
mbed1 Tapton school eds robot project
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Mbed 1 Robot, robot base sensors and bluetooth 00002 // serial port pins 9 and 10(tx rx) used to communicate with robot base 00003 #include "mbed.h" 00004 #include "m3pi.h" 00005 #include "string" 00006 #include <stdio.h> 00007 #include <stdlib.h> 00008 #include <iostream> 00009 //Serial pc(USBTX, USBRX); // for terminal diagnostics 00010 Serial device(p28, p27); // Bluetooth comms tx rx 00011 Serial communicate(p13, p14); // to Mbed 2 tx rx 00012 m3pi m3pi; 00013 DigitalOut myled1(LED1); 00014 DigitalOut myled2(LED2); 00015 DigitalOut myled3(LED3); 00016 DigitalOut myled4(LED4); 00017 DigitalIn stopgo (p20); // from mbed2 sensor move forward or not something blocking (1=block) 00018 00019 char letters[26] = { 00020 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 00021 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 00022 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z' 00023 }; 00024 char receivedchar[10]; 00025 00026 00027 void rxCallback() //interrupt from rx serial port 00028 { 00029 myled3=!myled3; 00030 receivedchar[0]=communicate.getc(); // put received character from mbed2 into array for later 00031 // pc.printf("%d ",receivedchar[0]); 00032 //m3pi.cls(); 00033 m3pi.locate(0,0); // x then y co-ordinates on screen 00034 m3pi.printf("%d ",receivedchar[0]); 00035 //wait (0.2); 00036 } 00037 00038 void txCallback() //interrupt from tx serial port 00039 { 00040 myled4=!myled4; 00041 } 00042 00043 int main() 00044 { 00045 int cmd_option; 00046 char cmd_convert; 00047 // Display welcome message to the scripting 00048 // display_part("Init : ", "FIDO-Ed"); 00049 00050 // Configure device baud rates 00051 device.baud(115200); //Bluetooth 00052 // pc.baud(115200); //for diagnostics pc 00053 communicate.baud(19200); //to mbed 2 00054 setbuf(stdin,NULL); //clear serial input buffer 00055 communicate.attach(&txCallback, Serial::TxIrq); 00056 communicate.attach(&rxCallback, Serial::RxIrq); //for serial interrupt callback function 00057 m3pi.cls(); 00058 cmd_convert=0; 00059 00060 // Global program variables 00061 bool nerror = true; 00062 00063 // Iterate while simulation is error free 00064 while(nerror) 00065 { 00066 myled1=stopgo; // mbed2 says stop or go - 1/on =something blocking movement 00067 // Check for readability of device 00068 if(device.readable()) 00069 { 00070 // Display device recieved transactions 00071 // Fetch command and cast to char value 00072 cmd_option = device.getc(); 00073 cmd_convert = static_cast<char>(cmd_option); 00074 m3pi.locate(0,1); 00075 m3pi.printf("B%c %d", cmd_convert,cmd_option); 00076 // Hold for half a second 00077 wait(0.2); 00078 } 00079 00080 if (cmd_convert !=0) 00081 { 00082 if(cmd_convert >=49 && cmd_convert <=53) // numbers 1 to 5 from mobile app, Movement 00083 { 00084 // Determine action to be taken based on switching 00085 switch(cmd_convert) 00086 { 00087 00088 case '1': //Move Forward 00089 if(!stopgo) //if nothing blocking way 00090 { 00091 // while (!stopgo) 00092 // { 00093 communicate.putc(49); //move forward - send 1 to mbed 2 00094 cmd_convert=0; 00095 m3pi.forward(0.25); 00096 wait(1.1); 00097 m3pi.stop(); 00098 // } 00099 } 00100 else 00101 { 00102 communicate.putc(53); // dont move forward and send 5 to mbed 2 00103 } 00104 m3pi.stop(); 00105 break; 00106 00107 case '2': //Turn Right 00108 if(!stopgo) //if nothing blocking way 00109 { 00110 // while (!stopgo) 00111 // { 00112 communicate.putc(50);// turn right and send 2 to mbed2 00113 cmd_convert=0; 00114 m3pi.right(0.25); 00115 wait(0.5); 00116 m3pi.stop(); 00117 // } 00118 } 00119 else 00120 { 00121 m3pi.stop(); 00122 communicate.putc(53); // dont move forward and send 5 to mbed 2 00123 } 00124 break; 00125 00126 case '3': //Backwards - no movement 00127 communicate.putc(51); // turn around and send 3 to mbed 2 00128 cmd_convert=0; 00129 // m3pi.right(0.5); 00130 // wait(0.25); 00131 // m3pi.stop(); 00132 break; 00133 00134 case '4': // Turn Left 00135 if(!stopgo) //if nothing blocking way 00136 { 00137 // while (!stopgo) 00138 // { 00139 communicate.putc(52); //turn left and send 4 to mbed2 00140 cmd_convert=0; 00141 m3pi.left(0.25); 00142 wait(0.5); 00143 m3pi.stop(); 00144 // } 00145 } 00146 else 00147 { 00148 m3pi.stop(); 00149 communicate.putc(53); // dont move forward and send 5 to mbed 2 00150 } 00151 break; 00152 00153 00154 } // switch 00155 } 00156 if(cmd_convert >=65 && cmd_convert <=122) // send any other valid command to mbed2 00157 { 00158 communicate.putc(cmd_convert); //send cmd_convert to mbed 2 00159 cmd_convert=0; 00160 } //cmd_convert >=65 00161 } // if cmd_convert not 0 00162 }}
Generated on Wed Jul 13 2022 00:37:33 by
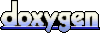