
System Management code
Dependencies: CANBuffer mbed SystemManagement mbed-rtos
SysMngmt.cpp
00001 /* 00002 Reads CAN Messages and various data inputs, outputs using Xbee radio modules 00003 00004 Revised Sept 30, 2014: Began analyzing and commenting program, trying to figure out what the hell it does (Martin Deng) 00005 */ 00006 00007 #include "SysMngmt.h" 00008 #include "Get_IMD.h" 00009 #include "PollSwitch.h" 00010 #include "TemperatureRead.h" 00011 #include "Store_RTC.h" 00012 #include "XBee_Lib.h" 00013 #include "CANBuffer.h" 00014 00015 #include "mbed.h" 00016 #include "rtos.h" 00017 00018 #include "Watchdog.cpp" 00019 #include "FanPump.h" 00020 #include "DC_DC.h" 00021 #include "PollSwitch.h" 00022 00023 //Possible problems in IMD coz change of counter 00024 //Possible problems in BatteryStatus coz change in library 00025 00026 /* 00027 00028 Attach Ticker every 10msec to 00029 Get IMD 00030 Poll Switches 00031 Temperature Read 00032 Get Battery State 00033 End Ticker Send message through CAN 00034 00035 CAN interrupt Rx Interrupt 00036 Recieve CAN message into a buffer. Return 00037 Buffer values(as long as !empty) -> SD Card, Xbee -> remove element 00038 00039 extern "C" void CAN_IRQHandler(void) 00040 { 00041 CANMessage Rxmsg; 00042 CAN_SysM.read(Rxmsg); 00043 RecieveBuffer.add(Rxmsg); 00044 } 00045 00046 http://developer.mbed.org/users/AjK/notebook/getting-closer-to-the-hardware/ 00047 00048 extern "C" means this is linked assuming it's C code 00049 C++ linker appearently adds extra crap with the function arguments keeping functions of this kind from linking properly 00050 00051 Interrupt handler, This is probably linked to the Timer 2 interrupt request somewhere by the libraries 00052 00053 extern "C" void TIMER2_IRQHandler(void) 00054 { 00055 if((LPC_TIM2->IR & 0x01) == 0x01) // if MR0 interrupt 00056 { 00057 // This probably shouldn't be here, never have a printf() in an interrupt, locks up the main thread 00058 // printf("Every 1ms\n\r"); 00059 00060 LPC_TIM2->IR |= 1 << 0; // Clear MR0 interrupt flag 00061 00062 // gonna hope all these calculations are correct 00063 // writes to RTC store, but there's no read code here 00064 // 2 misleading things. First, RTC store writes to a general purpose register 00065 // secondly, no code reads from this register in this .cpp, but maybe some other code does 00066 00067 Bat_I_Ratio=BatISense.read(); 00068 BATA_msec=(((Bat_I_Ratio*3.3) - BAT_ISENSE_OFFSET_V)/BAT_ISENSE_INCREMENT); 00069 BATmA_Hr+=(BATA_msec*MSEC_HRS); 00070 store.write(BATmA_Hr,0); 00071 00072 DC_I_Ratio=DCSense.read(); 00073 DCA_msec=(((DC_I_Ratio*3.3) - DC_DC_ISENSE_OFFSET_V)/DC_DC_ISENSE_INCREMENT); 00074 store.write(DCA_msec,1); 00075 00076 LPC_TIM2->TCR |= (1<<1); //Reset Timer1 00077 LPC_TIM2->TCR &= ~(1<<1); //Re Enable Timer1 00078 } 00079 } 00080 00081 Appears to read a whole bunch of DigitalOut pins in void PollSwitch(), store the value in uint16_t Rxpoll, 00082 and write the result to the CAN bus 00083 00084 void Poll() 00085 { 00086 uint16_t Rxpoll; 00087 uint16_t recv,temp,i=0; //Test 00088 char Result[4]={0}; 00089 Rxpoll=PollSwitch(); 00090 00091 Result[0]=(char)(Rxpoll&0x00ff); 00092 Result[1]=(char)((Rxpoll&0xff00)>>8); 00093 CANMessage Txmsg(410,Result,sizeof(Result)); 00094 CAN_SysM.write(Txmsg); 00095 00096 //Test 00097 recv=(((uint16_t)Txmsg.data[1]<<8) | (0x00ff&(uint16_t)Txmsg.data[0])); 00098 printf("Recv:%d\n\r",recv); 00099 00100 while(i <= 12) 00101 { 00102 temp=recv; 00103 if(((temp & (1 << i))>>i)==1) 00104 pc.printf("Switch OFF:%d\n\r",i); 00105 ++i; 00106 } 00107 } 00108 00109 00110 void Temp() 00111 { 00112 float DC_DC_Temperature, Coolant1_Temperature, Coolant2_Temperature, ChargerFET_Temperature; 00113 float Resistance; 00114 float Vadc; 00115 int i; 00116 ftc send, recv; 00117 recv.FLOAT=0.0; 00118 send.FLOAT=0.0; 00119 00120 Vadc=DC_DC.read()*VDD; 00121 Resistance=((float)R10K*Vadc)/((float)VDD + Vadc); 00122 DC_DC_Temperature=ReadTemp(TR_NXFT15XH103FA_Map, Resistance, TABLE_SIZE_NXFT15XH103FA); 00123 send.FLOAT=DC_DC_Temperature; 00124 CANMessage Txmsg_DC_DC(450,send.C_FLOAT,sizeof(send.C_FLOAT)); 00125 CAN_SysM.write(Txmsg_DC_DC); 00126 00127 for(i=0; i<4;i++) 00128 recv.C_FLOAT[i]=Txmsg_DC_DC.data[i]; 00129 pc.printf("DC_DC:%f\n\r",recv.FLOAT); 00130 00131 Vadc=ChargerFET.read()*VDD; 00132 Resistance=((float)R10K*Vadc)/((float)VDD + Vadc); 00133 ChargerFET_Temperature=ReadTemp(TR_NXFT15XH103FA_Map, Resistance, TABLE_SIZE_NXFT15XH103FA); 00134 send.FLOAT=ChargerFET_Temperature; 00135 CANMessage Txmsg_ChargerFET(451,send.C_FLOAT,sizeof(send.C_FLOAT)); 00136 CAN_SysM.write(Txmsg_ChargerFET); 00137 00138 for(i=0; i<4;i++) 00139 recv.C_FLOAT[i]=Txmsg_ChargerFET.data[i]; 00140 pc.printf("ChargerFET:%f\n\r",recv.FLOAT); 00141 00142 Vadc=Coolant1.read()*VDD; 00143 Resistance=((float)R10K*Vadc)/((float)VDD + Vadc); 00144 Coolant1_Temperature=ReadTemp(TR_NTCLP00E3103H_Map, Resistance, TABLE_SIZE_NTCLP00E3103H); 00145 send.FLOAT=Coolant1_Temperature; 00146 CANMessage Txmsg_Coolant1(452,send.C_FLOAT,sizeof(send.C_FLOAT)); 00147 CAN_SysM.write(Txmsg_Coolant1); 00148 //Control Fans 00149 00150 for(i=0; i<4;i++) 00151 recv.C_FLOAT[i]=Txmsg_Coolant1.data[i]; 00152 pc.printf("Coolant1:%f\n\r",recv.FLOAT); 00153 00154 Vadc=Coolant2.read()*VDD; 00155 Resistance=((float)R10K*Vadc)/((float)VDD + Vadc); 00156 Coolant2_Temperature=ReadTemp(TR_NTCLP00E3103H_Map, Resistance, TABLE_SIZE_NTCLP00E3103H); 00157 send.FLOAT=Coolant2_Temperature; 00158 CANMessage Txmsg_Coolant2(453,send.C_FLOAT,sizeof(send.C_FLOAT)); 00159 CAN_SysM.write(Txmsg_Coolant2); 00160 //Control Fans 00161 00162 for(i=0; i<4;i++) 00163 recv.C_FLOAT[i]=Txmsg_Coolant2.data[i]; 00164 pc.printf("Coolant2:%f\n\r",recv.FLOAT); 00165 } 00166 00167 void IMD() 00168 { 00169 IMD_Measurement_Output IMD_Signal; 00170 char status[4]; 00171 ftc send; 00172 00173 IMD_Signal=Get_Measurement(); 00174 send.FLOAT=IMD_Signal.Frequency; 00175 CANMessage Txmsg_Frequency(421,send.C_FLOAT,sizeof(send.C_FLOAT)); 00176 CAN_SysM.write(Txmsg_Frequency); 00177 00178 send.FLOAT=IMD_Signal.Duty_Cycle; 00179 CANMessage Txmsg_DutyCycle(422,send.C_FLOAT,sizeof(send.C_FLOAT)); 00180 CAN_SysM.write(Txmsg_DutyCycle); 00181 00182 status[0]=Result.Encoded_Status; 00183 CANMessage Txmsg_Status(423,status,sizeof(status)); 00184 CAN_SysM.write(Txmsg_Status); 00185 } 00186 */ 00187 00188 /* 00189 Activates a whole crapload of functions and pins on the chip 00190 00191 void Init() 00192 { 00193 00194 Timers to call various functions at different intervals 00195 These things behave weirdly when wait(ms) is involved. Probably have to rewrite 00196 00197 00198 //ReadIMD.attach(&IMD,0.1); 00199 //PollSDSwitch.attach(&Poll,0.1); 00200 00201 //ReadTemperature.attach(&Temp,0.1); 00202 //ReadBatteryState.attach(&Battery,0.1); 00203 00204 00205 Initialize Timer2 for Battery State 00206 00207 LPC_SC 0x400F C000 (System Control) 00208 ->PCONP 0x400F C0C4 (Power Control for Peripherals Register) 00209 |= (1<<22) 22 Bit (Timer 2 power/clock control bit) 00210 00211 ->PCLKSEL1 Peripheral Clock Selection register 1 (controls rate of clock signal supplied to peripheral) 00212 |= ((1<<12) | (1<<13)); 12:13 Bits (Peripheral Clock Selection for TIMER2) 00213 00214 LPC_TIM2 0x4009 0000 (Timer 2) 00215 ->TCR 0x4009 0004 (Timer Control Register) 00216 |= (1<<0); 0 Bit (Counter Enable) 00217 00218 ->MR0 0x4009 0018 (Match Register) 00219 00220 ->MCR 0x4009 0014 (Match Control Register) What to do when Match Register matches the Timer Counter 00221 |= (1<<0); 0 Bit (Interrupt on MR0, interrupt generated when MR0 matches the value in TC) 00222 00223 00224 LPC_SC->PCONP |= (1<<22); //PoewerOn Timer/Counter2 00225 LPC_SC->PCLKSEL1 |= ((1<<12) | (1<<13)); //Prescale Timer2 CCLK/8 00226 LPC_TIM2->TCR |= (1<<0); //Enable Timer2 00227 LPC_TIM2->MR0 = 11999; // 1msec 00228 LPC_TIM2->MCR |= (1<<0); 00229 00230 Nested Vectored Interrupt Controller (NVIC) 00231 00232 NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority) 00233 sets priority of an interrupt 00234 00235 IRQn_Type 00236 Interrupt number definitions 00237 Interrupt Request (IRQ) 00238 00239 NVIC_EnableIRQ(IRQn_Type IRQn) 00240 Enable external interrupt (in this case, the TIMER2_IRQHandler(void) function above gets called every time 00241 Timer2 generates an interrupt signal) 00242 00243 00244 NVIC_SetPriority(TIMER0_IRQn,200); //IMD Capture Interrupt 00245 NVIC_SetPriority(TIMER1_IRQn,200); //IMD 1msec sampling Interrupt 00246 NVIC_SetPriority(TIMER2_IRQn,1); //Battery 1msec sampling Interrupt 00247 NVIC_SetPriority(TIMER3_IRQn,255); //mbed Timer/Ticker/Wait Interrupt 00248 NVIC_SetPriority(CAN_IRQn,2); 00249 00250 NVIC_EnableIRQ(TIMER2_IRQn); //Enable TIMER2 IRQ 00251 00252 CAN_SysM.mode(CAN::GlobalTest); 00253 00254 00255 //NVIC_EnableIRQ(CAN_IRQn); 00256 //NVIC_EnableIRQ(CANActivity_IRQn); 00257 } 00258 00259 00260 Main Loop: Currently reads CANMessages from Can interface (Pins: rd = p30, td = p29) 00261 Send CANMessage data through XBee radio transmitters 00262 */ 00263 00264 00265 CANBuffer rxBuffer(CAN1, MEDIUM); 00266 XBee250x XbeeTx; 00267 Serial pc1(USBTX,USBRX); 00268 00269 char sys_src_id = 4; // source address of system management 00270 00271 int main() { 00272 CANMessage rx_msg; 00273 Watchdog wdt; 00274 00275 wdt.kick(10.0); 00276 pc1.baud(115200); 00277 00278 FanPump fanPump(&rxBuffer); 00279 DC dc_dc(&fanPump, &rxBuffer); 00280 PollSwitch pollSwitch(&rxBuffer); 00281 00282 fanPump.start_update(); 00283 dc_dc.start_update(); 00284 pollSwitch.start_update(); 00285 00286 while(1) 00287 { 00288 if(rxBuffer.rxRead(rx_msg)){ 00289 char src_addr = (rx_msg.id & 0x0700) >> 8; // get bits 10:8 00290 00291 if(src_addr == sys_src_id){ 00292 char cont_id = (rx_msg.id & 0x00FF); // get bits 7:0 00293 00294 // only control fans of dc_dc converter is on 00295 if(cont_id == RX_FAN_ID && dc_dc.is_on()) 00296 { 00297 fanPump.set_fan((FanSelect)rx_msg.data[0], rx_msg.data[1]); 00298 } 00299 00300 if(cont_id == RX_DC_DC_ID){ 00301 dc_dc.set(rx_msg.data[0]); 00302 } 00303 } // check for correct src_addr 00304 } // check CANBuffer 00305 00306 wdt.kick(); 00307 } // main while loop 00308 }
Generated on Sat Aug 13 2022 03:42:38 by
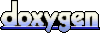