BLE switch interface with GROVE joystic for micro:bit http://mahoro-ba.net/e2073.html
Embed:
(wiki syntax)
Show/hide line numbers
KeyValueInt.cpp
00001 //================================= 00002 // Class KeyValueInt 00003 //================================= 00004 // The MIT License (MIT) Copyright (c) 2018 Masatomo Kojima 00005 // 00006 // Keys and values are stored in pairs, maximum and minimum values are set, 00007 // and values can be easily increased or decreased. 00008 // キーと値をペアで記憶する。値の最大値、最小値を設定し、値の増減を容易にする。 00009 00010 #include "KeyValueInt.h" 00011 00012 /** ---------- 00013 * @brief キーと値をペアで記憶する。値の最大値、最小値を設定し、値の増減を容易にする。 00014 * @param *key キー 00015 * @param disp LED表示の文字 00016 * @param data データ 00017 * @param min 最小値 00018 * @param max 最大値 00019 * @param rotation true:循環する false:循環しない 00020 */ 00021 KeyValueInt::KeyValueInt(const char *key, char disp, int data, int min, int max, bool rotation) { 00022 this->key = key; 00023 this->disp = (disp==0 ? key[0] : disp); 00024 this->value = data; 00025 this->min = min; 00026 this->max = max; 00027 this->rotation = rotation; 00028 set(data); 00029 } 00030 00031 /** ---------- 00032 * @brief 最大値と最小値を考慮して、正しい範囲の値を返す 00033 * @param data データ 00034 */ 00035 int KeyValueInt::range(int data){ 00036 if (this->rotation) { 00037 if (data < this->min) data = this->max; 00038 if (data > this->max) data = this->min; 00039 } else { 00040 if (data < this->min) data = this->min; 00041 if (data > this->max) data = this->max; 00042 } 00043 return data; 00044 } 00045 00046 /** ---------- 00047 * @brief 値を更新する 00048 * @param data データ 00049 */ 00050 int KeyValueInt::set(int data){ 00051 this->value = range(data); 00052 return this->value; 00053 } 00054 00055 /** ---------- 00056 * @brief 値を増やす 00057 * @param delta 変化量(1) 00058 */ 00059 void KeyValueInt::inc(int delta){ 00060 set(range(value + delta)); 00061 } 00062 00063 /** ---------- 00064 * @brief 値を減らす 00065 * @param delta 変化量(1) 00066 */ 00067 void KeyValueInt::dec(int delta){ 00068 set(range(value - delta)); 00069 }
Generated on Tue Jul 12 2022 19:55:32 by
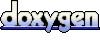