
Embed:
(wiki syntax)
Show/hide line numbers
OS_Target_cpp.cpp
00001 //****************************************************************************** 00002 //* 00003 //* FULLNAME: Single-Chip Microcontroller Real-Time Operating System 00004 //* 00005 //* NICKNAME: scmRTOS 00006 //* 00007 //* PROCESSOR: ARM Cortex-M3 00008 //* 00009 //* TOOLKIT: EWARM (IAR Systems) 00010 //* 00011 //* PURPOSE: Target Dependent Stuff Source 00012 //* 00013 //* Version: 3.10 00014 //* 00015 //* $Revision: 195 $ 00016 //* $Date:: 2008-06-19 #$ 00017 //* 00018 //* Copyright (c) 2003-2010, Harry E. Zhurov 00019 //* 00020 //* Permission is hereby granted, free of charge, to any person 00021 //* obtaining a copy of this software and associated documentation 00022 //* files (the "Software"), to deal in the Software without restriction, 00023 //* including without limitation the rights to use, copy, modify, merge, 00024 //* publish, distribute, sublicense, and/or sell copies of the Software, 00025 //* and to permit persons to whom the Software is furnished to do so, 00026 //* subject to the following conditions: 00027 //* 00028 //* The above copyright notice and this permission notice shall be included 00029 //* in all copies or substantial portions of the Software. 00030 //* 00031 //* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00032 //* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00033 //* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00034 //* IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY 00035 //* CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, 00036 //* TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH 00037 //* THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00038 //* 00039 //* ================================================================= 00040 //* See http://scmrtos.sourceforge.net for documentation, latest 00041 //* information, license and contact details. 00042 //* ================================================================= 00043 //* 00044 //****************************************************************************** 00045 //* Ported by Andrey Chuikin, Copyright (c) 2008-2010 00046 00047 00048 #include <scmRTOS.h> 00049 00050 using namespace OS; 00051 00052 //------------------------------------------------------------------------------ 00053 // 00054 // OS Process's constructor 00055 // 00056 // Performs: 00057 // * initializing process data; 00058 // * registering of the process in kernel; 00059 // * preparing stack frame; 00060 // 00061 // 00062 TBaseProcess::TBaseProcess(TStackItem* Stack, word stack_size, TPriority pr, void (*exec)()) 00063 : StackPointer(Stack) 00064 , StackSize(stack_size) 00065 , Timeout(0) 00066 , Priority(pr) 00067 { 00068 Kernel.RegisterProcess(this); 00069 00070 #ifdef DEBUG_STACK 00071 //--------------------------------------------------------------- 00072 // Fill stack area with some value to be able later to define stack usage 00073 for (StackPointer-=(StackSize/sizeof(TStackItem)); StackPointer < Stack; *(StackPointer++) = STACK_FILL_CONST); 00074 #endif //DEBUG_STACK 00075 00076 //--------------------------------------------------------------- 00077 // 00078 // Prepare Process Stack Frame 00079 // 00080 *(--StackPointer) = 0x01000000L; // xPSR 00081 *(--StackPointer) = reinterpret_cast<dword>(exec); // Entry Point 00082 StackPointer -= 14; // emulate "push R14,R12,R3,R2,R1,R0,R11-R4" 00083 00084 // The code below can be used for debug purpose. In this case comment 00085 // line above and uncomment block below. 00086 /* 00087 *(--StackPointer) = 0xFFFFFFFEL; // R14 (LR) (init value will cause fault if ever used) 00088 *(--StackPointer) = 0x12121212L; // R12 00089 *(--StackPointer) = 0x03030303L; // R3 00090 *(--StackPointer) = 0x02020202L; // R2 00091 *(--StackPointer) = 0x01010101L; // R1 00092 *(--StackPointer) = 0x00000000L; // R0 00093 00094 // Remaining registers saved on process stack 00095 *(--StackPointer) = 0x11111111L; // R11 00096 *(--StackPointer) = 0x10101010L; // R10 00097 *(--StackPointer) = 0x09090909L; // R9 00098 *(--StackPointer) = 0x08080808L; // R8 00099 *(--StackPointer) = 0x07070707L; // R7 00100 *(--StackPointer) = 0x06060606L; // R6 00101 *(--StackPointer) = 0x05050505L; // R5 00102 *(--StackPointer) = 0x04040404L; // R4 00103 */ 00104 } 00105 //------------------------------------------------------------------------------ 00106 // 00107 // Idle Process 00108 // 00109 typedef process<prIDLE, scmRTOS_IDLE_PROCESS_STACK_SIZE> TIdleProcess; 00110 00111 TIdleProcess IdleProcess; 00112 00113 template<> OS_PROCESS void TIdleProcess::Exec() 00114 { 00115 for(;;) 00116 { 00117 #if scmRTOS_IDLE_HOOK_ENABLE == 1 00118 IdleProcessUserHook(); 00119 #endif 00120 } 00121 } 00122 //------------------------------------------------------------------------------ 00123 OS_INTERRUPT void OS::SysTick_Handler() 00124 { 00125 scmRTOS_ISRW_TYPE ISR; 00126 00127 Kernel.SystemTimer(); 00128 00129 #if scmRTOS_SYSTIMER_NEST_INTS_ENABLE == 0 00130 DISABLE_NESTED_INTERRUPTS(); 00131 #endif 00132 00133 #if scmRTOS_SYSTIMER_HOOK_ENABLE == 1 00134 SystemTimerUserHook(); 00135 #endif 00136 } 00137 //------------------------------------------------------------------------------
Generated on Tue Jul 12 2022 21:14:46 by
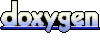