Elements used in the Balls and Things games for the RETRO.
Dependents: RETRO_BallsAndPaddle RETRO_BallAndHoles
Ball.h
00001 #pragma once 00002 #include "mbed.h" 00003 00004 #include "Color565.h" 00005 #include "font_OEM.h" 00006 #include "LCD_ST7735.h" 00007 00008 #include "Shapes.h" 00009 #include "Vector.h" 00010 #include "Physics.h" 00011 00012 class Ball 00013 { 00014 public: 00015 static const bool fFixed=false; 00016 bool fActive; 00017 00018 Ball(); 00019 Ball(LCD_ST7735* pDisp); 00020 void initialize(int X, int Y, int R, uint16_t uColor); 00021 void setSpeed(int X, int Y); 00022 void changeSpeed(bool fUp); 00023 void unmove(); 00024 void update(); 00025 void clear(); 00026 void clearPrev(); 00027 void draw(); 00028 void redraw(); 00029 00030 Position pos; 00031 int nRadius; 00032 Vector vSpeed; 00033 00034 Circle getBoundingCircle(); 00035 bool collides(Rectangle r); 00036 bool collides(Circle cObject); 00037 bool collides(Line ln); 00038 void Bounce(Vector vBounce); 00039 void BounceAgainst(Vector vBounce); 00040 00041 private: 00042 uint16_t uColor; 00043 uint16_t uColorHigh; 00044 uint16_t uColorMid; 00045 uint16_t uColorLow; 00046 LCD_ST7735* pDisp; 00047 00048 uint16_t dimmedColor(uint16_t uColor); 00049 }; 00050
Generated on Thu Jul 14 2022 10:55:45 by
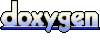