
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
m2mobjectinstance.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_OBJECT_INSTANCE_H 00017 #define M2M_OBJECT_INSTANCE_H 00018 00019 #include "mbed-client/m2mvector.h" 00020 #include "mbed-client/m2mresource.h" 00021 00022 //FORWARD DECLARATION 00023 typedef Vector<M2MResource *> M2MResourceList; 00024 typedef Vector<M2MResourceInstance *> M2MResourceInstanceList; 00025 00026 class M2MObjectCallback { 00027 public: 00028 virtual void notification_update() = 0; 00029 }; 00030 00031 /** 00032 * @brief M2MObjectInstance. 00033 * This class is the instance class for mbed Client Objects. All defined 00034 * LWM2M object models can be created based on it. This class also holds all resource 00035 * instances associated with the given object. 00036 */ 00037 00038 class M2MObjectInstance : public M2MBase, 00039 public M2MObjectInstanceCallback 00040 { 00041 00042 friend class M2MObject; 00043 00044 private: // Constructor and destructor are private which means 00045 // that these objects can be created or 00046 // deleted only through function provided by M2MObject. 00047 00048 /** 00049 * @brief Constructor 00050 * @param name, Name of the object 00051 */ 00052 M2MObjectInstance(const String &object_name, 00053 M2MObjectCallback &object_callback); 00054 00055 00056 // Prevents the use of default constructor. 00057 M2MObjectInstance(); 00058 00059 // Prevents the use of assignment operator. 00060 M2MObjectInstance& operator=( const M2MObjectInstance& /*other*/ ); 00061 00062 // Prevents the use of copy constructor. 00063 M2MObjectInstance( const M2MObjectInstance& /*other*/ ); 00064 00065 /** 00066 * Destructor 00067 */ 00068 virtual ~M2MObjectInstance(); 00069 00070 public: 00071 00072 /** 00073 * @brief Creates a static resource for a given mbed Client Inteface object. With this, the 00074 * client can respond to server's GET methods with the provided value. 00075 * @param resource_name, Name of the resource. 00076 * @param resource_type, Type of the resource. 00077 * @param value, Pointer to the value of the resource. 00078 * @param value_length, Length of the value in the pointer. 00079 * @param multiple_instance, Resource can have 00080 * multiple instances, default is false. 00081 * @return M2MResource, Resource for managing other client operations. 00082 */ 00083 M2MResource* create_static_resource(const String &resource_name, 00084 const String &resource_type, 00085 M2MResourceInstance::ResourceType type, 00086 const uint8_t *value, 00087 const uint8_t value_length, 00088 bool multiple_instance = false); 00089 00090 /** 00091 * @brief Creates a dynamic resource for a given mbed Client Inteface object. With this, 00092 * the client can respond to different queries from the server (GET,PUT etc). 00093 * This type of resource is also observable and carries callbacks. 00094 * @param resource_name, Name of the resource. 00095 * @param resource_type, Type of the resource. 00096 * @param observable, Indication, whether the resource is observable or not. 00097 * @param multiple_instance, Resource can have 00098 * multiple instances, default is false. 00099 * @return M2MResource, Resource for managing other client operations. 00100 */ 00101 M2MResource* create_dynamic_resource(const String &resource_name, 00102 const String &resource_type, 00103 M2MResourceInstance::ResourceType type, 00104 bool observable, 00105 bool multiple_instance = false); 00106 00107 00108 /** 00109 * @brief Creates a static resource instance for a given mbed Client Inteface object. With this, 00110 * the client can respond to server's GET methods with the provided value. 00111 * @param resource_name, Name of the resource. 00112 * @param resource_type, Type of the resource. 00113 * @param value, Pointer to the value of the resource. 00114 * @param value_length, Length of the value in pointer. 00115 * @param instance_id, Instance Id of the resource. 00116 * @return M2MResourceInstance, Resource Instance for managing other client operations. 00117 */ 00118 M2MResourceInstance* create_static_resource_instance(const String &resource_name, 00119 const String &resource_type, 00120 M2MResourceInstance::ResourceType type, 00121 const uint8_t *value, 00122 const uint8_t value_length, 00123 uint16_t instance_id); 00124 00125 /** 00126 * @brief Creates a dynamic resource instance for a given mbed Client Inteface object. With this, 00127 * the client can respond to different queries from the server (GET,PUT etc). 00128 * This type of resource is also observable and carries callbacks. 00129 * @param resource_name, Name of the resource. 00130 * @param resource_type, Type of the resource. 00131 * @param observable, Indicates, whether the resource is observable or not. 00132 * @param instance_id, Instance Id of the resource. 00133 * @return M2MResourceInstance, Resource Instance for managing other client operations. 00134 */ 00135 M2MResourceInstance* create_dynamic_resource_instance(const String &resource_name, 00136 const String &resource_type, 00137 M2MResourceInstance::ResourceType type, 00138 bool observable, 00139 uint16_t instance_id); 00140 00141 /** 00142 * @brief Removes the resource with the given name. 00143 * @param name, Name of the resource to be removed. 00144 * @return True if removed, else false. 00145 */ 00146 virtual bool remove_resource(const String &name); 00147 00148 /** 00149 * @brief Removes the resource instance with the given name. 00150 * @param resource_name, Name of the resource instance to be removed. 00151 * @param instance_id, Instance Id of the instance. 00152 * @return True if removed, else false. 00153 */ 00154 virtual bool remove_resource_instance(const String &resource_name, 00155 uint16_t instance_id); 00156 00157 /** 00158 * @brief Returns resource with the given name. 00159 * @param name, Name of the requested resource. 00160 * @return Resource reference if found, else NULL. 00161 */ 00162 virtual M2MResource* resource(const String &name) const; 00163 00164 /** 00165 * @brief Returns a list of M2MResourceBase objects. 00166 * @return List of Resource base with the object instance. 00167 */ 00168 virtual const M2MResourceList& resources() const; 00169 00170 /** 00171 * @brief Returns the total number of resources with the object. 00172 * @return Total number of the resources. 00173 */ 00174 virtual uint16_t resource_count() const; 00175 00176 /** 00177 * @brief Returns the total number of single resource instances. 00178 * @param resource, Name of the resource. 00179 * @return Total number of the resources. 00180 */ 00181 virtual uint16_t resource_count(const String& resource) const; 00182 00183 /** 00184 * @brief Returns object type. 00185 * @return BaseType. 00186 */ 00187 virtual M2MBase::BaseType base_type() const; 00188 00189 /** 00190 * @brief Adds the observation level for the object. 00191 * @param observation_level, Level of observation. 00192 */ 00193 virtual void add_observation_level(M2MBase::Observation observation_level); 00194 00195 /** 00196 * @brief Removes the observation level from the object. 00197 * @param observation_level, Level of observation. 00198 */ 00199 virtual void remove_observation_level(M2MBase::Observation observation_level); 00200 00201 /** 00202 * @brief Handles GET request for the registered objects. 00203 * @param nsdl, NSDL handler for the CoAP library. 00204 * @param received_coap_header, CoAP message received from the server. 00205 * @param observation_handler, Handler object for sending 00206 * observation callbacks. 00207 * @return sn_coap_hdr_s, Message that needs to be sent to the server. 00208 */ 00209 virtual sn_coap_hdr_s* handle_get_request(nsdl_s *nsdl, 00210 sn_coap_hdr_s *received_coap_header, 00211 M2MObservationHandler *observation_handler = NULL); 00212 /** 00213 * @brief Handles PUT request for the registered objects. 00214 * @param nsdl, NSDL handler for the CoAP library. 00215 * @param received_coap_header, CoAP message received from the server. 00216 * @param observation_handler, Handler object for sending 00217 * observation callbacks. 00218 * @return sn_coap_hdr_s, Message that needs to be sent to the server. 00219 */ 00220 virtual sn_coap_hdr_s* handle_put_request(nsdl_s *nsdl, 00221 sn_coap_hdr_s *received_coap_header, 00222 M2MObservationHandler *observation_handler = NULL); 00223 00224 /** 00225 * @brief Handles GET request for the registered objects. 00226 * @param nsdl, NSDL handler for the CoAP library. 00227 * @param received_coap_header, CoAP message received from the server. 00228 * @param observation_handler, Handler object for sending 00229 * observation callbacks. 00230 * @return sn_coap_hdr_s, Message that needs to be sent to the server. 00231 */ 00232 virtual sn_coap_hdr_s* handle_post_request(nsdl_s *nsdl, 00233 sn_coap_hdr_s *received_coap_header, 00234 M2MObservationHandler *observation_handler = NULL); 00235 00236 00237 protected : 00238 00239 virtual void notification_update(M2MBase::Observation observation_level); 00240 00241 private: 00242 00243 M2MObjectCallback &_object_callback; 00244 M2MResourceList _resource_list; // owned 00245 00246 friend class Test_M2MObjectInstance; 00247 friend class Test_M2MObject; 00248 friend class Test_M2MDevice; 00249 friend class Test_M2MSecurity; 00250 friend class Test_M2MServer; 00251 friend class Test_M2MNsdlInterface; 00252 friend class Test_M2MFirmware; 00253 friend class Test_M2MTLVSerializer; 00254 friend class Test_M2MTLVDeserializer; 00255 }; 00256 00257 #endif // M2M_OBJECT_INSTANCE_H
Generated on Tue Jul 12 2022 18:27:24 by
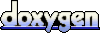