
This library is designed to create and run state graphs. It supports hierarchical states and the parallel execution
utt_foobar_with_ud.h
00001 #ifndef __UTT_FOOBAR_WUD_H__ 00002 #define __UTT_FOOBAR_WUD_H__ 00003 00004 #include "StateMachine.h" 00005 00006 class Foo : public State{ 00007 00008 public: 00009 00010 static const char* OUTCOME_BAR; 00011 00012 Foo(const char* uuid, UserData *ud): 00013 State(uuid), 00014 _count(0) 00015 { 00016 // Capture data ptr by key 00017 _data = ud->get<DataInt32*>("COUNT"); 00018 } 00019 00020 virtual void onEntry(){ /* Do something */ } 00021 00022 virtual const char* onExecute(){ 00023 00024 _count++; 00025 00026 return OUTCOME_BAR; 00027 } 00028 00029 virtual void onExit(){ 00030 // Update data 00031 _data->setData(_count); 00032 } 00033 00034 private: 00035 int _count; 00036 DataInt32 *_data; 00037 }; 00038 00039 const char* Foo::OUTCOME_BAR = "OUTCOME_BAR"; 00040 00041 class Bar : public State{ 00042 00043 public: 00044 00045 static const char* OUTCOME_FOO; 00046 00047 Bar(const char* uuid, UserData *ud): 00048 State(uuid) 00049 { 00050 // Capture data ptr 00051 _data = ud->get<DataInt32*>("COUNT"); 00052 } 00053 00054 virtual void onEntry(){ 00055 } 00056 00057 virtual const char* onExecute(){ 00058 00059 Logger::info("COUNT : %i",_data->getData()); 00060 00061 // Check data value 00062 if (_data->getData() >= 20){ 00063 return SUCCEDED; 00064 } 00065 00066 return OUTCOME_FOO; 00067 } 00068 00069 virtual void onExit(){ 00070 } 00071 00072 private: 00073 DataInt32 *_data; 00074 }; 00075 00076 const char* Bar::OUTCOME_FOO = "OUTCOME_FOO"; 00077 00078 class FooBar : public StateMachine{ 00079 00080 public: 00081 00082 FooBar(const char* uuid, UserData *ud): 00083 StateMachine(uuid, ud), 00084 foo(NULL), bar(NULL) 00085 { 00086 // States instance 00087 foo = this->Instance<Foo>("FOO"); 00088 bar = this->Instance<Bar>("BAR"); 00089 00090 // Connect Foo to Bar 00091 this->connect(STATE(foo), Foo::OUTCOME_BAR, STATE(bar)); 00092 00093 // Connect Bar to Foo 00094 this->connect(STATE(bar), Bar::OUTCOME_FOO, STATE(foo)); 00095 00096 // Connect Bar to exit state machine 00097 this->connect(STATE(bar), Bar::SUCCEDED, SUCCEDED); 00098 00099 this->setInitialState(STATE(foo)); 00100 } 00101 00102 private: 00103 Foo *foo; 00104 Bar *bar; 00105 }; 00106 00107 /* 00108 InterruptIn button(PC_13); 00109 DigitalOut led(LED1); 00110 */ 00111 void unit_test(){ 00112 00113 StateMachine root("FOOBAR_ROOT"); 00114 00115 button.rise(&root, &FooBar::preempt); 00116 00117 DataInt32* data = new DataInt32(0); 00118 00119 root.getUserData()->put("COUNT", data); 00120 00121 FooBar *foobar1 = root.Instance<FooBar>("FOOBAR_1"); 00122 FooBar *foobar2 = root.Instance<FooBar>("FOOBAR_2"); 00123 00124 root.setInitialState(STATE(foobar1)); 00125 00126 root.connect(STATE(foobar1), "SUCCEDED", STATE(foobar2)); 00127 root.connect(STATE(foobar2), "SUCCEDED", "SUCCEDED"); 00128 00129 root.printGraph(); 00130 00131 printf("STATE MACHINE RETURN %s \n",root.execute()); 00132 } 00133 00134 00135 #endif /* #ifndef __UTT_FOOBAR_WUD_H__*/
Generated on Fri Jul 15 2022 16:02:41 by
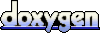