
A reverse delay to help with the study of reverse speech
Fork of Reverseguitar20kcutoff by
main.cpp
00001 #include <mbed.h> 00002 00003 // Boolean types 00004 #define TRUE 1 00005 #define FALSE 0 00006 00007 /* ADC for the microphone/input, DAC for the speaker/output */ 00008 AnalogIn mic(p19); 00009 AnalogOut speaker(p18); 00010 00011 // Allocate a buffer to be used for the audio recording 00012 static const size_t BufferSize = 15 * 1024; 00013 static unsigned short Buffer[BufferSize]; 00014 00015 00016 int main(void) 00017 { 00018 unsigned short ReadSample = 0xFFFF; 00019 // Indices to track where the playback and recording should take place in the 00020 // audio buffer. The recording can occur one sample behind the current playback 00021 // index since it is no longer required. 00022 int Index = 0; 00023 // Reverse the direction the buffer is walked between each iteration to save memory 00024 int Direction = 1; 00025 // Have audio to playback 00026 int Playback = FALSE; 00027 // The amount of data to be recorded before starting reverse playback 00028 // NOTE: Probably want this to be configured at runtime via a knob, etc. 00029 int ChunkSize = BufferSize; 00030 00031 // Infinite loop of recording and reverse playback 00032 for (;;) 00033 { 00034 unsigned short PlaySample; 00035 00036 // Slow down the sampling rate so that it doesn't exceed 20kHz 00037 wait_us(50); 00038 00039 // Read out the sample from the buffer to be played back 00040 if (Playback) 00041 { 00042 PlaySample = Buffer[Index]; 00043 speaker.write_u16(PlaySample); 00044 } 00045 00046 // Obtain current audio sample from the A/D converter. 00047 ReadSample = mic.read_u16(); 00048 00049 // Record the sample into the buffer right where a space was freed up from the PlaySample read above 00050 Buffer[Index] = ReadSample; 00051 00052 // Increment the buffer pointer 00053 Index += Direction; 00054 00055 // Check to see if the chunk has been filled 00056 if (Index < 0) 00057 { 00058 // Now have a chunk to be played back 00059 Playback = TRUE; 00060 // Reverse the direction of playback and recording 00061 Direction *= -1; 00062 Index = 0; 00063 } 00064 else if (Index >= ChunkSize) 00065 { 00066 // Now have a chunk to be played back 00067 Playback = TRUE; 00068 // Reverse the direction of playback and recording 00069 Direction *= -1; 00070 Index = ChunkSize - 1; 00071 } 00072 } 00073 }
Generated on Wed Jul 20 2022 12:09:55 by
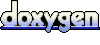