
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
m2minterfacefactory.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed-client/m2minterfacefactory.h" 00017 #include "mbed-client/m2mserver.h" 00018 #include "mbed-client/m2mdevice.h" 00019 #include "mbed-client/m2mfirmware.h" 00020 #include "mbed-client/m2mobject.h" 00021 #include "mbed-client/m2mconstants.h" 00022 #include "mbed-client/m2mconfig.h" 00023 #include "include/m2minterfaceimpl.h" 00024 #include "ns_trace.h" 00025 00026 M2MInterface* M2MInterfaceFactory::create_interface(M2MInterfaceObserver &observer, 00027 const String &endpoint_name, 00028 const String &endpoint_type, 00029 const int32_t life_time, 00030 const uint16_t listen_port, 00031 const String &domain, 00032 M2MInterface::BindingMode mode, 00033 M2MInterface::NetworkStack stack, 00034 const String &context_address) 00035 { 00036 tr_debug("M2MInterfaceFactory::create_interface - IN"); 00037 tr_debug("M2MInterfaceFactory::create_interface - parameters endpoint name : %s",endpoint_name.c_str()); 00038 tr_debug("M2MInterfaceFactory::create_interface - parameters endpoint type : %s",endpoint_type.c_str()); 00039 tr_debug("M2MInterfaceFactory::create_interface - parameters life time(in secs): %d",life_time); 00040 tr_debug("M2MInterfaceFactory::create_interface - parameters Listen Port : %d",listen_port); 00041 tr_debug("M2MInterfaceFactory::create_interface - parameters Binding Mode : %d",(int)mode); 00042 tr_debug("M2MInterfaceFactory::create_interface - parameters NetworkStack : %d",(int)stack); 00043 M2MInterfaceImpl *interface = NULL; 00044 00045 00046 bool endpoint_type_valid = true; 00047 if(!endpoint_type.empty()) { 00048 if(endpoint_type.size() > MAX_ALLOWED_STRING_LENGTH){ 00049 endpoint_type_valid = false; 00050 } 00051 } 00052 00053 bool domain_valid = true; 00054 if(!domain.empty()) { 00055 if(domain.size() > MAX_ALLOWED_STRING_LENGTH){ 00056 domain_valid = false; 00057 } 00058 } 00059 00060 if(((life_time == -1) || (life_time >= MINIMUM_REGISTRATION_TIME)) && 00061 !endpoint_name.empty() && (endpoint_name.size() <= MAX_ALLOWED_STRING_LENGTH) && 00062 endpoint_type_valid && domain_valid) { 00063 tr_debug("M2MInterfaceFactory::create_interface - Creating M2MInterfaceImpl"); 00064 interface = new M2MInterfaceImpl(observer, endpoint_name, 00065 endpoint_type, life_time, 00066 listen_port, domain, mode, 00067 stack, context_address); 00068 00069 } 00070 tr_debug("M2MInterfaceFactory::create_interface - OUT"); 00071 return interface; 00072 } 00073 00074 M2MSecurity* M2MInterfaceFactory::create_security(M2MSecurity::ServerType server_type) 00075 { 00076 tr_debug("M2MInterfaceFactory::create_security"); 00077 M2MSecurity *security = new M2MSecurity(server_type); 00078 return security; 00079 } 00080 00081 M2MServer* M2MInterfaceFactory::create_server() 00082 { 00083 tr_debug("M2MInterfaceFactory::create_server"); 00084 M2MServer *server = new M2MServer(); 00085 return server; 00086 } 00087 00088 M2MDevice* M2MInterfaceFactory::create_device() 00089 { 00090 tr_debug("M2MInterfaceFactory::create_device"); 00091 M2MDevice* device = M2MDevice::get_instance(); 00092 return device; 00093 } 00094 00095 M2MFirmware* M2MInterfaceFactory::create_firmware() 00096 { 00097 tr_debug("M2MInterfaceFactory::create_firmware"); 00098 M2MFirmware* firmware = M2MFirmware::get_instance(); 00099 return firmware; 00100 } 00101 00102 M2MObject* M2MInterfaceFactory::create_object(const String &name) 00103 { 00104 tr_debug("M2MInterfaceFactory::create_object : Name : %s", name.c_str()); 00105 if(name.size() > MAX_ALLOWED_STRING_LENGTH || name.empty()){ 00106 return NULL; 00107 } 00108 00109 M2MObject *object = NULL; 00110 object = new M2MObject(name); 00111 return object; 00112 }
Generated on Tue Jul 12 2022 18:27:24 by
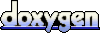