
mbed client lightswitch demo
Dependencies: mbed Socket lwip-eth lwip-sys lwip
Fork of mbed-client-classic-example-lwip by
m2mconnectionhandler.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_CONNECTION_HANDLER_H__ 00017 #define M2M_CONNECTION_HANDLER_H__ 00018 00019 #include "mbed-client/m2mconnectionobserver.h" 00020 #include "mbed-client/m2mconfig.h" 00021 #include "mbed-client/m2minterface.h" 00022 #include "nsdl-c/sn_nsdl.h" 00023 00024 class M2MConnectionSecurity; 00025 class M2MConnectionHandlerPimpl; 00026 00027 /** 00028 * @brief M2MConnectionHandler. 00029 * This class handles the socket connection for the LWM2M Client. 00030 */ 00031 00032 class M2MConnectionHandler { 00033 public: 00034 00035 /** 00036 * @enum ConnectionError 00037 * This enum defines an error that can come from 00038 * socket read and write operation. 00039 */ 00040 typedef enum { 00041 CONNECTION_ERROR_WANTS_READ = -1000, 00042 CONNECTION_ERROR_WANTS_WRITE = -1001 00043 }ConnectionError; 00044 00045 public: 00046 00047 /** 00048 * @brief Constructor 00049 */ 00050 M2MConnectionHandler(M2MConnectionObserver &observer, 00051 M2MConnectionSecurity* sec, 00052 M2MInterface::BindingMode mode, 00053 M2MInterface::NetworkStack stack); 00054 00055 /** 00056 * @brief Destructor 00057 */ 00058 ~M2MConnectionHandler(); 00059 00060 /** 00061 * @brief This binds the socket connection. 00062 * @param listen_port Port to be listened to for an incoming connection. 00063 * @return True if successful, else false. 00064 */ 00065 bool bind_connection(const uint16_t listen_port); 00066 00067 /** 00068 * @brief This resolves the server address. Output is 00069 * returned through a callback. 00070 * @param String Server address. 00071 * @param uint16_t Server port. 00072 * @param ServerType, Server Type to be resolved. 00073 * @param security, M2MSecurity object that determines what 00074 * type of secure connection will be used by the socket. 00075 * @return True if address is valid, else false. 00076 */ 00077 bool resolve_server_address(const String& server_address, 00078 const uint16_t server_port, 00079 M2MConnectionObserver::ServerType server_type, 00080 const M2MSecurity* security); 00081 00082 /** 00083 * @brief Sends data to the connected server. 00084 * @param data_ptr, Data to be sent. 00085 * @param data_len, Length of data to be sent. 00086 * @param address_ptr, Address structure where data has to be sent. 00087 * @return True if data is sent successfully, else false. 00088 */ 00089 bool send_data(uint8_t *data_ptr, 00090 uint16_t data_len, 00091 sn_nsdl_addr_s *address_ptr); 00092 00093 /** 00094 * @brief Listens to the incoming data from a remote server. 00095 * @return True if successful, else false. 00096 */ 00097 bool start_listening_for_data(); 00098 00099 /** 00100 * @brief Stops listening to the incoming data. 00101 */ 00102 void stop_listening(); 00103 00104 /** 00105 * @brief sendToSocket Sends directly to socket. This is used by 00106 * security classes to send the data after it has been encrypted. 00107 * @param buf Buffer to send. 00108 * @param len Length of a buffer. 00109 * @return Number of bytes sent or -1 if failed. 00110 */ 00111 int send_to_socket(const unsigned char *buf, size_t len); 00112 00113 /** 00114 * @brief receiveFromSocket Receives directly from a socket. This 00115 * is used by the security classes to receive raw data to be decrypted. 00116 * @param buf Buffer to send. 00117 * @param len Length of a buffer. 00118 * @return Number of bytes read or -1 if failed. 00119 */ 00120 int receive_from_socket(unsigned char *buf, size_t len); 00121 00122 /** 00123 * @brief Closes the open connection. 00124 */ 00125 void close_connection(); 00126 00127 /** 00128 * @brief Error handling for DTLS connectivity. 00129 * @param error, Error code from TLS library 00130 */ 00131 void handle_connection_error(int error); 00132 00133 private: 00134 00135 M2MConnectionObserver &_observer; 00136 M2MConnectionHandlerPimpl *_private_impl; 00137 00138 friend class Test_M2MConnectionHandler; 00139 friend class Test_M2MConnectionHandler_mbed; 00140 friend class Test_M2MConnectionHandler_linux; 00141 friend class M2MConnection_TestObserver; 00142 }; 00143 00144 #endif //M2M_CONNECTION_HANDLER_H__ 00145
Generated on Tue Jul 12 2022 18:27:23 by
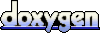