00077 void M2MTimerPimpl::timer_run() 00078 { 00079 if (!_dtls_type) { 00080 while (_running) { 00081 _thread->wait(_interval); 00082 if (!_running) return; 00083 _observer.timer_expired(_type); 00084 if (_single_shot) return; 00085 } 00086 } else { 00087 _thread->wait(_intermediate_interval); 00088 if (!_running) return; 00089 _status++; 00090 _thread->wait(_total_interval - _intermediate_interval); 00091 if (!_running) return; 00092 _status++; 00093 _observer.timer_expired(_type); 00094 } 00095 } 00096 00097 bool M2MTimerPimpl::is_intermediate_interval_passed() 00098 { 00099 if (_status > 0) { 00100 return true; 00101 } 00102 return false; 00103 } 00104 00105 bool M2MTimerPimpl::is_total_interval_passed() 00106 { 00107 if (_status > 1) { 00108 return true; 00109 } 00110 return false; 00111 }
Generated on Tue Jul 12 2022 18:27:24 by
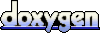