
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
m2mconstants.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2MCONSTANTS_H 00017 #define M2MCONSTANTS_H 00018 00019 #include <stdint.h> 00020 #include "m2mconfig.h" 00021 00022 const int MAX_VALUE_LENGTH = 256; 00023 const int BUFFER_LENGTH = 1024; 00024 extern const String COAP; 00025 const int32_t MINIMUM_REGISTRATION_TIME = 60; //in seconds 00026 const uint64_t ONE_SECOND_TIMER = 1; 00027 const uint16_t MAX_ALLOWED_STRING_LENGTH = 64; 00028 const uint16_t OPTIMUM_LIFETIME = 3600; 00029 const uint16_t REDUCE_LIFETIME = 900; 00030 const float REDUCTION_FACTOR = 0.75f; 00031 00032 const int RETRY_COUNT = 2; 00033 const int RETRY_INTERVAL = 5; 00034 00035 // values per: draft-ietf-core-observe-16 00036 // OMA LWM2M CR ref. 00037 #define START_OBSERVATION 0 00038 #define STOP_OBSERVATION 1 00039 00040 // PUT attributes to be checked from server 00041 extern const String EQUAL; 00042 extern const String AMP; 00043 extern const String PMIN; 00044 extern const String PMAX; 00045 extern const String GT; 00046 extern const String LT; 00047 extern const String ST; 00048 extern const String CANCEL; 00049 00050 //LWM2MOBJECT NAME/ID 00051 extern const String M2M_SECURITY_ID; 00052 extern const String M2M_SERVER_ID; 00053 extern const String M2M_ACCESS_CONTROL_ID; 00054 extern const String M2M_DEVICE_ID; 00055 00056 extern const String M2M_CONNECTIVITY_MONITOR_ID; 00057 extern const String M2M_FIRMWARE_ID; 00058 extern const String M2M_LOCATION_ID; 00059 extern const String M2M_CONNECTIVITY_STATISTICS_ID; 00060 extern const String RESERVED_ID; 00061 00062 //OBJECT RESOURCE TYPE 00063 extern const String OMA_RESOURCE_TYPE; 00064 00065 //DEVICE RESOURCES 00066 extern const String DEVICE_MANUFACTURER; 00067 extern const String DEVICE_DEVICE_TYPE; 00068 extern const String DEVICE_MODEL_NUMBER; 00069 extern const String DEVICE_SERIAL_NUMBER; 00070 extern const String DEVICE_HARDWARE_VERSION; 00071 extern const String DEVICE_FIRMWARE_VERSION; 00072 extern const String DEVICE_SOFTWARE_VERSION; 00073 extern const String DEVICE_REBOOT; 00074 extern const String DEVICE_FACTORY_RESET; 00075 extern const String DEVICE_AVAILABLE_POWER_SOURCES; 00076 extern const String DEVICE_POWER_SOURCE_VOLTAGE; 00077 extern const String DEVICE_POWER_SOURCE_CURRENT; 00078 extern const String DEVICE_BATTERY_LEVEL; 00079 extern const String DEVICE_BATTERY_STATUS; 00080 extern const String DEVICE_MEMORY_FREE; 00081 extern const String DEVICE_MEMORY_TOTAL; 00082 extern const String DEVICE_ERROR_CODE; 00083 extern const String DEVICE_RESET_ERROR_CODE; 00084 extern const String DEVICE_CURRENT_TIME; 00085 extern const String DEVICE_UTC_OFFSET; 00086 extern const String DEVICE_TIMEZONE; 00087 extern const String DEVICE_SUPPORTED_BINDING_MODE; 00088 00089 extern const String BINDING_MODE_UDP; 00090 extern const String BINDING_MODE_UDP_QUEUE; 00091 extern const String BINDING_MODE_SMS; 00092 extern const String BINDING_MODE_SMS_QUEUE; 00093 00094 extern const String ERROR_CODE_VALUE; 00095 00096 00097 00098 //SECURITY RESOURCES 00099 extern const String SECURITY_M2M_SERVER_URI; 00100 extern const String SECURITY_BOOTSTRAP_SERVER; 00101 extern const String SECURITY_SECURITY_MODE; 00102 extern const String SECURITY_PUBLIC_KEY; 00103 extern const String SECURITY_SERVER_PUBLIC_KEY; 00104 extern const String SECURITY_SECRET_KEY; 00105 extern const String SECURITY_SMS_SECURITY_MODE; 00106 extern const String SECURITY_SMS_BINDING_KEY; 00107 extern const String SECURITY_SMS_BINDING_SECRET_KEY; 00108 extern const String SECURITY_M2M_SERVER_SMS_NUMBER; 00109 extern const String SECURITY_SHORT_SERVER_ID; 00110 extern const String SECURITY_CLIENT_HOLD_OFF_TIME; 00111 00112 //SERVER RESOURCES 00113 extern const String SERVER_SHORT_SERVER_ID; 00114 extern const String SERVER_LIFETIME; 00115 extern const String SERVER_DEFAULT_MIN_PERIOD; 00116 extern const String SERVER_DEFAULT_MAX_PERIOD; 00117 extern const String SERVER_DISABLE; 00118 extern const String SERVER_DISABLE_TIMEOUT; 00119 extern const String SERVER_NOTIFICATION_STORAGE; 00120 extern const String SERVER_BINDING; 00121 extern const String SERVER_REGISTRATION_UPDATE; 00122 00123 //FIRMWARE RESOURCES 00124 extern const String FIRMWARE_PACKAGE; 00125 extern const String FIRMWARE_PACKAGE_URI; 00126 extern const String FIRMWARE_UPDATE; 00127 extern const String FIRMWARE_STATE; 00128 extern const String FIRMWARE_UPDATE_SUPPORTED_OBJECTS; 00129 extern const String FIRMWARE_UPDATE_RESULT; 00130 extern const String FIRMWARE_PACKAGE_NAME; 00131 extern const String FIRMWARE_PACKAGE_VERSION; 00132 00133 // TLV serializer / deserializer 00134 const uint8_t TYPE_RESOURCE = 0xC0; 00135 const uint8_t TYPE_MULTIPLE_RESOURCE = 0x80; 00136 const uint8_t TYPE_RESOURCE_INSTANCE = 0x40; 00137 const uint8_t TYPE_OBJECT_INSTANCE = 0x0; 00138 00139 const uint8_t ID8 = 0x0; 00140 const uint8_t ID16 = 0x20; 00141 00142 const uint8_t LENGTH8 = 0x08; 00143 const uint8_t LENGTH16 = 0x10; 00144 const uint8_t LENGTH24 = 0x18; 00145 00146 const uint8_t COAP_CONTENT_OMA_TLV_TYPE = 99; 00147 const uint8_t COAP_CONTENT_OMA_JSON_TYPE = 100; 00148 00149 const uint16_t MAX_UNINT_16_COUNT = 65535; 00150 00151 #endif // M2MCONSTANTS_H
Generated on Tue Jul 12 2022 18:27:23 by
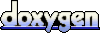