library for Sharp memory LCD (LS027B4DH01)
Dependents: Sharp_Memory_LCD_Test Sharp_Memory_LCD_Test
sharp_mlcd.h
00001 /* 00002 * mbed library for the Sharp memory LCD LS027BDH01 / LS013B4DN04 00003 * derived from C12832_lcd 00004 * Copyright (c) 2014 Masato YAMANISHI 00005 * Released under the MIT License: http://mbed.org/license/mit 00006 */ 00007 00008 /* mbed library for the mbed Lab Board 128*32 pixel LCD 00009 * use C12832 controller 00010 * Copyright (c) 2012 Peter Drescher - DC2PD 00011 * Released under the MIT License: http://mbed.org/license/mit 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00014 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00015 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00016 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00017 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00019 * THE SOFTWARE. 00020 */ 00021 00022 #ifndef SHARP_MLCD_H 00023 #define SHARP_MLCD_H 00024 00025 #include "mbed.h" 00026 #include "GraphicsDisplay.h" 00027 00028 /** optional Defines : 00029 * #define debug_lcd 1 enable infos to PC_USB 00030 */ 00031 00032 #define BPP 1 // Bits per pixel 00033 #define WIDTH 400 // 96 00034 #define HEIGHT 240 // 96 00035 #define PAGES (HEIGHT/8) // 6 00036 #define BUFFER_SIZE (WIDTH*HEIGHT/8) 00037 00038 00039 /** Draw mode 00040 * NORMAl 00041 * XOR set pixel by xor the screen 00042 */ 00043 enum {NORMAL,XOR}; 00044 00045 /** Bitmap 00046 */ 00047 struct Bitmap{ 00048 int xSize; 00049 int ySize; 00050 int Byte_in_Line; 00051 char* data; 00052 }; 00053 00054 class sharp_mlcd : public GraphicsDisplay 00055 { 00056 public: 00057 /** Create a aqm1248a object connected to SPI1 00058 * 00059 */ 00060 00061 sharp_mlcd(const char* name = "LCD"); 00062 00063 /** Get the width of the screen in pixel 00064 * 00065 * @param 00066 * @returns width of screen in pixel 00067 * 00068 */ 00069 virtual int width(); 00070 00071 /** Get the height of the screen in pixel 00072 * 00073 * @returns height of screen in pixel 00074 * 00075 */ 00076 virtual int height(); 00077 00078 /** Draw a pixel at x,y black or white 00079 * 00080 * @param x horizontal position 00081 * @param y vertical position 00082 * @param colour ,1 set pixel ,0 erase pixel 00083 */ 00084 virtual void pixel(int x, int y,int colour); 00085 00086 /** draw a circle 00087 * 00088 * @param x0,y0 center 00089 * @param r radius 00090 * @param colour ,1 set pixel ,0 erase pixel 00091 * 00092 */ 00093 void circle(int x, int y, int r, int colour); 00094 00095 /** draw a filled circle 00096 * 00097 * @param x0,y0 center 00098 * @param r radius 00099 * @param color ,1 set pixel ,0 erase pixel 00100 * 00101 * use circle with different radius, 00102 * can miss some pixel 00103 */ 00104 void fillcircle(int x, int y, int r, int colour); 00105 00106 /** draw a 1 pixel line 00107 * 00108 * @param x0,y0 start point 00109 * @param x1,y1 stop point 00110 * @param color ,1 set pixel ,0 erase pixel 00111 * 00112 */ 00113 void line(int x0, int y0, int x1, int y1, int colour); 00114 00115 /** draw a rect 00116 * 00117 * @param x0,y0 top left corner 00118 * @param x1,y1 down right corner 00119 * @param color 1 set pixel ,0 erase pixel 00120 * * 00121 */ 00122 void rect(int x0, int y0, int x1, int y1, int colour); 00123 00124 /** draw a filled rect 00125 * 00126 * @param x0,y0 top left corner 00127 * @param x1,y1 down right corner 00128 * @param color 1 set pixel ,0 erase pixel 00129 * 00130 */ 00131 void fillrect(int x0, int y0, int x1, int y1, int colour); 00132 00133 /** copy display buffer to lcd 00134 * 00135 */ 00136 00137 void copy_to_lcd(void); 00138 00139 /** clear the screen 00140 * 00141 */ 00142 virtual void cls(void); 00143 00144 /** set the drawing mode 00145 * 00146 * @param mode NORMAl or XOR 00147 */ 00148 00149 void setmode(int mode); 00150 00151 virtual int columns(void); 00152 00153 /** calculate the max number of columns 00154 * 00155 * @returns max column 00156 * depends on actual font size 00157 * 00158 */ 00159 virtual int rows(void); 00160 00161 /** put a char on the screen 00162 * 00163 * @param value char to print 00164 * @returns printed char 00165 * 00166 */ 00167 virtual int _putc(int value); 00168 00169 /** draw a character on given position out of the active font to the LCD 00170 * 00171 * @param x x-position of char (top left) 00172 * @param y y-position 00173 * @param c char to print 00174 * 00175 */ 00176 virtual void character(int x, int y, int c); 00177 00178 /** setup cursor position 00179 * 00180 * @param x x-position (top left) 00181 * @param y y-position 00182 */ 00183 virtual void locate(int x, int y); 00184 00185 /** setup auto update of screen 00186 * 00187 * @param up 1 = on , 0 = off 00188 * if switched off the program has to call copy_to_lcd() 00189 * to update screen from framebuffer 00190 */ 00191 void set_auto_up(unsigned int up); 00192 00193 /** get status of the auto update function 00194 * 00195 * @returns if auto update is on 00196 */ 00197 unsigned int get_auto_up(void); 00198 00199 /** Vars */ 00200 SPI _spi; 00201 // DigitalOut _reset; 00202 // DigitalOut _A0; 00203 DigitalOut _EXTCOM; 00204 DigitalOut _DISP; 00205 DigitalOut _CS; 00206 unsigned char* font; 00207 unsigned int draw_mode; 00208 00209 // Ticker timer; 00210 00211 /** select the font to use 00212 * 00213 * @param f pointer to font array 00214 * 00215 * font array can created with GLCD Font Creator from http://www.mikroe.com 00216 * you have to add 4 parameter at the beginning of the font array to use: 00217 * - the number of byte / char 00218 * - the vertial size in pixel 00219 * - the horizontal size in pixel 00220 * - the number of byte per vertical line 00221 * you also have to change the array to char[] 00222 * 00223 */ 00224 void set_font(unsigned char* f); 00225 00226 /** print bitmap to buffer 00227 * 00228 * @param bm Bitmap in flash 00229 * @param x x start 00230 * @param y y start 00231 * 00232 */ 00233 00234 void print_bm(Bitmap bm, int x, int y, int color); 00235 void attime(); 00236 void set_reverse_mode(unsigned int r); 00237 00238 protected: 00239 00240 /** draw a horizontal line 00241 * 00242 * @param x0 horizontal start 00243 * @param x1 horizontal stop 00244 * @param y vertical position 00245 * @param ,1 set pixel ,0 erase pixel 00246 * 00247 */ 00248 void hline(int x0, int x1, int y, int colour); 00249 00250 /** draw a vertical line 00251 * 00252 * @param x horizontal position 00253 * @param y0 vertical start 00254 * @param y1 vertical stop 00255 * @param ,1 set pixel ,0 erase pixel 00256 */ 00257 void vline(int y0, int y1, int x, int colour); 00258 00259 /** Init the C12832 LCD controller 00260 * 00261 */ 00262 void lcd_reset(); 00263 00264 /** Write data to the LCD controller 00265 * 00266 * @param dat data written to LCD controller 00267 * 00268 */ 00269 void wr_dat(unsigned char value); 00270 00271 /** Write a command the LCD controller 00272 * 00273 * @param cmd: command to be written 00274 * 00275 */ 00276 void wr_cmd(unsigned char value); 00277 00278 void wr_cnt(unsigned char cmd); 00279 00280 // unsigned int orientation; 00281 unsigned int char_x; 00282 unsigned int char_y; 00283 unsigned char buffer[BUFFER_SIZE]; 00284 unsigned int reverse; 00285 unsigned int auto_up; 00286 00287 unsigned int flip; 00288 00289 unsigned char bit_reverse(unsigned char c); 00290 }; 00291 00292 #endif
Generated on Tue Jul 12 2022 20:55:35 by
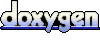